forked from science-ation/science-ation
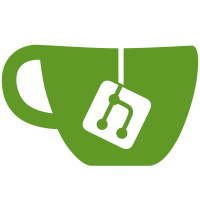
Fix the registration_list.php to work again - temporarily disable "create new project" and the ability to ADD students to an existing project
913 lines
32 KiB
PHP
913 lines
32 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
// legacy - functions previously included here have been split off into participants.inc.php,
|
|
// but expected in this file by other code
|
|
require_once("participant.inc.php");
|
|
require_once("projects.inc.php");
|
|
|
|
function generateProjectNumber($registration_id)
|
|
{
|
|
global $config, $conference;
|
|
|
|
$reg_id = $registration_id;
|
|
|
|
$q=mysql_query("SELECT projects.projectcategories_id,
|
|
projects.projectdivisions_id,
|
|
projectcategories.category_shortform,
|
|
projectdivisions.division_shortform
|
|
FROM
|
|
projects,
|
|
projectcategories,
|
|
projectdivisions
|
|
WHERE
|
|
registrations_id='$reg_id'
|
|
AND projects.projectdivisions_id=projectdivisions.id
|
|
AND projects.projectcategories_id=projectcategories.id
|
|
AND projectcategories.conferences_id='{$conference['id']}'
|
|
AND projectdivisions.conferences_id='{$conference['id']}'
|
|
");
|
|
echo mysql_error();
|
|
$r=mysql_fetch_object($q);
|
|
|
|
$p=array('number'=>array(), 'sort'=>array() );
|
|
$p['number']['str'] = $config['project_num_format'];
|
|
$p['sort']['str'] = trim($config['project_sort_format']);
|
|
|
|
if($p['sort']['str'] == '') $p['sort']['str'] = $p['number']['str'];
|
|
|
|
/* Replace each letter with {letter}, so that we can do additional
|
|
* replacements below, without risking subsituting in a letter that may
|
|
* get replaced. */
|
|
foreach(array('number','sort') as $x) {
|
|
$p[$x]['str']=ereg_replace('[CcDd]', '{\\0}', $p[$x]['str']);
|
|
$p[$x]['str']=ereg_replace('(N|X)([0-9])?', '{\\0}', $p[$x]['str']);
|
|
}
|
|
|
|
/* Do some replacements that we don' thave to do anything fancy with,
|
|
* and setup some variables for future queries */
|
|
foreach(array('number','sort') as $x) {
|
|
$p[$x]['str']=str_replace('{D}',$r->projectdivisions_id,$p[$x]['str']);
|
|
$p[$x]['str']=str_replace('{C}',$r->projectcategories_id,$p[$x]['str']);
|
|
$p[$x]['str']=str_replace('{d}',$r->division_shortform,$p[$x]['str']);
|
|
$p[$x]['str']=str_replace('{c}',$r->category_shortform,$p[$x]['str']);
|
|
$p[$x]['n_used'] = array();
|
|
$p[$x]['x_used'] = array();
|
|
}
|
|
|
|
/* Build a total list of projects for finding a global number, and
|
|
* while constructing the list, build a list for the division/cat
|
|
* sequence number */
|
|
$q = mysql_query("SELECT projectnumber_seq,projectsort_seq,
|
|
projectdivisions_id,projectcategories_id
|
|
FROM projects
|
|
WHERE conferences_id='{$conference['id']}'
|
|
AND projectnumber_seq!='0'
|
|
AND projectnumber IS NOT NULL");
|
|
echo mysql_error();
|
|
while($i = mysql_fetch_object($q)) {
|
|
if( ($r->projectdivisions_id == $i->projectdivisions_id)
|
|
&&($r->projectcategories_id == $i->projectcategories_id) ) {
|
|
$p['number']['n_used'][] = $i->projectnumber_seq;
|
|
$p['sort']['n_used'][] = $i->projectsort_seq;
|
|
}
|
|
|
|
$p['number']['x_used'][] = $i->projectnumber_seq;
|
|
$p['sort']['x_used'][] = $i->projectsort_seq;
|
|
}
|
|
|
|
/* We only support one N or X to keep things simple, find which
|
|
* one we need and how much to pad it */
|
|
foreach(array('number','sort') as $x) {
|
|
if(ereg("(N|X)([0-9])?", $p[$x]['str'], $regs)) {
|
|
$p[$x]['seq_type'] = $regs[1];
|
|
if($regs[2] != '')
|
|
$p[$x]['seq_pad'] = $regs[2];
|
|
else
|
|
$p[$x]['seq_pad'] = ($regs[1] == 'N') ? 2 : 3;
|
|
|
|
if($regs[1] == 'N')
|
|
$p[$x]['used'] = $p[$x]['n_used'];
|
|
else
|
|
$p[$x]['used'] = $p[$x]['x_used'];
|
|
} else {
|
|
/* FIXME: maybe we should error here? Not having an N
|
|
* or an X in the projectnumber or projectsort is a bad
|
|
* thing */
|
|
$p[$x]['seq_type'] = '';
|
|
$p[$x]['seq_pad'] = 0;
|
|
$p[$x]['used'] = array();
|
|
}
|
|
}
|
|
|
|
/* Find the lowest unused number. FIXME: this could be a config
|
|
* option, we could search for the lowest unused number (if projects
|
|
* get deleted), or we could just go +1 beyond the highest */
|
|
foreach(array('number','sort') as $x) {
|
|
if($p[$x]['seq_type'] == '') continue;
|
|
$n = 0;
|
|
while(1) {
|
|
$n++;
|
|
if(in_array($n, $p[$x]['used'])) continue;
|
|
|
|
$r = sprintf("%'0{$p[$x]['seq_pad']}d", $n);
|
|
$str = ereg_replace("{(N|X)([0-9])?}", $r, $p[$x]['str']);
|
|
$p[$x]['str'] = $str;
|
|
$p[$x]['n'] = $n;
|
|
break;
|
|
}
|
|
|
|
/* If we're using the same number type for sorting, then we, in
|
|
* theory, know what that number is, so we can go ahead and
|
|
* blindly use it */
|
|
if($p['number']['seq_type'] == $p['sort']['seq_type']) {
|
|
$r = sprintf("%'0{$p['sort']['seq_pad']}d", $n);
|
|
$p['sort']['str'] = ereg_replace("{(N|X)([0-9])?}", $r, $p['sort']['str']);
|
|
$p['sort']['n'] = $n;
|
|
break;
|
|
}
|
|
}
|
|
|
|
return array($p['number']['str'], $p['sort']['str'],
|
|
$p['number']['n'], $p['sort']['n']);
|
|
}
|
|
|
|
function computeRegistrationFee($regid)
|
|
{
|
|
global $config, $conference;
|
|
$ret = array();
|
|
|
|
$regfee_items = array();
|
|
$q = mysql_query("SELECT * FROM regfee_items
|
|
WHERE conferences_id='{$conference['id']}'");
|
|
while($i = mysql_fetch_assoc($q)) $regfee_items[] = $i;
|
|
|
|
$q=mysql_query("SELECT * FROM students WHERE registrations_id='$regid' AND conferences_id='".$conference['id']."'");
|
|
$n_students = mysql_num_rows($q);
|
|
$n_tshirts = 0;
|
|
$sel = array();
|
|
while($s = mysql_fetch_object($q)) {
|
|
if($s->tshirt != 'none') $n_tshirts++;
|
|
|
|
/* Check their regfee items too */
|
|
if($config['participant_regfee_items_enable'] != 'yes') continue;
|
|
|
|
$sel_q = mysql_query("SELECT * FROM regfee_items_link
|
|
WHERE students_id={$s->id}");
|
|
while($info_q = mysql_fetch_assoc($sel_q)) {
|
|
$sel[] = $info_q['regfee_items_id'];
|
|
}
|
|
}
|
|
|
|
if($config['regfee_per'] == 'student') {
|
|
$f = $config['regfee'] * $n_students;
|
|
$ret[] = array( 'id' => 'regfee',
|
|
'text' => "Fair Registration (per student)",
|
|
'base' => $config['regfee'],
|
|
'num' => $n_students,
|
|
'ext' => $f );
|
|
$regfee += $f;
|
|
} else {
|
|
$ret[] = array( 'id' => 'regfee',
|
|
'text' => "Fair Registration (per project)",
|
|
'base' => $config['regfee'],
|
|
'num' => 1,
|
|
'ext' => $config['regfee'] );
|
|
$regfee += $config['regfee'];
|
|
}
|
|
|
|
if($config['participant_student_tshirt'] == 'yes') {
|
|
$tsc = floatval($config['participant_student_tshirt_cost']);
|
|
if($tsc != 0.0) {
|
|
$f = $n_tshirts * $tsc;
|
|
$regfee += $f;
|
|
if($n_tshirts != 0) {
|
|
$ret[] = array( 'id' => 'tshirt',
|
|
'text' => "T-Shirts",
|
|
'base' => $tsc,
|
|
'num' => $n_tshirts,
|
|
'ext' => $f);
|
|
}
|
|
}
|
|
}
|
|
|
|
/* $sel will be empty if regfee_items is disabled */
|
|
foreach($regfee_items as $rfi) {
|
|
$cnt = 0;
|
|
foreach($sel as $s) if($rfi['id'] == $s) $cnt++;
|
|
|
|
if($cnt == 0) continue;
|
|
|
|
$tsc = floatval($rfi['cost']);
|
|
|
|
/* If it's per project, force the count to 1 */
|
|
if($rfi['per'] == 'project') {
|
|
$cnt = 1;
|
|
}
|
|
|
|
$f = $tsc * $cnt;
|
|
$ret[] = array( 'id' => "regfee_item_{$rfi['id']}",
|
|
'text' => "{$rfi['name']} (per {$rfi['per']})" ,
|
|
'base' => $tsc,
|
|
'num' => $cnt,
|
|
'ext' => $f);
|
|
$regfee += $f;
|
|
}
|
|
return array($regfee, $ret);
|
|
}
|
|
|
|
/******************************************************************************
|
|
New functionality split off for API purposes
|
|
******************************************************************************/
|
|
|
|
/** Hmm - perhaps these sholud be split into separate files ...
|
|
This section is for project/registration related functions **/
|
|
|
|
function saveProjectData($data,$registrations_id=null){
|
|
global $conference, $config;
|
|
|
|
//if we have it passed in, then use it, otherwise, use the session
|
|
if($registrations_id)
|
|
$rid=$registrations_id;
|
|
else
|
|
$rid=$_SESSION['registration_id'];
|
|
|
|
//inconsistency here, we give the objecet to them with an "id" but we expect a "project_id" back
|
|
if($data['id'] && !$data['project_id']) $data['project_id']=$data['id'];
|
|
|
|
$requiredFields = array('project_id', 'title', 'projectdivisions_id', 'language');
|
|
if($config['participant_short_title_enable'] == 'yes')
|
|
$requiredFields[] = 'shorttitle';
|
|
if($config['participant_project_summary_wordmin'] > 0)
|
|
$required_fields[] = 'summary';
|
|
if($config['participant_project_table'] == 'yes')
|
|
$requiredFields[] = 'req_table';
|
|
if($config['participant_project_electricity'] == 'yes')
|
|
$requiredFields[] = 'req_electricity';
|
|
|
|
$missingFields = array();
|
|
foreach($requiredFields as $field){
|
|
if(!array_key_exists($field, $data)){
|
|
$missingFields[] = $field;
|
|
}else if(trim($data[$field]) == ''){
|
|
$missingFields[] = $field;
|
|
}
|
|
}
|
|
|
|
if(registrationFormsReceived()){
|
|
$message = i18n("Cannot make changes to forms once they have been received by the fair");
|
|
}else if(registrationDeadlinePassed()){
|
|
$message = i18n("Cannot make changes to forms after registration deadline");
|
|
/* if fields are missing, thtas okay, save what we do have, no point makign them re-enter the whole thing
|
|
}else if(count($missingFields)){
|
|
$message = i18n("Required fields missing: %1", array(implode(', ', $missingFields)));
|
|
*/
|
|
}else{
|
|
//first, lets make sure this project really does belong to them
|
|
$qstr="SELECT * FROM projects WHERE id='" . $data['project_id'] . "' AND registrations_id='" . $rid . "' AND conferences_id='" . $conference['id'] . "'";
|
|
$q = mysql_query($qstr);
|
|
if(mysql_num_rows($q) == 1) {
|
|
$summarywords = preg_split("/[\s,]+/", $data['summary']);
|
|
$summarywordcount = count($summarywords);
|
|
if($summarywordcount > $config['participant_project_summary_wordmax'] || $summarywordcount<$config['participant_project_summary_wordmin'])
|
|
$summarycountok=0;
|
|
else
|
|
$summarycountok=1;
|
|
|
|
if($config['participant_project_title_charmax'] && strlen($data['title'])>$config['participant_project_title_charmax']) //0 for no limit, eg 255 database field limit
|
|
{
|
|
$title=substr($data['title'],0,$config['participant_project_title_charmax']);
|
|
$message = i18n("Project title truncated to %1 characters",array($config['participant_project_title_charmax']));
|
|
}
|
|
else
|
|
$title=$data['title'];
|
|
|
|
if($config['participant_short_title_enable'] == 'yes'
|
|
&& $config['participant_short_title_charmax']
|
|
&& strlen($data['shorttitle'])>$config['participant_short_title_charmax']) //0 for no limit, eg 255 database field limit
|
|
{
|
|
$shorttitle=substr($data['shorttitle'],0,$config['participant_short_title_charmax']);
|
|
$message = i18n("Short project title truncated to %1 characters",array($config['participant_short_title_charmax']));
|
|
}
|
|
else
|
|
$shorttitle=$data['shorttitle'];
|
|
|
|
mysql_query("UPDATE projects SET " .
|
|
"title='" . mysql_real_escape_string($title)."', " .
|
|
"shorttitle='" . mysql_real_escape_string($shorttitle) . "', " .
|
|
"projectdivisions_id='" . $data['projectdivisions_id'] . "', " .
|
|
"language='" . mysql_real_escape_string($data['language']) . "', " .
|
|
"req_table='" . mysql_real_escape_string($data['req_table']) . "', " .
|
|
"req_electricity='" . mysql_real_escape_string($data['req_electricity']) . "', " .
|
|
"req_special='" . mysql_real_escape_string($data['req_special']) . "', " .
|
|
"summary='" . mysql_real_escape_string($data['summary']) . "', " .
|
|
"summarycountok='$summarycountok'" .
|
|
"WHERE id='" . $data['project_id'] . "'");
|
|
$message = mysql_error();
|
|
|
|
//update the safetyquestion answers (safety table)
|
|
if(is_array($data['safetyquestions'])) {
|
|
//wipe them all out first
|
|
mysql_query("DELETE FROM safety WHERE registrations_id='{$rid}' AND conferences_id='{$conference['id']}'");
|
|
//and add them back
|
|
foreach($data['safetyquestions'] AS $q) {
|
|
if($q['id']) {
|
|
mysql_query("INSERT INTO safety (registrations_id,safetyquestions_id,answer,conferences_id) VALUES (
|
|
'{$rid}',
|
|
'{$q['id']}',
|
|
'".mysql_real_escape_string($q['answer'])."',
|
|
'{$conference['id']}')");
|
|
}
|
|
}
|
|
}
|
|
|
|
//and update the special award nominations
|
|
//but make sure they dont sign up for anything they're not allowed to :p
|
|
if(is_array($data['specialawards'])) {
|
|
$eligibleAwards = getSpecialAwardsEligibleForProject($data['project_id']);
|
|
$eligibleAwardsList=array();
|
|
foreach($eligibleAwards AS $ea) {
|
|
$eligibleAwardsList[]=$ea['id'];
|
|
}
|
|
mysql_query("DELETE FROM project_specialawards_link WHERE projects_id='{$data['project_id']}' AND conferences_id='{$conference['id']}'");
|
|
|
|
//now filter the list we've been given, to make sure everything's hunky dory
|
|
$awards=array();
|
|
foreach($data['specialawards'] AS $sa) {
|
|
//If all they've selected is they don't want to self nominate, then erase all other selections
|
|
if($sa['id']==-1 && $sa['selected']) {
|
|
//reset the array
|
|
unset($awards);
|
|
$awards=array();
|
|
$awards[]=$sa;
|
|
//and stop looping
|
|
break;
|
|
}
|
|
else {
|
|
if($sa['selected'] && in_array($sa['id'],$eligibleAwardsList)) {
|
|
echo "id {$sa['id']} is selected AND eligible\n";
|
|
$awards[]=$sa;
|
|
}
|
|
}
|
|
}
|
|
$idx=0;
|
|
foreach($awards AS $sa) {
|
|
$qstr="INSERT INTO project_specialawards_link (award_awards_id,projects_id,conferences_id) VALUES ('".intval($sa['id'])."','".intval($data['project_id'])."','{$conference['id']}')";
|
|
mysql_query($qstr);
|
|
$idx++;
|
|
//dont let them sign up for more than they are allowed to
|
|
if($idx>=$config['maxspecialawardsperproject'])
|
|
break;
|
|
}
|
|
}
|
|
|
|
//if the status is 'new', change it to 'open' but we dont change any other statuses to 'open'
|
|
//becuase if tis already complete, or paymentpending, we dont want to reset that
|
|
//though, they shouldnt be submitting this is its complete or paymentpending, EXCEPT
|
|
//if they are saving the special award nominations.... crap... ummmm
|
|
//FIXME: read above comment, and figure out a solution
|
|
mysql_query("UPDATE registrations SET status='open' WHERE status='new' AND id='{$rid}'");
|
|
|
|
//and update nummentors in registrations, yea, i know its not in the projects table
|
|
if(isset($data['nummentors'])) {
|
|
if($data['nummentors']==null) {
|
|
mysql_query("UPDATE registrations SET nummentors=NULL WHERE id='{$rid}'");
|
|
}
|
|
else {
|
|
mysql_query("UPDATE registrations SET nummentors='".intval($data['nummentors'])."' WHERE id='{$rid}'");
|
|
}
|
|
}
|
|
|
|
//and update numstudents in registrations, yea, i know its not in the projects table
|
|
if(isset($data['numstudents'])) {
|
|
if($data['numstudents']==null) {
|
|
mysql_query("UPDATE registrations SET numstudents=NULL WHERE id='{$rid}'");
|
|
}
|
|
else {
|
|
mysql_query("UPDATE registrations SET numstudents='".intval($data['numstudents'])."' WHERE id='{$rid}'");
|
|
}
|
|
}
|
|
|
|
if($message == ''){
|
|
$message = 'success';
|
|
}
|
|
}
|
|
else {
|
|
$message = i18n("Invalid project to update: %1",array($qstr));
|
|
}
|
|
}
|
|
return $message;
|
|
}
|
|
|
|
//get a random registration number between 100000 and 999999 (six digit integer)
|
|
// that isn't already in use
|
|
//TODO - set up a method of ensuring that the very unlikely chance of two people simultaneously
|
|
// getting the same number is in fact impossible
|
|
function getNewRegNum(){
|
|
global $conference;
|
|
do {
|
|
$regnum = rand(100000,999999);
|
|
$q = mysql_query("SELECT * FROM registrations WHERE num='$regnum' AND conferences_id=".$conference['id']);
|
|
}while(mysql_num_rows($q) > 0);
|
|
return $regnum;
|
|
}
|
|
|
|
// add a registration record and return it's unique id and registration number in an array
|
|
// returns an error message if the user is alredy registered
|
|
function addRegistration($userId){
|
|
global $conference;
|
|
|
|
// first we make sure that they don't already have a project on the go
|
|
$query = mysql_query("SELECT * FROM users WHERE id = $userId");
|
|
$err = mysql_error();
|
|
if($err){
|
|
return "register_participants.inc.php::addRegistration -> " . $err;
|
|
}
|
|
|
|
$row = mysql_fetch_assoc($query);
|
|
if(!$row){
|
|
return "register_participants.inc.php::addRegistration -> user not found";
|
|
}
|
|
|
|
if($row['registrations_id'] != null){
|
|
return "register_participants.inc.php::addRegistration -> user already has a project registered";
|
|
}
|
|
|
|
// create the new registration record, and assign a random/unique registration number.
|
|
$regnum = getNewRegNum();
|
|
|
|
//actually insert it
|
|
$query = "INSERT INTO registrations (num,email,start,status,conferences_id) VALUES (".
|
|
"'$regnum', ".
|
|
"'".$_SESSION['email']."', ".
|
|
"NOW(), ".
|
|
"'new', ".
|
|
$conference['id'].
|
|
")";
|
|
mysql_query($query);
|
|
$err = mysql_error();
|
|
if($err){
|
|
return "register_participants.inc.php::addRegistration -> " . $err;
|
|
}else{
|
|
$regid = mysql_insert_id();
|
|
}
|
|
|
|
// update the user now, connecting them to that registration
|
|
$query = "UPDATE users SET registrations_id = $regid WHERE id = $userId";
|
|
mysql_query($query);
|
|
$err = mysql_error();
|
|
if($err){
|
|
return "register_participants.inc.php::addRegistration -> " . $err;
|
|
}
|
|
|
|
// ok, if the flow hits this point, then we've successfully added the registration and
|
|
// linked the user to it. Return the registration number
|
|
return array('registrations_id' => $regid, 'registration_number' => $regnum);
|
|
}
|
|
|
|
// get the registration id for a specific user.
|
|
//Accepts either the user object or the user id, and returns the registration id, or NULL if none is set, error message if something's borked
|
|
function getRegistrationsId($user){
|
|
// get the user id
|
|
if(is_array($user)){
|
|
if(!array_key_exists('id', $user)) return "register_participants.inc.php::getRegistrationsId -> Invalid user object";
|
|
$uid = $user['id'];
|
|
}else{
|
|
$uid = $user;
|
|
}
|
|
|
|
// grab their registrations id
|
|
$result = mysql_fetch_assoc(mysql_query("SELECT registrations_id FROM users WHERE id = $uid"));
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::getRegistrationsId -> " . mysql_error();
|
|
}
|
|
|
|
return $result['registrations_id'];
|
|
}
|
|
function getRegistration($id) {
|
|
global $conference;
|
|
$q=mysql_query("SELECT id,id AS registration_id,num,num AS registration_number,start,status AS registration_status,end,nummentors,numstudents FROM registrations WHERE id='$id' AND conferences_id='{$conference['id']}'");
|
|
$reg=mysql_fetch_assoc($q);
|
|
if(!$reg) return null;
|
|
|
|
//get the students
|
|
$s=mysql_query("SELECT users.id,users.firstname,users.lastname, accounts.id AS accounts_id, accounts.username FROM users JOIN accounts ON users.accounts_id=accounts.id WHERE users.registrations_id='$id' AND users.conferences_id='{$conference['id']}'");
|
|
while($r=mysql_fetch_assoc($s)) {
|
|
//asdf
|
|
$r['status']=studentIndividualStatus($r['id']);
|
|
$reg['students'][]=$r;
|
|
}
|
|
|
|
//and also get the project
|
|
$pq=mysql_query("SELECT id,projectnumber,title FROM projects WHERE registrations_id='$id' AND conferences_id='{$conference['id']}'");
|
|
$p=mysql_fetch_assoc($pq);
|
|
|
|
//move nummentors from registration object into the project object
|
|
//we'll also load it on getProejct, and save it on saveProjectData
|
|
//even though its in the registrations table not the projects table
|
|
$p['nummentors']=$reg['nummentors'];
|
|
$p['numstudents']=$reg['numstudents'];
|
|
unset($reg['nummentors']);
|
|
unset($reg['numstudents']);
|
|
|
|
$reg['project']=$p;
|
|
|
|
//and finally, the status breakdown for the entire registration
|
|
$reg['status']=participant_status_completion_details(null,$id);
|
|
return $reg;
|
|
}
|
|
|
|
function saveRegistrationData($d) {
|
|
global $conference;
|
|
/*
|
|
if($d['id']) {
|
|
mysql_query("UPDATE registrations SET nummentors='{$d['nummentors']}' WHERE id='{$d['id']}' AND conferences_id='{$conference['id']}'");
|
|
return getRegistration($d['id']);
|
|
}
|
|
else
|
|
return null;
|
|
*/
|
|
return getRegistration($data['id']);
|
|
}
|
|
|
|
function getProject($userId,$registrations_id=null){
|
|
global $conference;
|
|
|
|
if($registrations_id) {
|
|
$regId=$registrations_id;
|
|
}
|
|
else {
|
|
$userId = intval($userId);
|
|
$result = mysql_fetch_assoc(mysql_query("SELECT registrations_id FROM users WHERE id='$userId'"));
|
|
if(!is_array($result)){
|
|
return "register_participants.inc.php::getProject -> User not found";
|
|
}
|
|
$regId = $result['registrations_id'];
|
|
if(!$regId) {
|
|
return "register_participants.inc.php::getProject -> no registration id";
|
|
}
|
|
}
|
|
|
|
// FIXME - in the future, this should be able to handle a many-to-many
|
|
// relationship in projects to registrations (so remove that LIMIT 1)
|
|
$fields = implode(',', array(
|
|
'projects.id',
|
|
'projects.id AS project_id',
|
|
'registrations.num AS registration_number',
|
|
'projects.projectdivisions_id',
|
|
'projects.title',
|
|
'projects.language',
|
|
'projects.req_electricity',
|
|
'projects.registrations_id',
|
|
'projects.req_table',
|
|
'projects.req_special',
|
|
'projects.summary'
|
|
));
|
|
$q = mysql_query("SELECT $fields FROM projects
|
|
JOIN registrations ON registrations.id = projects.registrations_id
|
|
WHERE projects.registrations_id='$regId'
|
|
AND projects.conferences_id='".$conference['id']."'
|
|
LIMIT 1");
|
|
if(mysql_error()) {
|
|
return "register_participants.inc.php::getProject -> sql error: " . $mysql_error();
|
|
} else if(mysql_num_rows($q)!=1) {
|
|
return "register_participants.inc.php::getProject -> no project";
|
|
}
|
|
else {
|
|
$returnval = mysql_fetch_assoc($q);
|
|
}
|
|
|
|
$q=mysql_query("SELECT nummentors,numstudents FROM registrations WHERE id='$regId'");
|
|
$r=mysql_fetch_assoc($q);
|
|
$returnval['nummentors']=$r['nummentors'];
|
|
$returnval['numstudents']=$r['numstudents'];
|
|
|
|
$safetyquestions=getSafetyQuestions($regId);
|
|
$returnval['safetyquestions']=$safetyquestions;
|
|
|
|
/*
|
|
$noawards=getNominatedForNoSpecialAwardsForProject($returnval['id']);
|
|
$returnval['specialawards_none']=$noawards;
|
|
*/
|
|
|
|
$specialawards=getAwardListing($returnval['id']);
|
|
$returnval['specialawards']=$specialawards;
|
|
return $returnval;
|
|
}
|
|
|
|
// add a project
|
|
function addProject($registrations_id){
|
|
global $conference;
|
|
// get the appropriate category for this project
|
|
$projCategory = getProjectCategory($registrations_id);
|
|
mysql_query("
|
|
INSERT INTO projects(registrations_id, conferences_id, projectcategories_id)
|
|
VALUES ('" . $registrations_id . "','" . $conference['id']."', '$projCategory')
|
|
");
|
|
//now query the one we just inserted
|
|
$returnval=getProject(null,$registrations_id);
|
|
return $returnval;
|
|
}
|
|
|
|
// join an existing project
|
|
// perhaps a bit of a misnomer as it's actually the registration that's being joined, but meh.
|
|
// return 'ok' on success, error message on failure
|
|
function joinProject($registration_number, $email){
|
|
global $config;
|
|
global $conference;
|
|
|
|
$uid = $_SESSION['users_id'];
|
|
if(getRegistrationsId($uid) !== null){
|
|
return 'register_participants.inc.php::joinProject -> you are already registered for a project';
|
|
}
|
|
|
|
// let's avoid any SQL naughtiness
|
|
$email = mysql_real_escape_string($email);
|
|
$registration_number = intval($registration_number);
|
|
|
|
$query = mysql_query("SELECT id FROM registrations WHERE num = '$registration_number' AND conferences_id='{$conference['id']}'");
|
|
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::joinProject -> " . mysql_error();
|
|
}
|
|
$result = mysql_fetch_assoc($query);
|
|
if(!$result){
|
|
return "register_participants.inc.php::joinProject -> invalid registration number";
|
|
}
|
|
$registration_id = $result['id'];
|
|
//okay the registration number matches, but now does it match the user/account tied to it?
|
|
|
|
$q=mysql_query("SELECT users.id,accounts.id,accounts.email,accounts.pendingemail
|
|
FROM users
|
|
JOIN accounts ON users.accounts_id=accounts.id
|
|
WHERE
|
|
users.registrations_id='$registration_id'
|
|
AND ( accounts.username='$email'
|
|
OR accounts.email='$email'
|
|
OR accounts.pendingemail='$email'
|
|
)");
|
|
|
|
if(!$r=mysql_fetch_object($q)) {
|
|
return "register_participants.inc.php::joinProject -> email address doesnt match registration";
|
|
}
|
|
|
|
|
|
// let's see if this project already has the maximum number of students on it
|
|
$ucount = mysql_fetch_array(mysql_query("SELECT COUNT(*) FROM users WHERE registrations_id = $registration_id"));
|
|
if($ucount[0] >= $config['maxstudentsperproject']){
|
|
return "register_participants.inc.php::joinProject -> the specified project already has the maxiumum number of students assigned to it";
|
|
}
|
|
|
|
// ok, if we've made it this far, they've correctly added the info that we verify with. Go ahead
|
|
// and add them to the registration
|
|
mysql_query("UPDATE users SET registrations_id = $registration_id WHERE id = $uid");
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::joinProject -> " . mysql_error();
|
|
}
|
|
|
|
// finally, we can update the project's age category if necessary
|
|
$category = getProjectCategory($registration_id);
|
|
if($category !== null){
|
|
mysql_query("UPDATE projects SET projectcategories_id = $category WHERE registrations_id = $registration_id");
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::joinProject -> " . mysql_error();
|
|
}
|
|
}
|
|
|
|
|
|
return 'ok';
|
|
}
|
|
|
|
// disassociate the active user from the specified project registration. If the registration no longer
|
|
// has any users connected to it, delete it, and any projects tied to it
|
|
function removeProject($registrations_id){
|
|
// make sure this user is indeed connected to the specified project
|
|
$uid = $_SESSION['users_id'];
|
|
$regId = getRegistrationsId($uid);
|
|
$registrations_id = intval($registrations_id);
|
|
if($regId != $registrations_id){
|
|
return 'register_participants.inc.php::removeProject -> you are not connected to that project';
|
|
}
|
|
|
|
mysql_query("UPDATE users SET registrations_id = null WHERE id = $uid");
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::removeProject -> " . mysql_error();
|
|
}
|
|
|
|
// now let's see if anyone else is connected to that registration
|
|
$q = mysql_query("SELECT COUNT(*) AS tally FROM users WHERE registrations_id = $registrations_id");
|
|
$result = mysql_fetch_assoc($q);
|
|
if($result['tally'] == 0){
|
|
//nobody wants the poor lonely registration. Let's put it out of it's misery
|
|
|
|
mysql_query("DELETE FROM registrations WHERE id = $registrations_id");
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::removeProject -> " . mysql_error();
|
|
}
|
|
|
|
$projIds = array();
|
|
$q = mysql_query("SELECT id FROM projects WHERE registrations_id = $registrations_id");
|
|
while($row = mysql_fetch_assoc($q)){
|
|
$projIds[] = $row['id'];
|
|
}
|
|
if(count($projIds) > 0){
|
|
mysql_query("DELETE FROM project_specialawards_link
|
|
WHERE projects_id IN(" . implode('.', $projIds) . ")");
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::removeProject -> " . mysql_error();
|
|
}
|
|
}
|
|
|
|
mysql_query("DELETE FROM projects WHERE registrations_id = $registrations_id");
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::removeProject -> " . mysql_error();
|
|
}
|
|
|
|
}
|
|
|
|
return 'ok';
|
|
}
|
|
|
|
/********
|
|
More functions split off for API purposes - these ones for managing mentors
|
|
*******/
|
|
|
|
/** create a mentor that is tied to a particular registration **/
|
|
function addMentor($registrations_id){
|
|
global $conference;
|
|
//verify that the registrations id is a valid one:
|
|
$registrations_id = mysql_real_escape_string($registrations_id);
|
|
//echo "query = SELECT COUNT(*) AS tally FROM users WHERE id = " . $_SESSION['users_id'] . " AND registrations_id = " . $registrations_id . "<br/>";
|
|
$q = mysql_fetch_assoc(mysql_query("SELECT COUNT(*) AS tally FROM users WHERE id = " . $_SESSION['users_id'] . " AND registrations_id = " . $registrations_id));
|
|
if($q['tally'] != 1){
|
|
return "register_participants.inc.php::addMentor -> invalid registrations id";
|
|
}
|
|
|
|
// ok, let's go ahead and create a mentor
|
|
mysql_query("INSERT INTO mentors (registrations_id, conferences_id) VALUES($registrations_id, {$conference['id']})");
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::addMentor -> " . mysql_error();
|
|
}
|
|
|
|
// and now we can return an array that is the empty record for the mentor
|
|
$fields = 'id, firstname, lastname, email, phone, organization, position, description, conferences_id';
|
|
$mentorId = mysql_insert_id();
|
|
$q = mysql_query("SELECT $fields FROM mentors WHERE id = $mentorId");
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::addMentor -> " . mysql_error();
|
|
}
|
|
|
|
return mysql_fetch_assoc($q);
|
|
}
|
|
|
|
// find out if the specified user is allowed to edit the specified mentor. returns a boolean answer
|
|
function userCanEditMentor($userId, $mentorId){
|
|
// All necessary fields are there, now let's see if the record exists.
|
|
$row = mysql_fetch_assoc(mysql_query("SELECT registrations_id FROM mentors WHERE id = $mentorId"));
|
|
if(!$row){
|
|
return false;
|
|
}
|
|
$regId = $row['registrations_id'];
|
|
|
|
// Is this user connected to the same registration as this mentor?
|
|
$row = mysql_fetch_assoc(mysql_query("SELECT registrations_id FROM users WHERE id = $userId"));
|
|
if($row['registrations_id'] != $regId){
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
// return a list of fields that should be included in the mentor array
|
|
function getMentorFields(){
|
|
return array(
|
|
'id',
|
|
'firstname', 'lastname',
|
|
'email', 'phone',
|
|
'organization', 'position',
|
|
'description',
|
|
);
|
|
}
|
|
|
|
// take the passed array of data and save it to the corresponding record in the mentors table
|
|
function saveMentorData($data){
|
|
// Make sure all of the required fields have been included.
|
|
$missingFields = array();
|
|
$fields = getMentorFields();
|
|
foreach($fields as $key){
|
|
if(array_key_exists($key, $data)){
|
|
// might as well make 'em sql safe while we're here
|
|
$data[$key] = mysql_real_escape_string($data[$key]);
|
|
}else{
|
|
$missingFields[] = $key;
|
|
}
|
|
}
|
|
if(count($missingFields) != 0){
|
|
return "register_participants.inc.php::saveMentorData -> mentor object missing fields: " . implode(', ', $missingFields);
|
|
}
|
|
|
|
if(!userCanEditMentor($_SESSION['users_id'], $data['id'])){
|
|
return "register_participants.inc.php::saveMentorData -> current user not associated with the specified mentor";
|
|
}
|
|
|
|
// Ok, everything checks out. Let's go ahead and update the record.
|
|
$query = "UPDATE mentors SET ";
|
|
$queryParts = array();
|
|
foreach($fields as $key){
|
|
if($key == 'id') continue;
|
|
if($key == 'registrations_id') continue;
|
|
$queryParts[] = "`$key` = '{$data[$key]}' ";
|
|
}
|
|
$query .= implode(',', $queryParts);
|
|
$query .= "WHERE id = " . $data['id'];
|
|
mysql_query($query);
|
|
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::saveMentorData -> " . mysql_error();
|
|
}
|
|
|
|
return 'ok';
|
|
}
|
|
|
|
// delete the mentor whith the specified id
|
|
function removeMentor($mentorId){
|
|
$mentorId = intval($mentorId);
|
|
if(!userCanEditMentor($_SESSION['users_id'], $mentorId)){
|
|
return "register_participants.inc.php::removeMentor -> current user not associated with the specified mentor";
|
|
}
|
|
|
|
mysql_query("DELETE FROM mentors WHERE id = $mentorId");
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::removeMentor -> " . mysql_error();
|
|
}
|
|
|
|
return 'ok';
|
|
}
|
|
|
|
// get a list of all mentors associated with the specified registration
|
|
function getMentors($registrations_id){
|
|
// Is this user connected to the same registration as this mentor?
|
|
$row = mysql_fetch_assoc(mysql_query("SELECT registrations_id FROM users WHERE id = " . $_SESSION['users_id']));
|
|
if($row['registrations_id'] != $registrations_id){
|
|
return "register_participants.inc.php::getMentors -> current user not associated with the specified registration id";
|
|
}
|
|
|
|
$fields = getMentorFields();
|
|
$query = mysql_query("SELECT `" . implode('`,`', $fields) . "` FROM mentors WHERE registrations_id = $registrations_id ORDER BY id");
|
|
if(mysql_error()){
|
|
return "register_participants.inc.php::getMentors -> " . mysql_error();
|
|
}
|
|
$returnval = array();
|
|
while($row = mysql_fetch_assoc($query)){
|
|
$returnval[] = $row;
|
|
}
|
|
return $returnval;
|
|
}
|
|
|
|
function getSafetyQuestions($reg_id="") {
|
|
global $conference;
|
|
if($reg_id) $regId=$reg_id;
|
|
else $regId=$_SESSION['registration_id'];
|
|
|
|
//get their answers
|
|
$safetyanswers=array();
|
|
$q=mysql_query("SELECT * FROM safety WHERE registrations_id='$regId'");
|
|
while($r=mysql_fetch_object($q)) {
|
|
$safetyanswers[$r->safetyquestions_id]=$r->answer;
|
|
}
|
|
|
|
$ret=array();
|
|
//now get all the possible questiosn
|
|
$q=mysql_query("SELECT `id`,`question`,`type`,`required`,`ord` FROM safetyquestions WHERE conferences_id='".$conference['id']."' ORDER BY ord");
|
|
while($r=mysql_fetch_assoc($q)) {
|
|
if(array_key_exists($r['id'],$safetyanswers))
|
|
$r['answer']=$safetyanswers[$r['id']];
|
|
else
|
|
$r['answer']=null;
|
|
$ret[]=$r;
|
|
}
|
|
return $ret;
|
|
}
|
|
|
|
?>
|