forked from science-ation/science-ation
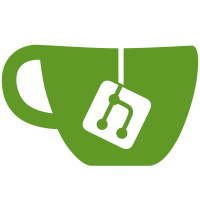
- Moved the tableeditor class to accept a generic class, no longer a person class - Restored the original functionality where everything is specified in the constructor, but added a 'class' which can be a dummy class to return to the original tableeditor functionality. The tableeditor checks for class->tableEditorLoad() now, and if that exists it calls it. If it doesn't then it calls a default Load() routine which contains the original code from the tableeditor. Same with save. Still needs work, but it's getting there.
189 lines
3.9 KiB
PHP
189 lines
3.9 KiB
PHP
<?
|
|
|
|
|
|
|
|
class person {
|
|
var $id;
|
|
// var $fields;
|
|
|
|
function person($person_id=NULL)
|
|
{
|
|
if($person_id == NULL) {
|
|
print("Empty constructor called\n");
|
|
$this->id = FALSE;
|
|
} else {
|
|
print("ID $person_id construction called\n");
|
|
$this->id = $person_id;
|
|
}
|
|
// $this->fields = NULL;
|
|
}
|
|
|
|
|
|
};
|
|
|
|
|
|
class judge extends person {
|
|
|
|
/* Static members for the table editor */
|
|
function tableEditorSetup($editor)
|
|
{
|
|
global $judges_fields;
|
|
/* Setup the table editor with the fields we want to display
|
|
* when displaying a list of judges, and also the type of each
|
|
* field where required */
|
|
$l = array( 'id' => 'ID',
|
|
'firstname' => 'First Name',
|
|
'lastname' => 'Last Name'
|
|
);
|
|
|
|
/* Most of these should be moved to the base class, as they
|
|
* will be the same for all person groups */
|
|
$e = array( 'firstname' => 'First Name',
|
|
'lastname' => 'Last Name',
|
|
'email' => 'Email Address',
|
|
'address' =>"Address 1",
|
|
'address2' =>"Address 2",
|
|
'city' => 'City',
|
|
'province' => 'Province',
|
|
'postalcode' => 'Postal Code',
|
|
'phonework' => 'Phone (Work)',
|
|
'phonecell' => 'Phone (Cell)',
|
|
'organization' => 'Organization',
|
|
'language' => 'Language(s)',
|
|
'complete' => "Complete" );
|
|
|
|
$editor->setTable('judges');
|
|
$editor->setListFields($l);
|
|
$editor->setEditFields($e);
|
|
|
|
/* Build an array of langauges that we support */
|
|
$langs = array();
|
|
$q=mysql_query("SELECT * FROM languages WHERE active='Y'");
|
|
while($r=mysql_fetch_object($q)) {
|
|
$langs[$r->lang] = $r->langname;
|
|
}
|
|
|
|
$editor->setFieldOptions('language', $langs);
|
|
$editor->setFieldInputType('language', 'multicheck');
|
|
}
|
|
|
|
/* Functions for $this */
|
|
|
|
|
|
function judge($judge_id=NULL)
|
|
{
|
|
global $judges_fields;
|
|
person::person($judge_id);
|
|
// $this->fields = $judges_fields;
|
|
}
|
|
|
|
function tableEditorLoad()
|
|
{
|
|
$id = $this->id;
|
|
|
|
print("Loading Judge ID $id\n");
|
|
|
|
$q=mysql_query("SELECT judges.*
|
|
FROM judges
|
|
WHERE judges.id='$id'");
|
|
echo mysql_error();
|
|
|
|
|
|
/* We assume that the field names in the array we want to return
|
|
* are the same as those in the database, so we'll turn the entire
|
|
* query into a single associative array */
|
|
$j = mysql_fetch_assoc($q);
|
|
|
|
/* Now turn on the ones this judge has selected */
|
|
$q=mysql_query("SELECT languages_lang
|
|
FROM judges_languages
|
|
WHERE judges_id='$id'");
|
|
while($r=mysql_fetch_object($q)) {
|
|
$j['language'][$r->languages_lang] = 1;
|
|
}
|
|
|
|
print_r($j);
|
|
|
|
return $j;
|
|
}
|
|
|
|
function tableEditorSave($data)
|
|
{
|
|
$judges_fields = array( 'firstname' => 'First Name',
|
|
'lastname' => 'Last Name',
|
|
'email' => 'Email Address',
|
|
'address' =>"Address 1",
|
|
'address2' =>"Address 2",
|
|
'city' => 'City',
|
|
'province' => 'Province',
|
|
'postalcode' => 'Postal Code',
|
|
'phonework' => 'Phone (Work)',
|
|
'phonecell' => 'Phone (Cell)',
|
|
'organization' => 'Organization',
|
|
'complete' => "Complete" );
|
|
|
|
$query = "";
|
|
|
|
$insert_mode = ($this->id == false) ? 1 : 0;
|
|
|
|
if($insert_mode) {
|
|
$query="INSERT INTO judges (";
|
|
//create list of fields to insert
|
|
foreach($data AS $f=>$n)
|
|
$query.="`$f`,";
|
|
//rip off the last comma
|
|
$query=substr($query,0,-1);
|
|
$query.=") VALUES (";
|
|
} else {
|
|
$query="UPDATE `judges` SET ";
|
|
}
|
|
|
|
foreach($judges_fields AS $f=>$n)
|
|
{
|
|
if($insert_mode) $field = '';
|
|
else $field = "`$f`=";
|
|
|
|
$n = $data[$f];
|
|
|
|
$query .= $field.$n.",";
|
|
}
|
|
//rip off the last comma
|
|
$query=substr($query,0,-1);
|
|
|
|
if($insertmode) {
|
|
$query.=")";
|
|
} else {
|
|
$query.=" WHERE id='{$this->id}'";
|
|
}
|
|
|
|
echo $query;
|
|
// mysql_query($query);
|
|
|
|
print("data: \n");
|
|
print_r($data);
|
|
print("-- \n");
|
|
|
|
/* judges_languages */
|
|
/* First delete all the languages, then insert the ones the judge
|
|
* has selected */
|
|
$query = "DELETE FROM judges_languages WHERE judges_id='{$this->id}'";
|
|
//mysql_query($query);
|
|
|
|
print_r($data['language']);
|
|
$keys = array_keys($data['language']);
|
|
foreach($keys as $k) {
|
|
$query = "INSERT INTO
|
|
judges_languages (judges_id,languages_lang)
|
|
VALUES ('{$this->id}','$k')";
|
|
print("$query");
|
|
// mysql_query($query);
|
|
|
|
}
|
|
|
|
|
|
}
|
|
|
|
};
|
|
|
|
?>
|