forked from science-ation/science-ation
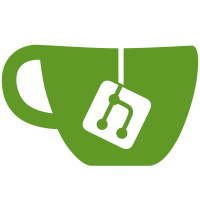
checks for maximum number of nominations allow logins of users when registration is closed
240 lines
8.4 KiB
PHP
240 lines
8.4 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require("common.inc.php");
|
|
|
|
$q=mysql_query("SELECT (NOW()>'".$config['dates']['regopen']."' AND NOW()<'".$config['dates']['regclose']."') AS datecheck");
|
|
$datecheck=mysql_fetch_object($q);
|
|
|
|
if($_POST['action']=="new")
|
|
{
|
|
$q=mysql_query("SELECT email,num,id FROM registrations WHERE email='".$_SESSION['email']."' AND num='".$_POST['regnum']."' AND year=".$config['FAIRYEAR']);
|
|
if(mysql_num_rows($q))
|
|
{
|
|
$r=mysql_fetch_object($q);
|
|
$_SESSION['registration_number']=$r->num;
|
|
$_SESSION['registration_id']=$r->id;
|
|
mysql_query("INSERT INTO students (registrations_id,email,year) VALUES ('$r->id','".mysql_escape_string($_SESSION['email'])."','".$config['FAIRYEAR']."')");
|
|
mysql_query("UPDATE registrations SET status='open' WHERE id='$r->id'");
|
|
|
|
header("Location: register_participants_main.php");
|
|
exit;
|
|
|
|
}
|
|
else
|
|
{
|
|
send_header("Participant Registration");
|
|
echo error(i18n("Invalid registration number (%1) for email address %2",array($_POST['regnum'],$_SESSION['email'])));
|
|
$_POST['action']="login";
|
|
}
|
|
|
|
}
|
|
else if($_POST['action']=="continue")
|
|
{
|
|
|
|
$q=mysql_query("SELECT registrations.id AS regid, registrations.num AS regnum, students.id AS studentid, students.firstname FROM registrations,students ".
|
|
"WHERE students.email='".$_SESSION['email']."' ".
|
|
"AND registrations.num='".$_POST['regnum']."' ".
|
|
"AND students.registrations_id=registrations.id ".
|
|
"AND registrations.year=".$config['FAIRYEAR']." ".
|
|
"AND students.year=".$config['FAIRYEAR']);
|
|
|
|
if(mysql_num_rows($q))
|
|
{
|
|
$r=mysql_fetch_object($q);
|
|
$_SESSION['registration_number']=$r->regnum;
|
|
$_SESSION['registration_id']=$r->regid;
|
|
header("Location: register_participants_main.php");
|
|
exit;
|
|
}
|
|
else
|
|
{
|
|
send_header("Participant Registration");
|
|
echo error(i18n("Invalid registration number (%1) for email address %2",array($_POST['regnum'],$_SESSION['email'])));
|
|
$_POST['action']="login";
|
|
}
|
|
|
|
}
|
|
else if($_GET['action']=="resend" && $_SESSION['email'])
|
|
{
|
|
$q=mysql_query("SELECT registrations.num FROM registrations, students WHERE students.email='".$_SESSION['email']."' AND students.registrations_id=registrations.id");
|
|
$r=mysql_fetch_object($q);
|
|
|
|
email_send("register_participants_resend_regnum",$_SESSION['email'],array("FAIRNAME"=>i18n($config['fairname'])),array("REGNUM"=>$r->num));
|
|
|
|
send_header("Participant Registration");
|
|
echo notice(i18n("Your registration number has been resent to your email addess <b>%1</b>",array($_SESSION['email'])));
|
|
}
|
|
else if($_GET['action']=="logout")
|
|
{
|
|
unset($_SESSION['email']);
|
|
unset($_SESSION['registration_number']);
|
|
unset($_SESSION['registration_id']);
|
|
send_header("Participant Registration");
|
|
echo notice(i18n("You have been successfully logged out"));
|
|
}
|
|
|
|
|
|
//if they've alreayd logged in, and somehow wound back up here, take them back to where they should be
|
|
if($_SESSION['registration_number'] && $_SESSION['registration_id'] && $_SESSION['email'])
|
|
{
|
|
header("Location: register_participants_main.php");
|
|
|
|
}
|
|
|
|
send_header("Participant Registration");
|
|
|
|
if($_POST['action']=="login" && ( $_POST['email'] || $_SESSION['email']) )
|
|
{
|
|
if($_POST['email'])
|
|
$_SESSION['email']=$_POST['email'];
|
|
|
|
echo "<form method=\"post\" action=\"register_participants.php\">";
|
|
|
|
$allownew=true;
|
|
//first, check if they have any registrations waiting to be opened
|
|
$q=mysql_query("SELECT * FROM registrations WHERE email='".$_SESSION['email']."' AND status='new' AND year=".$config['FAIRYEAR']);
|
|
if(mysql_num_rows($q)>0)
|
|
{
|
|
echo i18n("Please enter your <b>registration number</b> that you received in your email, in order to begin your new registration");
|
|
echo "<input type=\"hidden\" name=\"action\" value=\"new\">";
|
|
$allownew=false;
|
|
}
|
|
else
|
|
{
|
|
$q=mysql_query("SELECT students.email,
|
|
registrations.status
|
|
FROM students,
|
|
registrations
|
|
WHERE
|
|
students.email='".$_SESSION['email']."'
|
|
AND students.year=".$config['FAIRYEAR']."
|
|
AND registrations.year=".$config['FAIRYEAR']."
|
|
AND registrations.status='open'");
|
|
if(mysql_num_rows($q)>0)
|
|
{
|
|
echo i18n("Please enter your <b>registration number</b> in order to continue your registration");
|
|
echo "<input type=\"hidden\" name=\"action\" value=\"continue\">";
|
|
$allownew=false;
|
|
echo "<br />";
|
|
}
|
|
}
|
|
$showform=true;
|
|
|
|
if($allownew)
|
|
{
|
|
if($datecheck->datecheck==0)
|
|
{
|
|
echo error(i18n("Registration is closed. You can not create a new account"));
|
|
$showform=false;
|
|
echo "<A href=\"register_participants.php\">Back to Participant Registration Login Page</a>";
|
|
|
|
}
|
|
else
|
|
{
|
|
$regnum=0;
|
|
//now create the new registration record, and assign a random/unique registration number to then.
|
|
do
|
|
{
|
|
//random number between
|
|
//100000 and 999999 (six digit integer)
|
|
$regnum=rand(100000,999999);
|
|
$q=mysql_query("SELECT * FROM registrations WHERE num='$regnum' AND year=".$config['FAIRYEAR']);
|
|
}while(mysql_num_rows($q)>0);
|
|
|
|
//actually insert it
|
|
mysql_query("INSERT INTO registrations (num,email,start,status,year) VALUES (".
|
|
"'$regnum',".
|
|
"'".$_SESSION['email']."',".
|
|
"NOW(),".
|
|
"'new',".
|
|
$config['FAIRYEAR'].
|
|
")");
|
|
|
|
|
|
$mailbody= "A new registration account has been created for you.\n".
|
|
"To access your registration account, please enter\n".
|
|
"enter the following registration number into the\n".
|
|
"registration website:\n".
|
|
"\n".
|
|
"Registration Number: $regnum\n".
|
|
"\n";
|
|
mail($_SESSION['email'],i18n("Registration for %1",array(i18n($config['fairname']))),$mailbody);
|
|
|
|
echo i18n("You have been identified as a new registrant. An email has been sent to <b>%1</b> which contains your new <b>registration number</b>. Please check your email to obtain your <b>registration number</b> and then enter it below:",array($_SESSION['email']));
|
|
echo "<input type=\"hidden\" name=\"action\" value=\"new\">";
|
|
}
|
|
|
|
}
|
|
if($showform)
|
|
{
|
|
echo "<br />";
|
|
echo i18n("Registration Number:");
|
|
echo "<input type=\"text\" size=\"10\" name=\"regnum\">";
|
|
echo "<input type=\"submit\" value=\"Submit\">";
|
|
echo "</form>";
|
|
echo "<br />";
|
|
echo i18n("If you have lost or forgotten your <b>registration number</b>, please <a href=\"register_participants.php?action=resend\">click here to resend</a> it to your email address");
|
|
}
|
|
}
|
|
else
|
|
{
|
|
//Lets check the date - if we are AFTER 'regopen' and BEFORE 'regclose' then we can login
|
|
//otherwise, registration is closed - no logins!
|
|
|
|
//this will return 1 if its between the dates, 0 otherwise.
|
|
if($datecheck->datecheck==0)
|
|
{
|
|
echo notice(i18n("Registration for the %1 %2 is now closed. Existing registrants can login and view (read only) their information, as well as apply for special awards (if applicable).",array($config['FAIRYEAR'],$config['fairname'])));
|
|
echo i18n("Please enter your email address to login");
|
|
echo "<br />";
|
|
echo "<br />";
|
|
$buttontext=i18n("Login");
|
|
}
|
|
else
|
|
{
|
|
|
|
echo i18n("Please enter your email address to :");
|
|
echo "<ul>";
|
|
echo "<li>".i18n("Begin a new registration")."</li>";
|
|
echo "<li>".i18n("Continue a previously started registration")."</li>";
|
|
echo "<li>".i18n("Modify an existing registration")."</li>";
|
|
echo "</ul>";
|
|
echo i18n("You must enter a valid email address. We will be emailing you information which you will need to complete the registration process!");
|
|
echo "<br />";
|
|
echo "<br />";
|
|
$buttontext=i18n("Begin");
|
|
}
|
|
|
|
?>
|
|
<form method="post" action="register_participants.php">
|
|
<input type="hidden" name="action" value="login" />
|
|
<?=i18n("Email")?>: <input type="text" name="email" size="30" />
|
|
<input type="submit" value="<?=$buttontext?>" />
|
|
</form>
|
|
<?
|
|
}
|
|
send_footer();
|
|
?>
|