forked from science-ation/science-ation
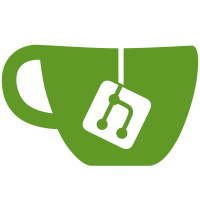
Add the translation dropdown to a few fields in teh award sponsors, awards and award prizes.
246 lines
5.6 KiB
PHP
246 lines
5.6 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
|
|
<script type="text/javascript">
|
|
|
|
function getHTTPObject()
|
|
{
|
|
var xmlhttp;
|
|
/*@cc_on
|
|
@if (@_jscript_version >= 5)
|
|
try {
|
|
xmlhttp = new ActiveXObject("Msxml2.XMLHTTP");
|
|
} catch (e) {
|
|
try {
|
|
xmlhttp = new ActiveXObject("Microsoft.XMLHTTP");
|
|
} catch (E) {
|
|
xmlhttp = false;
|
|
}
|
|
}
|
|
@else
|
|
xmlhttp = false;
|
|
@end @*/
|
|
if (!xmlhttp && typeof XMLHttpRequest != 'undefined')
|
|
{
|
|
try {
|
|
xmlhttp = new XMLHttpRequest();
|
|
} catch (e)
|
|
{
|
|
xmlhttp = false;
|
|
}
|
|
}
|
|
return xmlhttp;
|
|
}
|
|
|
|
|
|
var http=getHTTPObject();
|
|
var queryInProgress=false;
|
|
var translateOpen=false;
|
|
var translationObject;
|
|
var langstr="<? foreach($config['languages'] AS $l=>$ln){ $lstr.="$l/"; } echo substr($lstr,0,-1)?>";
|
|
|
|
var jsTranslateLangs=new Array();
|
|
<?
|
|
$x=0;
|
|
foreach($config['languages'] AS $l=>$ln)
|
|
{
|
|
if($l=="en")continue;
|
|
echo "jsTranslateLangs[$x]=\"$l\";\n";
|
|
$x++;
|
|
}
|
|
?>
|
|
|
|
|
|
function getTranslations(o)
|
|
{
|
|
var objItem=document.getElementById(o);
|
|
var url="<?=$config['SFIABDIRECTORY']?>/admin/gettranslation.php?str="+escape(objItem.value);
|
|
|
|
if(!queryInProgress)
|
|
{
|
|
queryInProgress=true;
|
|
http.open("GET", url , true);
|
|
http.onreadystatechange = handleResponse;
|
|
http.send(null);
|
|
}
|
|
}
|
|
|
|
function handleResponse()
|
|
{
|
|
try {
|
|
|
|
if(http.readyState==4)
|
|
{
|
|
queryInProgress=false;
|
|
var d=document.getElementById('translationdropdown');
|
|
// alert(completeField);
|
|
|
|
try {
|
|
results = http.responseText.split('\n');
|
|
var num=results.length;
|
|
|
|
for(i=0;i<results.length;i++)
|
|
{
|
|
if(!results[i]){ num--; continue; }
|
|
|
|
line = results[i].split(':');
|
|
//line[0] has "en" or "fr" or the lang code
|
|
//line[1] has the actual translation for that language
|
|
objInput=document.getElementById('translation['+line[0]+']');
|
|
if(line[1])
|
|
{
|
|
//alert(objInput);
|
|
objInput.value=line[1];
|
|
}
|
|
else
|
|
{
|
|
objInput.value="";
|
|
}
|
|
}
|
|
}
|
|
catch(e)
|
|
{
|
|
|
|
}
|
|
}
|
|
|
|
}
|
|
catch(e)
|
|
{
|
|
}
|
|
}
|
|
|
|
function translateButton(o)
|
|
{
|
|
document.write("<a href=\"#\" onclick=\"return translateDropdown('"+o+"')\"><?=i18n("translations")?></a>");
|
|
}
|
|
function translateDropdown(o)
|
|
{
|
|
translationObject=document.getElementById(o);
|
|
var d=document.getElementById('translationdropdown');
|
|
if(translateOpen)
|
|
d.style.visibility="hidden";
|
|
else
|
|
{
|
|
getTranslations(o);
|
|
objItem=translationObject;
|
|
|
|
var tagheight=objItem.offsetHeight;
|
|
var tagwidth=objItem.offsetWidth;
|
|
|
|
//alert('tagheight='+tagheight+' tagwidth='+tagwidth);
|
|
|
|
var intx=0;
|
|
var inty=0;
|
|
|
|
do
|
|
{
|
|
intx+=objItem.offsetLeft;
|
|
inty+=objItem.offsetTop;
|
|
objParent=objItem.offsetParent.tagName;
|
|
objItem=objItem.offsetParent;
|
|
} while(objParent!="BODY");
|
|
|
|
d.style.top=inty+tagheight+"px";
|
|
d.style.left=intx+"px";
|
|
d.style.width=tagwidth+"px";
|
|
// d.style.height=dropdownheight+"px";
|
|
d.style.visibility="visible";
|
|
|
|
oButton=document.getElementById('buttonsavetranslations');
|
|
oButton.value="<?=i18n("Save Translations")?>";
|
|
|
|
}
|
|
translateOpen=!translateOpen;
|
|
return false;
|
|
|
|
}
|
|
|
|
function savetranslations()
|
|
{
|
|
var url="<?=$config['SFIABDIRECTORY']?>/admin/settranslation.php?str="+escape(translationObject.value);
|
|
var x;
|
|
for(x=0;x<jsTranslateLangs.length;x++)
|
|
{
|
|
oTmp=document.getElementById('translation['+jsTranslateLangs[x]+']');
|
|
url+="&"+jsTranslateLangs[x]+"="+escape(oTmp.value);
|
|
}
|
|
oButton=document.getElementById('buttonsavetranslations');
|
|
oButton.value="<?=i18n("Saving translations...")?>";
|
|
|
|
if(!queryInProgress)
|
|
{
|
|
queryInProgress=true;
|
|
http.open("GET", url , true);
|
|
http.onreadystatechange = handleSaveResponse;
|
|
http.send(null);
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
function handleSaveResponse()
|
|
{
|
|
try {
|
|
|
|
if(http.readyState==4)
|
|
{
|
|
queryInProgress=false;
|
|
var d=document.getElementById('translationdropdown');
|
|
|
|
oButton=document.getElementById('buttonsavetranslations');
|
|
oButton.value="<?=i18n("Translations Saved")?>";
|
|
|
|
setTimeout("translateDropdown('dummy');",500); //it doesnt matter what obj we use, because we're just clearing it anyways
|
|
|
|
//who cares what happens, we're done thats all we care about
|
|
|
|
}
|
|
|
|
}
|
|
catch(e)
|
|
{
|
|
}
|
|
}
|
|
</script>
|
|
|
|
<?
|
|
$num=count($config['languages'])-1; //subtract 1 for english
|
|
$divheight=($num*30)+50;
|
|
?>
|
|
<div name="translationdropdown" id="translationdropdown" style="background-color: white; visibility: hidden; position: absolute; border: 1px solid grey; top:0px; left:0px; width:300px; height:<?=$divheight?>px; overflow: auto">
|
|
<form name="translationform" method="get">
|
|
<table width="95%">
|
|
<?
|
|
foreach($config['languages'] AS $l=>$ln)
|
|
{
|
|
if($l=="en") continue;
|
|
echo "<tr><td>$ln</td><td><input type=\"text\" style=\"width: 100%\" id=\"translation[$l]\" name=\"translation[$l]\"></td></tr>";
|
|
}
|
|
echo "<tr><td colspan=\"2\" align=\"center\"><input type=\"button\" id=\"buttonsavetranslations\" onclick=\"return savetranslations()\" value=\"".i18n("Save Translations")."\"></td></tr>";
|
|
?>
|
|
</table>
|
|
</form>
|
|
</div>
|