forked from science-ation/science-ation
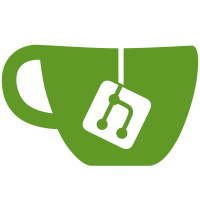
Also, update the CSS to make teh h1-h4's smaller and reduce the margin-bottom size for them
167 lines
3.9 KiB
PHP
167 lines
3.9 KiB
PHP
<?
|
|
function registrationFormsReceived($reg_id="")
|
|
{
|
|
if($reg_id) $rid=$reg_id;
|
|
else $rid=$_SESSION['registration_id'];
|
|
$q=mysql_query("SELECT status FROM registrations WHERE id='$rid'");
|
|
$r=mysql_fetch_object($q);
|
|
if($r->status=="complete" || $r->status=="paymentpending")
|
|
return true;
|
|
else
|
|
return false;
|
|
|
|
}
|
|
|
|
function studentStatus($reg_id="")
|
|
{
|
|
global $config;
|
|
$required_fields=array("firstname","lastname","address","city","postalcode","phone","email","grade","dateofbirth","schools_id","tshirt");
|
|
|
|
if($reg_id) $rid=$reg_id;
|
|
else $rid=$_SESSION['registration_id'];
|
|
|
|
$q=mysql_query("SELECT * FROM students WHERE registrations_id='$rid' AND year='".$config['FAIRYEAR']."'");
|
|
|
|
//if we dont have the minimum, return incomplete
|
|
if(mysql_num_rows($q)<$config['minstudentsperproject'])
|
|
return "incomplete";
|
|
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
foreach ($required_fields AS $req)
|
|
{
|
|
if(!$r->$req)
|
|
{
|
|
return "incomplete";
|
|
}
|
|
}
|
|
}
|
|
|
|
//if it made it through without returning incomplete, then we must be complete
|
|
return "complete";
|
|
}
|
|
|
|
function emergencycontactStatus($reg_id="")
|
|
{
|
|
global $config;
|
|
$required_fields=array("firstname","lastname","relation","phone1");
|
|
|
|
if($reg_id) $rid=$reg_id;
|
|
else $rid=$_SESSION['registration_id'];
|
|
|
|
$sq=mysql_query("SELECT id FROM students WHERE registrations_id='$rid' AND year='".$config['FAIRYEAR']."'");
|
|
$numstudents=mysql_num_rows($sq);
|
|
|
|
while($sr=mysql_fetch_object($sq))
|
|
{
|
|
$q=mysql_query("SELECT * FROM emergencycontact WHERE registrations_id='$rid' AND year='".$config['FAIRYEAR']."' AND students_id='$sr->id'");
|
|
|
|
$r=mysql_fetch_object($q);
|
|
|
|
foreach ($required_fields AS $req)
|
|
{
|
|
if(!$r->$req)
|
|
{
|
|
return "incomplete";
|
|
}
|
|
}
|
|
}
|
|
|
|
//if it made it through without returning incomplete, then we must be complete
|
|
return "complete";
|
|
}
|
|
|
|
function projectStatus($reg_id="")
|
|
{
|
|
global $config;
|
|
$required_fields=array("title","projectcategories_id","projectdivisions_id","summary","language","req_table","req_electricity");
|
|
|
|
if($reg_id) $rid=$reg_id;
|
|
else $rid=$_SESSION['registration_id'];
|
|
|
|
$q=mysql_query("SELECT * FROM projects WHERE registrations_id='$rid' AND year='".$config['FAIRYEAR']."'");
|
|
|
|
//if we dont have a project entry yet, return empty
|
|
if(!mysql_num_rows($q))
|
|
return "empty";
|
|
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
foreach ($required_fields AS $req)
|
|
{
|
|
if(!$r->$req)
|
|
{
|
|
return "incomplete";
|
|
}
|
|
}
|
|
}
|
|
|
|
//if it made it through without returning incomplete, then we must be complete
|
|
return "complete";
|
|
}
|
|
|
|
|
|
function mentorStatus($reg_id="")
|
|
{
|
|
global $config;
|
|
$required_fields=array("firstname","lastname","phone","email","organization","description");
|
|
|
|
if($reg_id) $rid=$reg_id;
|
|
else $rid=$_SESSION['registration_id'];
|
|
|
|
//first check the registrations table to see if 'nummentors' is set, or if its null
|
|
$q=mysql_query("SELECT nummentors FROM registrations WHERE id='$rid' AND year='".$config['FAIRYEAR']."'");
|
|
$r=mysql_fetch_object($q);
|
|
if($r->nummentors==null)
|
|
return "incomplete";
|
|
|
|
$q=mysql_query("SELECT * FROM mentors WHERE registrations_id='$rid' AND year='".$config['FAIRYEAR']."'");
|
|
|
|
//if we dont have the minimum, return incomplete
|
|
if(mysql_num_rows($q)<$config['minmentorserproject'])
|
|
return "incomplete";
|
|
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
foreach ($required_fields AS $req)
|
|
{
|
|
if(!$r->$req)
|
|
{
|
|
return "incomplete";
|
|
}
|
|
}
|
|
}
|
|
|
|
//if it made it through without returning incomplete, then we must be complete
|
|
return "complete";
|
|
|
|
}
|
|
|
|
function safetyStatus($reg_id="")
|
|
{
|
|
if($reg_id) $rid=$reg_id;
|
|
else $rid=$_SESSION['registration_id'];
|
|
|
|
//grab all of their answers
|
|
$q=mysql_query("SELECT * FROM safety WHERE registrations_id='$rid'");
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
$safetyanswers[$r->safetyquestions_id]=$r->answer;
|
|
}
|
|
|
|
//now grab all the questions
|
|
$q=mysql_query("SELECT * FROM safetyquestions ORDER BY ord");
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
if($r->required=="yes" && !$safetyanswers[$r->id])
|
|
{
|
|
return "incomplete";
|
|
}
|
|
}
|
|
return "complete";
|
|
|
|
}
|
|
|
|
|
|
?>
|