forked from science-ation/science-ation
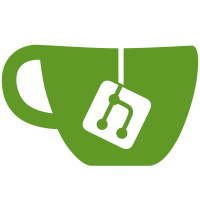
Update invitation system to keep track of the number that have been invited and not allow the school to invite more than they are allowed Update the schools editor, to allow specifying the max number of projects and whether the max is total for the school, or per age category Add required fields to the schools table Update the config variable for special awards registration to allow turning special awards registration off
200 lines
8.6 KiB
PHP
200 lines
8.6 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require("../common.inc.php");
|
|
auth_required('admin');
|
|
|
|
send_header("Administration - Schools");
|
|
?>
|
|
|
|
<?
|
|
echo "<a href=\"index.php\"><< ".i18n("Back to Administration")."</a>\n";
|
|
|
|
if($_POST['save']=="edit" || $_POST['save']=="add")
|
|
{
|
|
if($_POST['save']=="add")
|
|
{
|
|
$q=mysql_query("INSERT INTO schools (year) VALUES ('".$config['FAIRYEAR']."')");
|
|
$id=mysql_insert_id();
|
|
}
|
|
else
|
|
$id=$_POST['id'];
|
|
|
|
|
|
$exec="UPDATE schools SET ".
|
|
"school='".mysql_escape_string(stripslashes($_POST['school']))."', ".
|
|
"address='".mysql_escape_string(stripslashes($_POST['address']))."', ".
|
|
"city='".mysql_escape_string(stripslashes($_POST['city']))."', ".
|
|
"province_code='".mysql_escape_string(stripslashes($_POST['province_code']))."', ".
|
|
"postalcode='".mysql_escape_string(stripslashes($_POST['postalcode']))."', ".
|
|
"phone='".mysql_escape_string(stripslashes($_POST['phone']))."', ".
|
|
"fax='".mysql_escape_string(stripslashes($_POST['fax']))."', ".
|
|
"sciencehead='".mysql_escape_string(stripslashes($_POST['sciencehead']))."', ".
|
|
"scienceheadphone='".mysql_escape_string(stripslashes($_POST['scienceheadphone']))."', ".
|
|
"scienceheademail='".mysql_escape_string(stripslashes($_POST['scienceheademail']))."', ".
|
|
"registration_password='".mysql_escape_string(stripslashes($_POST['registration_password']))."', ".
|
|
"projectlimit='".mysql_escape_string(stripslashes($_POST['projectlimit']))."', ".
|
|
"projectlimitper='".mysql_escape_string(stripslashes($_POST['projectlimitper']))."', ".
|
|
"accesscode='".mysql_escape_string(stripslashes($_POST['accesscode']))."' ".
|
|
"WHERE id='$id'";
|
|
mysql_query($exec);
|
|
echo mysql_error();
|
|
|
|
if($_POST['save']=="add")
|
|
echo happy("School successfully added");
|
|
else
|
|
echo happy("Successfully saved changes to school");
|
|
}
|
|
|
|
if($_GET['action']=="delete" && $_GET['delete'])
|
|
{
|
|
mysql_query("DELETE FROM schools WHERE id='".$_GET['delete']."'");
|
|
echo happy("School successfully deleted");
|
|
}
|
|
|
|
if($_GET['action']=="edit" || $_GET['action']=="add")
|
|
{
|
|
|
|
echo "<a href=\"schools.php\"><< ".i18n("Back to Schools")."</a>\n";
|
|
if($_GET['action']=="edit")
|
|
{
|
|
echo "<h3>".i18n("Edit School")."</h3>\n";
|
|
$buttontext="Save School";
|
|
$q=mysql_query("SELECT * FROM schools WHERE id='".$_GET['edit']."'");
|
|
$r=mysql_fetch_object($q);
|
|
}
|
|
else if($_GET['action']=="add")
|
|
{
|
|
echo "<h3>".i18n("Add New School")."</h3>\n";
|
|
$buttontext="Add School";
|
|
}
|
|
$buttontext=i18n($buttontext);
|
|
|
|
echo "<form method=\"post\" action=\"schools.php\">\n";
|
|
echo "<input type=\"hidden\" name=\"save\" value=\"".$_GET['action']."\">\n";
|
|
|
|
if($_GET['action']=="edit")
|
|
echo "<input type=\"hidden\" name=\"id\" value=\"".$_GET['edit']."\">\n";
|
|
|
|
echo "<table>\n";
|
|
echo "<tr><td>".i18n("School Name")."</td><td><input type=\"text\" name=\"school\" value=\"".htmlspecialchars($r->school)."\" size=\"60\" maxlength=\"128\" /></td></tr>\n";
|
|
echo "<tr><td>".i18n("Address")."</td><td><input type=\"text\" name=\"address\" value=\"".htmlspecialchars($r->address)."\" size=\"60\" maxlength=\"64\" /></td></tr>\n";
|
|
echo "<tr><td>".i18n("City")."</td><td><input type=\"text\" name=\"city\" value=\"".htmlspecialchars($r->city)."\" size=\"32\" maxlength=\"32\" /></td></tr>\n";
|
|
echo "<tr><td>".i18n("Province")."</td><td>";
|
|
emit_province_selector("province_code",$r->province_code);
|
|
echo "</td></tr>\n";
|
|
echo "<tr><td>".i18n("Postal Code")."</td><td><input type=\"text\" name=\"postalcode\" value=\"$r->postalcode\" size=\"8\" maxlength=\"7\" /></td></tr>\n";
|
|
echo "<tr><td>".i18n("Phone")."</td><td><input type=\"text\" name=\"phone\" value=\"".htmlspecialchars($r->phone)."\" size=\"16\" maxlength=\"16\" /></td></tr>\n";
|
|
echo "<tr><td>".i18n("Fax")."</td><td><input type=\"text\" name=\"fax\" value=\"".htmlspecialchars($r->fax)."\" size=\"16\" maxlength=\"16\" /></td></tr>\n";
|
|
echo "<tr><td>".i18n("Access Code")."</td><td><input type=\"text\" name=\"accesscode\" value=\"".htmlspecialchars($r->accesscode)."\" size=\"32\" maxlength=\"32\" /></td></tr>\n";
|
|
echo "<tr><td colspan=2><br /><b>".i18n("Science head/teacher or science fair contact at school")."</b></td></tr>";
|
|
echo "<tr><td>".i18n("Name")."</td><td><input type=\"text\" name=\"sciencehead\" value=\"".htmlspecialchars($r->sciencehead)."\" size=\"60\" maxlength=\"64\" /></td></tr>\n";
|
|
echo "<tr><td>".i18n("Phone")."</td><td><input type=\"text\" name=\"scienceheadphone\" value=\"".htmlspecialchars($r->scienceheadphone)."\" size=\"16\" maxlength=\"16\" /></td></tr>\n";
|
|
echo "<tr><td>".i18n("Email")."</td><td><input type=\"text\" name=\"scienceheademail\" value=\"".htmlspecialchars($r->scienceheademail)."\" size=\"60\" maxlength=\"128\" /></td></tr>\n";
|
|
|
|
if($config['participant_registration_type']=="schoolpassword")
|
|
{
|
|
echo "<tr><td colspan=2><br /><b>".i18n("Participant Registration Password")."</b></td></tr>";
|
|
echo "<tr><td>".i18n("Password")."</td><td><input type=\"text\" name=\"registration_password\" value=\"".htmlspecialchars($r->registration_password)."\" size=\"32\" maxlength=\"32\" /></td></tr>\n";
|
|
}
|
|
echo "<tr><td colspan=2><br /><b>".i18n("Participant Registration Limits")."</b></td></tr>";
|
|
if($config['participant_registration_type']=="invite")
|
|
{
|
|
echo "<tr><td colspan=2>".i18n("Set to 0 to have no registration limit")."</td></tr>";
|
|
echo "<tr><td colspan=2>".i18n("Maximum of")." ";
|
|
echo "<input type=\"text\" name=\"projectlimit\" value=\"".htmlspecialchars($r->projectlimit)."\" size=\"4\" maxlength=\"4\" />";
|
|
echo " ";
|
|
echo i18n("projects");
|
|
echo " ";
|
|
echo "<select name=\"projectlimitper\">";
|
|
if($r->projectlimitper=="total") $sel="selected=\"selected\""; else $sel="";
|
|
echo "<option $sel value=\"total\">".i18n("total")."</option>\n";
|
|
if($r->projectlimitper=="agecategory") $sel="selected=\"selected\""; else $sel="";
|
|
echo "<option $sel value=\"agecategory\">".i18n("per age category")."</option>\n";
|
|
echo "</select>";
|
|
echo "</td></tr>\n";
|
|
}
|
|
else
|
|
{
|
|
echo "<tr><td colspan=2>".i18n("Participant registration limits are currently disabled. In order to use participant registration limits for schools, the participant registration type must be set to 'invite' in Configuration / Configuration Variables")."</td></tr>";
|
|
|
|
|
|
}
|
|
echo "<tr><td colspan=\"2\"> </td></tr>";
|
|
echo "<tr><td colspan=\"2\" align=\"center\"><input type=\"submit\" value=\"$buttontext\" /></td></tr>\n";
|
|
|
|
echo "</table>\n";
|
|
echo "</form>\n";
|
|
|
|
|
|
|
|
}
|
|
else
|
|
{
|
|
|
|
|
|
echo "<br />";
|
|
echo "<a href=\"schools.php?action=add\">Add New School</a>\n";
|
|
echo "<br />";
|
|
echo "<table class=\"summarytable\">";
|
|
echo "<tr>";
|
|
echo " <th>School</th>";
|
|
echo " <th>Address</th>";
|
|
echo " <th>Phone</th>";
|
|
echo " <th>Contact</th>";
|
|
if($config['participant_registration_type']=="schoolpassword")
|
|
echo " <th>Reg Pass</th>";
|
|
echo " <th>Action</th>";
|
|
echo "</tr>\n";
|
|
|
|
$q=mysql_query("SELECT * FROM schools WHERE year='".$config['FAIRYEAR']."' ORDER BY school");
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
echo "<tr>\n";
|
|
echo " <td>$r->school</td>\n";
|
|
echo " <td>$r->address, $r->city, $r->postalcode</td>\n";
|
|
echo " <td>$r->phone</td>\n";
|
|
echo " <td>$r->sciencehead</td>\n";
|
|
if($config['participant_registration_type']=="schoolpassword")
|
|
echo " <td>$r->registration_password</td>\n";
|
|
|
|
echo " <td align=\"center\">";
|
|
echo "<a href=\"schools.php?action=edit&edit=$r->id\"><img border=\"0\" src=\"".$config['SFIABDIRECTORY']."/images/16/edit.".$config['icon_extension']."\"></a>";
|
|
echo " ";
|
|
echo "<a onclick=\"return confirmClick('Are you sure you want to remove this school?')\" href=\"schools.php?action=delete&delete=$r->id\"><img border=0 src=\"".$config['SFIABDIRECTORY']."/images/16/button_cancel.".$config['icon_extension']."\"></a>";
|
|
|
|
|
|
echo " </td>\n";
|
|
echo "</tr>\n";
|
|
}
|
|
|
|
echo "</table>\n";
|
|
|
|
|
|
}
|
|
|
|
send_footer();
|
|
|
|
?>
|