forked from science-ation/science-ation
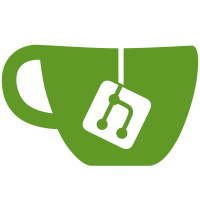
- Add the ability to specify a filter when calling for a report generation (e.g., so we can dynamically filter a report for a specific fundraising campaign)
247 lines
8.0 KiB
PHP
247 lines
8.0 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require_once("../common.inc.php");
|
|
require_once("../user.inc.php");
|
|
user_auth_required('committee', 'admin');
|
|
require_once('reports.inc.php');
|
|
|
|
|
|
$id = intval($_GET['id']);
|
|
$type = stripslashes($_GET['type']);
|
|
$stock = stripslashes($_GET['stock']);
|
|
$year = intval($_GET['year']);
|
|
$show_options = array_key_exists('show_options', $_GET);
|
|
if($year < 1000) $year = $config['FAIRYEAR'];
|
|
|
|
/* If it's a system report, turn that into the actual report id */
|
|
if(array_key_exists('sid', $_GET)) {
|
|
$sid = intval($_GET['sid']);
|
|
$q = mysql_query("SELECT id FROM reports WHERE system_report_id='$sid'");
|
|
$r = mysql_fetch_assoc($q);
|
|
$id = $r['id'];
|
|
}
|
|
|
|
switch($_GET['action']) {
|
|
case 'dialog_gen':
|
|
/* Add a custom filter */
|
|
$report = report_load($id);
|
|
if(is_array($_GET['filter'])) {
|
|
foreach($_GET['filter'] as $f=>$v) {
|
|
$report['options']['filter'][$f] = $v;
|
|
}
|
|
}
|
|
?>
|
|
<div id="report_dialog_gen" title="Generate Report" style="display: none">
|
|
<form id="report_dialog_form" >
|
|
<input type="hidden" name="id" value="<?=$id?>" />
|
|
<table class="editor" style="width:95%"><tr>
|
|
<td colspan="2"><br /><h3><?=i18n('Report Information')?></h3><br /></td>
|
|
</tr><tr>
|
|
<td class="label"><b><?=i18n("Report Name")?></b>:</td>
|
|
<td class="input"><?=$report['name']?></b></td>
|
|
</tr><tr>
|
|
<td class="label"><b><?=i18n("Description")?></b>:</td>
|
|
<td class="input"><?=$report['desc']?></b></td>
|
|
</tr><tr>
|
|
<td class="label"><b><?=i18n("Created By")?></b>:</td>
|
|
<td class="input"><?=$report['creator']?></td>
|
|
</tr><tr>
|
|
<?
|
|
/* See if the report is in this committee member's list */
|
|
$q = mysql_query("SELECT * FROM reports_committee
|
|
WHERE users_id='{$_SESSION['users_uid']}'
|
|
AND reports_id='{$report['id']}'");
|
|
if(mysql_num_rows($q) > 0) {
|
|
$i = mysql_fetch_assoc($q);
|
|
?>
|
|
<td colspan="2"><hr /><h3><?=i18n('My Reports Info')?></h3></td>
|
|
</tr><tr>
|
|
<td class="label"><b><?=i18n("Category")?></b>:</td>
|
|
<td class="input"><?=$i['category']?></b></td>
|
|
</tr><tr>
|
|
<td class="label"><b><?=i18n("Comment")?></b>:</td>
|
|
<td class="input"><?=$i['comment']?></b></td>
|
|
</tr><tr>
|
|
<? } ?>
|
|
|
|
<td colspan="2"><br /><hr /><h3><?=i18n('Report Options')?></h3><br /></td>
|
|
</tr>
|
|
<?
|
|
$format = $report['options']['type'];
|
|
$stock = $report['options']['stock'];
|
|
$year = $config['FAIRYEAR'];
|
|
|
|
/* Out of all the report optins, we really only want these ones */
|
|
$option_keys = array('type','stock');
|
|
foreach($report_options as $ok=>$o) {
|
|
if(!in_array($ok, $option_keys)) continue;
|
|
|
|
echo "<tr><td class=\"label\"><b>{$o['desc']}</b>:</td>";
|
|
echo "<td class=\"input\"><select name=\"$ok\" id=\"$ok\">";
|
|
foreach($o['values'] as $k=>$v) {
|
|
$sel = ($report['option'][$ok] == $k) ? 'selected=\"selected\"' : '';
|
|
echo "<option value=\"$k\" $sel>$v</option>";
|
|
}
|
|
echo "</select></td></tr>";
|
|
}
|
|
/* Find all the years */
|
|
$q = mysql_query("SELECT DISTINCT year FROM config WHERE year>1000 ORDER BY year DESC");
|
|
echo "<tr><td class=\"label\"><b>".i18n('Year')."</b>:</td>";
|
|
echo "<td class=\"input\"><select name=\"year\" id=\"year\">";
|
|
while($i = mysql_fetch_assoc($q)) {
|
|
$y = $i['year'];
|
|
$sel = ($config['FAIRYEAR'] == $y) ? 'selected=\"selected\"' : '';
|
|
echo "<option value=\"$y\" $sel>$y</option>";
|
|
}
|
|
echo "</select></td></tr>";
|
|
?>
|
|
</table>
|
|
<br />
|
|
<hr />
|
|
<div align="right">
|
|
<input type="submit" id="report_dialog_gen_pdf_button" value="<?=i18n('Download PDF')?>" />
|
|
<input type="submit" id="report_dialog_gen_cancel_button" value="<?=i18n('Cancel')?>" />
|
|
</form>
|
|
</div>
|
|
<script type="text/javascript">
|
|
$("#report_dialog_gen_pdf_button").click(function () {
|
|
var dlargs = $('#report_dialog_form').serialize();
|
|
var dlurl = "<?=$config['SFIABDIRECTORY']?>/admin/reports_gen.php?"+dlargs;
|
|
$('#content').attr('src',dlurl);
|
|
$('#report_dialog_gen').dialog("close");
|
|
return false;
|
|
});
|
|
$("#report_dialog_gen_cancel_button").click(function () {
|
|
$('#report_dialog_gen').dialog("close");
|
|
return false;
|
|
});
|
|
|
|
$("#report_dialog_gen").dialog({
|
|
bgiframe: true, autoOpen: true,
|
|
modal: true, resizable: false,
|
|
draggable: false,
|
|
width: 800, //(document.documentElement.clientWidth * 0.8);
|
|
height: (document.documentElement.clientHeight * 0.5),
|
|
close: function() {
|
|
$(this).dialog('destroy');
|
|
$('#report_dialog_gen').remove();
|
|
}
|
|
});
|
|
|
|
</script>
|
|
<?
|
|
exit;
|
|
}
|
|
|
|
|
|
if($show_options == false) {
|
|
if($id && $year) {
|
|
$report = report_load($id);
|
|
$report['year'] = $year;
|
|
if($type != '') $report['option']['type'] = $type;
|
|
if($stock != '') $report['option']['stock'] = $stock;
|
|
report_gen($report);
|
|
} else {
|
|
exit;
|
|
header("Location: reports.php");
|
|
}
|
|
exit;
|
|
}
|
|
|
|
$report = report_load($id);
|
|
|
|
send_header('Report Options', array(
|
|
'Committee Main' => 'committee_main.php',
|
|
'My Reports' => 'admin/reports.php'));
|
|
|
|
echo '<form method=\"get\" action="reports_gen.php">';
|
|
echo "<input type=\"hidden\" name=\"id\" value=\"$id\">";
|
|
|
|
echo '<table class="tableedit">';
|
|
echo "<tr><td><b>".i18n('Report Name')."</b>:</td>";
|
|
echo "<td>{$report['name']}</td></tr>";
|
|
echo "<tr><td><b>".i18n('Description')."</b>:</td>";
|
|
echo "<td>{$report['desc']}</td></tr>";
|
|
echo "<tr><td><b>".i18n('Created By')."</b>:</td>";
|
|
echo "<td>{$report['creator']}</td></tr>";
|
|
|
|
echo '<tr><td colspan="2"><hr /></td></tr>';
|
|
/* See if the report is in this committee member's list */
|
|
$q = mysql_query("SELECT * FROM reports_committee
|
|
WHERE users_id='{$_SESSION['users_uid']}'
|
|
AND reports_id='{$report['id']}'");
|
|
echo "<tr><td colspan=\"2\"><h3>".i18n('My Reports Info')."</h3></td></tr>";
|
|
if(mysql_num_rows($q) > 0) {
|
|
/* Yes, it is */
|
|
$i = mysql_fetch_object($q);
|
|
echo "<tr><td><b>".i18n('Category')."</b>:</td>";
|
|
echo "<td>{$i->category}</td></tr>";
|
|
echo "<tr><td><b>".i18n('Comment')."</b>:</td>";
|
|
echo "<td>{$i->comment}</td></tr>";
|
|
} else {
|
|
echo "<tr><td></td><td>".i18n('This report is NOT in your \'My Reports\' list.')."</td></tr>";
|
|
}
|
|
echo '<tr><td colspan="2"><hr /></td></tr>';
|
|
echo "<tr><td colspan=\"2\"><h3>".i18n('Report Options')."</h3></td></tr>";
|
|
|
|
$format = $report['options']['type'];
|
|
$stock = $report['options']['stock'];
|
|
$year = $config['FAIRYEAR'];
|
|
|
|
/* Out of all the report optoins, we really only want these ones */
|
|
$option_keys = array('type','stock');
|
|
foreach($report_options as $ok=>$o) {
|
|
if(!in_array($ok, $option_keys)) continue;
|
|
echo "<tr><td><b>{$o['desc']}</b>:</td>";
|
|
echo "<td><select name=\"$ok\" id=\"$ok\">";
|
|
foreach($o['values'] as $k=>$v) {
|
|
$sel = ($report['option'][$ok] == $k) ? 'selected=\"selected\"' : '';
|
|
echo "<option value=\"$k\" $sel>$v</option>";
|
|
}
|
|
echo "</select></td></tr>";
|
|
}
|
|
/* Find all the years */
|
|
$q = mysql_query("SELECT DISTINCT year FROM config WHERE year>1000 ORDER BY year DESC");
|
|
echo "<tr><td><b>".i18n('Year')."</b>:</td>";
|
|
echo "<td><select name=\"year\" id=\"year\">";
|
|
while($i = mysql_fetch_assoc($q)) {
|
|
$y = $i['year'];
|
|
$sel = ($config['FAIRYEAR'] == $y) ? 'selected=\"selected\"' : '';
|
|
echo "<option value=\"$y\" $sel>$y</option>";
|
|
}
|
|
echo "</select></td></tr>";
|
|
|
|
echo "</table>";
|
|
|
|
echo '<br />';
|
|
echo "<input type=\"submit\" value=\"".i18n('Generate Report')."\" />";
|
|
echo '</form>';
|
|
|
|
send_footer();
|
|
|
|
|
|
|
|
?>
|