forked from science-ation/science-ation
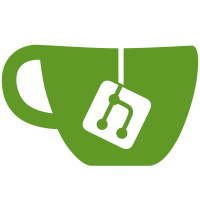
will be used for viewing information. also switch the judge info button to just say the name when a person is selected
320 lines
9.3 KiB
PHP
320 lines
9.3 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require("../common.inc.php");
|
|
auth_required('admin');
|
|
|
|
send_header("Administration - Judging Teams");
|
|
?>
|
|
<script language="javascript" type="text/javascript">
|
|
function addbuttonclicked(team)
|
|
{
|
|
document.forms.judges.action.value="add";
|
|
document.forms.judges.team_num.value=team;
|
|
document.forms.judges.submit();
|
|
}
|
|
function delbuttonclicked(team_id,team_num,team_name,judge)
|
|
{
|
|
document.forms.judges.action.value="del";
|
|
document.forms.judges.team_id.value=team_id;
|
|
document.forms.judges.team_num.value=team_num;
|
|
document.forms.judges.team_name.value=team_name;
|
|
document.forms.judges.judges_id.value=judge;
|
|
document.forms.judges.submit();
|
|
}
|
|
|
|
function saveteamnamesbuttonclicked()
|
|
{
|
|
document.forms.judges.action.value="saveteamnames";
|
|
document.forms.judges.submit();
|
|
}
|
|
|
|
function openjudgeinfo(id)
|
|
{
|
|
if(id)
|
|
currentid=id;
|
|
else
|
|
currentid=document.forms.judges["judgelist[]"].options[document.forms.judges["judgelist[]"].selectedIndex].value;
|
|
|
|
window.open("judges_info.php?id="+currentid,"JudgeInfo","location=no,menubar=no,directories=no,toolbar=no,width=600,height=400");
|
|
return false;
|
|
|
|
}
|
|
function switchjudgeinfo()
|
|
{
|
|
if(document.forms.judges["judgelist[]"].selectedIndex != -1)
|
|
{
|
|
currentname=document.forms.judges["judgelist[]"].options[document.forms.judges["judgelist[]"].selectedIndex].text;
|
|
currentid=document.forms.judges["judgelist[]"].options[document.forms.judges["judgelist[]"].selectedIndex].value;
|
|
|
|
document.forms.judges.judgeinfobutton.disabled=false;
|
|
document.forms.judges.judgeinfobutton.value=currentname;
|
|
|
|
}
|
|
else
|
|
{
|
|
document.forms.judges.judgeinfobutton.disabled=true;
|
|
document.forms.judges.judgeinfobutton.value="<? echo i18n("Judge Info")?>";
|
|
}
|
|
|
|
}
|
|
|
|
</script>
|
|
|
|
<?
|
|
echo "<a href=\"index.php\"><< ".i18n("Back to Administration")."</a>\n";
|
|
echo "<a href=\"judges.php\"><< ".i18n("Back to Judges")."</a>\n";
|
|
|
|
if($_POST['action']=="add" && $_POST['team_num'] && count($_POST['judgelist'])>0)
|
|
{
|
|
//first check if this team exists.
|
|
$q=mysql_query("SELECT id,name FROM judges_teams WHERE num='".$_POST['team_num']."' AND year='".$config['FAIRYEAR']."'");
|
|
if(mysql_num_rows($q))
|
|
{
|
|
$r=mysql_fetch_object($q);
|
|
$team_id=$r->id;
|
|
$team_name=$r->name;
|
|
}
|
|
else
|
|
{
|
|
echo notice(i18n("Creating new team #%1 with name '%2'",array($_POST['team_num'],$_POST['new_team_name'])));
|
|
mysql_query("INSERT INTO judges_teams (num,name,year) VALUES ('".$_POST['team_num']."','".$_POST['new_team_name']."','".$config['FAIRYEAR']."')");
|
|
$team_id=mysql_insert_id();
|
|
$team_name=$_POST['new_team_name'];
|
|
}
|
|
|
|
$added=0;
|
|
|
|
foreach($_POST['judgelist'] AS $selectedjudge)
|
|
{
|
|
mysql_query("INSERT INTO judges_teams_link (judges_id,judges_teams_id,year) VALUES ('$selectedjudge','$team_id','".$config['FAIRYEAR']."')");
|
|
$added++;
|
|
|
|
}
|
|
if($added==1) $j=i18n("judge");
|
|
else $j=i18n("judges");
|
|
|
|
echo happy(i18n("%1 %2 added to team #%3 (%4)",array($added,$j,$_POST['team_num'],$team_name)));
|
|
}
|
|
|
|
if($_POST['action']=="del" && $_POST['team_num'] && $_POST['team_id'] && $_POST['judges_id'])
|
|
{
|
|
mysql_query("DELETE FROM judges_teams_link WHERE judges_id='".$_POST['judges_id']."' AND judges_teams_id='".$_POST['team_id']."' AND year='".$config['FAIRYEAR']."'");
|
|
echo happy(i18n("Removed judge from team #%1 (%2)",array($_POST['team_num'],$_POST['team_name'])));
|
|
|
|
$q=mysql_query("SELECT * FROM judges_teams_link WHERE judges_teams_id='".$_POST['team_id']."' AND year='".$config['FAIRYEAR']."'");
|
|
if(mysql_num_rows($q)==0)
|
|
{
|
|
mysql_query("DELETE FROM judges_teams WHERE year='".$config['FAIRYEAR']."' AND id='".$_POST['team_id']."'");
|
|
echo notice(i18n("Removed empty team #%1 (%2)",array($_POST['team_num'],$_POST['team_name'])));
|
|
}
|
|
|
|
|
|
|
|
}
|
|
|
|
if($_POST['action']=="saveteamnames")
|
|
{
|
|
if(count($_POST['team_names']))
|
|
{
|
|
foreach($_POST['team_names'] AS $team_id=>$team_name)
|
|
{
|
|
mysql_query("UPDATE judges_teams SET name='".mysql_escape_string(stripslashes($team_name))."' WHERE id='$team_id'");
|
|
}
|
|
echo happy(i18n("Team names successfully saved"));
|
|
}
|
|
|
|
}
|
|
|
|
echo "<form name=\"judges\" method=\"post\" action=\"judges_teams.php\">";
|
|
echo "<input type=\"hidden\" name=\"action\">";
|
|
echo "<input type=\"hidden\" name=\"team_id\">";
|
|
echo "<input type=\"hidden\" name=\"team_num\">";
|
|
echo "<input type=\"hidden\" name=\"team_name\">";
|
|
echo "<input type=\"hidden\" name=\"judges_id\">";
|
|
echo "<table>";
|
|
echo "<tr>";
|
|
echo "<th>".i18n("Judges List");
|
|
echo "<br />";
|
|
echo "<input disabled=\"true\" name=\"judgeinfobutton\" id=\"judgeinfobutton\" onclick=\"openjudgeinfo()\" type=\"button\" value=\"".i18n("Judge Info")."\">";
|
|
echo "</th>";
|
|
echo "<th>".i18n("Judge Teams")."</th>";
|
|
echo "</tr>";
|
|
echo "<tr><td valign=\"top\">";
|
|
|
|
|
|
/*
|
|
//mysql 4.0 does not support subqueries - it is supported as of mysql 4.1
|
|
//this means we cant use NOT IN (SELECT..) so, we will have to workaround this
|
|
//at least for now.
|
|
|
|
$querystr="SELECT
|
|
judges.id,
|
|
judges.firstname,
|
|
judges.lastname
|
|
FROM
|
|
judges,
|
|
judges_years
|
|
WHERE
|
|
judges_years.year='".$config['FAIRYEAR']."' AND
|
|
judges.id=judges_years.judges_id AND
|
|
judges.id NOT IN (SELECT judges_id AS id FROM judges_teams_link WHERE judges_teams_link.year='".$config['FAIRYEAR']."')
|
|
ORDER BY
|
|
lastname,
|
|
firstname";
|
|
*/
|
|
$querystr="SELECT
|
|
judges.id,
|
|
judges.firstname,
|
|
judges.lastname,
|
|
judges_teams_link.judges_id
|
|
FROM
|
|
judges
|
|
LEFT JOIN judges_teams_link ON judges.id = judges_teams_link.judges_id,
|
|
judges_years
|
|
WHERE
|
|
judges_years.year='".$config['FAIRYEAR']."' AND
|
|
judges.id=judges_years.judges_id AND
|
|
judges_teams_link.judges_id IS NULL AND
|
|
judges.complete='yes'
|
|
ORDER BY
|
|
lastname,
|
|
firstname";
|
|
|
|
$q=mysql_query($querystr);
|
|
|
|
echo mysql_error();
|
|
echo "<select name=\"judgelist[]\" onchange=\"switchjudgeinfo()\" multiple=\"multiple\" style=\"width: 250px; height: 600px;\">";
|
|
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
if($r->firstname && $r->lastname)
|
|
echo "<option value=\"$r->id\">$r->firstname $r->lastname</option>\n";
|
|
}
|
|
|
|
echo "</select>";
|
|
echo "</td>";
|
|
echo "<td valign=\"top\">";
|
|
|
|
$q=mysql_query("SELECT judges_teams.id,
|
|
judges_teams.num,
|
|
judges_teams.name,
|
|
judges.id AS judges_id,
|
|
judges.firstname,
|
|
judges.lastname
|
|
|
|
FROM
|
|
judges,
|
|
judges_teams,
|
|
judges_teams_link
|
|
WHERE
|
|
judges_teams.year='".$config['FAIRYEAR']."' AND
|
|
judges_teams_link.judges_id=judges.id AND
|
|
judges_teams_link.judges_teams_id=judges_teams.id
|
|
ORDER BY
|
|
name,
|
|
num,
|
|
lastname,
|
|
firstname");
|
|
|
|
$lastteamid=-1;
|
|
$lastteamnum=-1;
|
|
echo mysql_error();
|
|
$teams=array();
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
if($r->id!=$lastteamid)
|
|
{
|
|
$teams[$r->id]['id']=$r->id;
|
|
$teams[$r->id]['num']=$r->num;
|
|
$teams[$r->id]['name']=$r->name;
|
|
$lastteamid=$r->id;
|
|
$lastteamnum=$r->num;
|
|
}
|
|
$teams[$lastteamid]['members'][]=array("id"=>$r->judges_id,"firstname"=>$r->firstname,"lastname"=>$r->lastname);
|
|
}
|
|
//echo nl2br(print_r($teams,true));
|
|
|
|
if($lastteamnum==-1) $newteamid=1;
|
|
else $newteamid=$lastteamnum+1;
|
|
|
|
|
|
echo "<table>";
|
|
echo "<tr><td valign=top>";
|
|
echo "<input onclick=\"addbuttonclicked('$newteamid')\" type=\"button\" value=\"Add >>\">";
|
|
echo "</td><td>";
|
|
|
|
echo "<table>";
|
|
echo "<tr><th align=\"left\">New #$newteamid: <input type=\"text\" name=\"new_team_name\" value=\"Team #$newteamid\" /></th></tr>";
|
|
echo "</table>";
|
|
|
|
echo "</td></tr></table>";
|
|
|
|
|
|
|
|
foreach($teams AS $team)
|
|
{
|
|
echo "<hr>";
|
|
|
|
echo "<table>";
|
|
echo "<tr><td valign=top>";
|
|
echo "<input onclick=\"addbuttonclicked('".$team['num']."')\" type=\"button\" value=\"Add >>\">";
|
|
echo "</td><td>";
|
|
|
|
echo "<table>\n";
|
|
echo "<tr><th colspan=\"2\" align=\"left\">#".$team['num'].": ";
|
|
echo "<input type=\"text\" name=\"team_names[".$team['id']."]\" value=\"".$team['name']."\" size=\"10\">";
|
|
echo "</th></tr>\n";
|
|
|
|
foreach($team['members'] AS $member)
|
|
{
|
|
echo "<tr><td>";
|
|
echo "<input onclick=\"delbuttonclicked('".$team['id']."','".$team['num']."','".htmlspecialchars($team['name'])."','".$member['id']."')\" type=\"button\" value=\"<<\">";
|
|
echo "</td><td>";
|
|
echo "<a href=\"\" onclick=\"return openjudgeinfo(".$member['id'].");\">";
|
|
echo $member['firstname']." ".$member['lastname'];
|
|
echo "</a>";
|
|
echo "</td></tr>";
|
|
}
|
|
|
|
echo "</table>";
|
|
|
|
echo "</td></tr></table>";
|
|
}
|
|
|
|
echo "<br />";
|
|
echo "<input type=\"button\" onclick=\"saveteamnamesbuttonclicked()\" type=\"button\" value=\"Save Team Names\" />";
|
|
|
|
|
|
|
|
echo "</td></tr>";
|
|
echo "</table>";
|
|
echo "</form>";
|
|
|
|
send_footer();
|
|
|
|
|
|
|
|
?>
|