forked from science-ation/science-ation
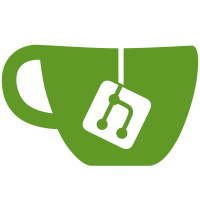
Tweak award eligibility display Add "Account" info to student reports (email, username, pendingemail) Move some project identification stuff around and fix the groupings
1065 lines
33 KiB
PHP
1065 lines
33 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
function report_students_i18n_fr(&$report, $field, $text)
|
|
{
|
|
return i18n($text, array(), array(), 'fr');
|
|
}
|
|
|
|
function reports_students_numstudents(&$report, $field, $text)
|
|
{
|
|
$conferences_id = $report['conferences_id'];
|
|
$q = mysql_query("SELECT users.id FROM users
|
|
WHERE users.registrations_id='$text'
|
|
AND users.conferences_id='$conferences_id'");
|
|
return mysql_num_rows($q);
|
|
}
|
|
|
|
function report_student_safety_question(&$report, $field, $text) {
|
|
|
|
|
|
/* Field is 'safetyquestion_x', registration_id is passed in $text */
|
|
$q_ord = intval(substr($field, 15));
|
|
$conferences_id = $report['conferences_id'];
|
|
$regid = $text;
|
|
|
|
//safetyquestions start counting 1-10, but when we LIMIT, we need to index on 0-9
|
|
$q_ord--;
|
|
|
|
$q=mysql_query("SELECT safetyquestions.question,
|
|
safety.answer
|
|
FROM safetyquestions
|
|
JOIN safety ON safetyquestions.id=safety.safetyquestions_id
|
|
WHERE safety.registrations_id='".$regid."'
|
|
ORDER BY safetyquestions.ord LIMIT $q_ord,1");
|
|
|
|
$r=mysql_fetch_object($q);
|
|
return $r->answer;
|
|
}
|
|
|
|
|
|
|
|
function reports_students_award_selfnom_num(&$report, $field, $text, $n)
|
|
{
|
|
$conferences_id = $report['conferences_id'];
|
|
$q = mysql_query("SELECT award_awards.name FROM
|
|
projects
|
|
LEFT JOIN project_specialawards_link ON project_specialawards_link.projects_id=projects.id
|
|
LEFT JOIN award_awards ON award_awards.id=project_specialawards_link.award_awards_id
|
|
WHERE projects.id='$text'
|
|
AND projects.conferences_id='$conferences_id'
|
|
AND project_specialawards_link.conferences_id='$conferences_id'
|
|
LIMIT $n,1");
|
|
echo mysql_error();
|
|
$i = mysql_fetch_assoc($q);
|
|
return $i['name'];
|
|
}
|
|
function reports_students_award_selfnom_1(&$report, $field, $text)
|
|
{
|
|
return reports_students_award_selfnom_num(&$report, $field, $text, 0);
|
|
}
|
|
function reports_students_award_selfnom_2(&$report, $field, $text)
|
|
{
|
|
return reports_students_award_selfnom_num(&$report, $field, $text, 1);
|
|
}
|
|
function reports_students_award_selfnom_3(&$report, $field, $text)
|
|
{
|
|
return reports_students_award_selfnom_num(&$report, $field, $text, 2);
|
|
}
|
|
function reports_students_award_selfnom_4(&$report, $field, $text)
|
|
{
|
|
return reports_students_award_selfnom_num(&$report, $field, $text, 3);
|
|
}
|
|
function reports_students_award_selfnom_5(&$report, $field, $text)
|
|
{
|
|
return reports_students_award_selfnom_num(&$report, $field, $text, 4);
|
|
}
|
|
function reports_students_school_principal(&$report, $field, $text)
|
|
{
|
|
if($text > 0) { /* text is the uid */
|
|
$u = user_load($text);
|
|
return $u['name'];
|
|
}
|
|
return '';
|
|
}
|
|
|
|
|
|
$report_students_fields = array(
|
|
'pn' => array(
|
|
'start_option_group' => 'Project Identification',
|
|
'name' => 'Project Number',
|
|
'header' => '#',
|
|
'width' => 18 /*mm*/,
|
|
'table' => 'projects.projectnumber',
|
|
'table_sort' => 'projects.projectsort, projects.projectnumber'),
|
|
|
|
'projectbarcode' => array(
|
|
'name' => 'Project Barcode',
|
|
'header' => 'Barcode',
|
|
'width' => 1,
|
|
'table' => 'projects.projectnumber',
|
|
'table_sort' => 'projects.projectsort, projects.projectnumber',
|
|
),
|
|
|
|
'registrations_num' => array(
|
|
'name' => 'Registration Number',
|
|
'header' => 'RegNum',
|
|
'width' => 16 /*mm*/,
|
|
'table' => 'registrations.num' ),
|
|
|
|
'paid' => array(
|
|
'name' => 'Paid',
|
|
'header' => 'Paid',
|
|
'width' => 10 /*mm*/,
|
|
'table' => 'registrations.status',
|
|
'value_map' => array ('complete' => '', 'paymentpending' => 'No')),
|
|
|
|
'email' => array(
|
|
'start_option_group' => 'Account Information',
|
|
'name' => 'Account -- Email',
|
|
'header' => 'Email',
|
|
'width' => 60 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'accounts.email'),
|
|
|
|
'pendingemail' => array(
|
|
'name' => 'Account -- Pending Email',
|
|
'header' => 'Email',
|
|
'width' => 60 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'accounts.pendingemail'),
|
|
|
|
'username' => array(
|
|
'name' => 'Account -- Username',
|
|
'header' => 'Username',
|
|
'width' => 60 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'accounts.username'),
|
|
|
|
'last_name' => array(
|
|
'start_option_group' => 'Student Name Information',
|
|
'name' => 'Student -- Last Name',
|
|
'header' => 'Last Name',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => 'users.lastname' ),
|
|
|
|
'first_name' => array(
|
|
'name' => 'Student -- First Name',
|
|
'header' => 'First Name',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => 'users.firstname' ),
|
|
|
|
'name' => array(
|
|
'name' => 'Student -- Full Name (last, first)',
|
|
'header' => 'Name',
|
|
'width' => 40.45 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "CONCAT(users.lastname, ', ', users.firstname)",
|
|
'table_sort'=> 'users.lastname' ),
|
|
|
|
'namefl' => array(
|
|
'name' => 'Student -- Full Name (first last)',
|
|
'header' => 'Name',
|
|
'width' => 44.45 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "CONCAT(users.firstname, ' ', users.lastname)",
|
|
'table_sort'=> 'users.lastname' ),
|
|
|
|
'partner' => array(
|
|
'name' => 'Student -- Partner Name (last, first)',
|
|
'header' => 'Partner',
|
|
'width' => 38.1 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "CONCAT(students2.lastname, ', ', students2.firstname)",
|
|
'components' => array('partner') ),
|
|
|
|
'partnerfl' => array(
|
|
'name' => 'Student -- Partner Name (first last)',
|
|
'header' => 'Partner',
|
|
'width' => 38.1 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "CONCAT(students2.firstname, ' ', students2.lastname)",
|
|
'components' => array('partner') ),
|
|
|
|
'bothnames' => array(
|
|
'name' => "Student -- Both Student Names",
|
|
'header' => 'Student(s)',
|
|
'width' => 76.2 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "CONCAT(users.firstname, ' ', users.lastname, IF(students2.lastname IS NULL,'', CONCAT(', ', students2.firstname, ' ', students2.lastname)))",
|
|
'table_sort' => 'users.lastname',
|
|
'components' => array('partner') ),
|
|
|
|
'allnames' => array(
|
|
'name' => "Student -- All Student Names (REQUIRES MYSQL 5.0) ",
|
|
'header' => 'Student(s)',
|
|
'width' => 76.2 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "GROUP_CONCAT(users.firstname, ' ', users.lastname ORDER BY users.lastname SEPARATOR ', ')",
|
|
'group_by' => array('users.registrations_id')),
|
|
|
|
'pronunciation' => array(
|
|
'name' => 'Student -- Name Pronunciation',
|
|
'header' => 'Pronunciation',
|
|
'width' => 50.8 /*mm*/,
|
|
'table' => 'users.pronunciation'),
|
|
|
|
'phone' => array(
|
|
'name' => 'Student -- Phone',
|
|
'header' => 'Phone',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => 'users.phone'),
|
|
|
|
'address' => array(
|
|
'name' => 'Student -- Street Address',
|
|
'header' => 'Address',
|
|
'width' => 50.8 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'users.address'),
|
|
|
|
'city' => array(
|
|
'name' => 'Student -- City',
|
|
'header' => 'City',
|
|
'width' => 38.1 /*mm*/,
|
|
'table' => 'users.city' ),
|
|
|
|
'province' => array(
|
|
'name' => 'Student -- '.$config['provincestate'],
|
|
'header' => $config['provincestate'],
|
|
'width' => 19.05 /*mm*/,
|
|
'table' => 'users.province' ),
|
|
|
|
'postal' => array(
|
|
'name' => 'Student -- '.$config['postalzip'],
|
|
'header' => $config['postalzip'],
|
|
'width' => 19.05 /*mm*/,
|
|
'table' => 'users.postalcode' ),
|
|
|
|
'address_full' => array(
|
|
'name' => 'Student -- Full Address',
|
|
'header' => 'Address',
|
|
'width' => 76.2 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "CONCAT(users.address, ', ', users.city, ', ', users.province, ', ', users.postalcode)" ),
|
|
|
|
|
|
'grade' => array(
|
|
'start_option_group' => 'Other Student Information',
|
|
'name' => 'Student -- Grade',
|
|
'header' => 'Gr.',
|
|
'width' => 7.62 /*mm*/,
|
|
'table' => 'users.grade'),
|
|
|
|
'grade_str' => array(
|
|
'name' => 'Student -- Grade ("Grade x", not just the number)',
|
|
'header' => 'Gr.',
|
|
'width' => 7.62 /*mm*/,
|
|
'table_sort' => 'users.grade',
|
|
'table' => "CONCAT('Grade ', users.grade)"),
|
|
|
|
'gender' => array(
|
|
'name' => 'Student -- Gender',
|
|
'header' => 'Gender',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => 'users.sex',
|
|
'value_map' =>array ('male' => 'Male', 'female' => 'Female')),
|
|
|
|
'birthdate' => array(
|
|
'name' => 'Student -- Birthdate',
|
|
'header' => 'Birthdate',
|
|
'width' => 22.86 /*mm*/,
|
|
'table' => 'users.dateofbirth'),
|
|
|
|
'age' => array(
|
|
'name' => 'Student -- Age (when this report is created)',
|
|
'header' => 'Age',
|
|
'width' => 10.16 /*mm*/,
|
|
'table' => "DATE_FORMAT(FROM_DAYS(TO_DAYS(NOW())-TO_DAYS(users.birthdate)), '%Y')+0",
|
|
'table_sort' => 'users.birthdate'),
|
|
|
|
'tshirt' => array(
|
|
'name' => 'Student -- T-Shirt Size',
|
|
'header' => 'T-Shirt',
|
|
'width' => 13.97 /*mm*/,
|
|
'table' => 'users.tshirt',
|
|
'value_map' => array ('none' => '', 'xsmall' => 'X-Small', 'small' => 'Small', 'medium' => 'Medium',
|
|
'large' => 'Large', 'xlarge' => 'X-Large')),
|
|
|
|
'medicalalert' => array(
|
|
'name' => 'Student -- Medical Alert Info',
|
|
'header' => 'medical',
|
|
'width' => 50.8 /*mm*/,
|
|
'table' => 'users.medicalalert'),
|
|
|
|
'foodreq' => array(
|
|
'name' => 'Student -- Food Requirements',
|
|
'header' => 'Food.Req.',
|
|
'width' => 50.8 /*mm*/,
|
|
'table' => 'users.foodreq'),
|
|
|
|
/* Project Information */
|
|
'title' => array(
|
|
'start_option_group' => 'Project Information',
|
|
'name' => 'Project -- Title',
|
|
'header' => 'Project Title',
|
|
'width' => 65 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'projects.title' ),
|
|
|
|
'shorttitle' => array(
|
|
'name' => 'Project -- Short Title',
|
|
'header' => 'Short Title',
|
|
'width' => 50.8 /*mm*/,
|
|
'table' => 'projects.shorttitle' ),
|
|
|
|
'division' => array(
|
|
'name' => 'Project -- Division',
|
|
'header' => 'Division',
|
|
'width' => 76.2 /*mm*/,
|
|
'table' => 'projectdivisions.division' ),
|
|
|
|
'div' => array(
|
|
'name' => 'Project -- Division Short Form' ,
|
|
'header' => 'Div',
|
|
'width' => 10.16 /*mm*/,
|
|
'table' => 'projectdivisions.division_shortform' ),
|
|
|
|
'fr_division' => array(
|
|
'name' => 'Project -- Division (French)',
|
|
'header' => i18n('Division', array(), array(), 'fr'),
|
|
'width' => 76.2 /*mm*/,
|
|
'table' => 'projectdivisions.division',
|
|
'exec_function' => 'report_students_i18n_fr'),
|
|
|
|
'category' => array(
|
|
'name' => 'Project -- Category',
|
|
'header' => 'Category',
|
|
'width' => 25.4 /*mm*/,
|
|
'table_sort' => 'projectcategories.id',
|
|
'table' => 'projectcategories.category' ),
|
|
|
|
'cat' => array(
|
|
'name' => 'Project -- Category Short Form' ,
|
|
'header' => 'cat',
|
|
'width' => 10.16 /*mm*/,
|
|
'table' => 'projectcategories.category_shortform' ),
|
|
|
|
'fr_category' => array(
|
|
'name' => 'Project -- Category (French)',
|
|
'header' => i18n('Category', array(), array(), 'fr'),
|
|
'width' => 25.4 /*mm*/,
|
|
'table_sort' => 'projectcategories.id',
|
|
'table' => 'projectcategories.category',
|
|
'exec_function' => 'report_students_i18n_fr'),
|
|
|
|
'categorydivision' => array(
|
|
'name' => 'Project -- Category and Division',
|
|
'header' => 'Category/Division',
|
|
'width' => 88.9 /*mm*/,
|
|
'table_sort' => 'projectcategories.id',
|
|
'table' => "CONCAT(projectcategories.category,' - ', projectdivisions.division)"),
|
|
|
|
'divisioncategory' => array(
|
|
'name' => 'Project -- Division and Category',
|
|
'header' => 'Division/Category',
|
|
'width' => 88.9 /*mm*/,
|
|
'table_sort' => 'projectdivisions.id',
|
|
'table' => "CONCAT(projectdivisions.division,' - ',projectcategories.category)"),
|
|
|
|
'summary' => array(
|
|
'name' => 'Project -- Summary',
|
|
'header' => 'Project Summary',
|
|
'width' => 101.6 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'projects.summary' ),
|
|
|
|
'language' => array(
|
|
'name' => 'Project -- Language',
|
|
'header' => 'Lang',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => 'projects.language' ),
|
|
|
|
'numstudents' => array(
|
|
'name' => 'Project -- Number of Students',
|
|
'header' => 'Stu.',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'reports_students_numstudents'),
|
|
|
|
'rank' => array(
|
|
'name' => 'Project -- Rank (left blank for judges to fill out)',
|
|
'header' => 'Rank',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => '""' ),
|
|
|
|
'req_elec' => array(
|
|
'name' => 'Project -- If the project requires electricity',
|
|
'header' => 'Elec',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => "projects.req_electricity",
|
|
'value_map' => array ('no' => '', 'yes' => 'Yes')),
|
|
|
|
'req_table' => array(
|
|
'name' => 'Project -- If the project requires a table',
|
|
'header' => 'Table',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => "projects.req_table",
|
|
'value_map' => array ('no' => '', 'yes' => 'Yes')),
|
|
|
|
'req_special' => array(
|
|
'name' => 'Project -- Any special requirements the project has',
|
|
'header' => 'Special Requirements',
|
|
'width' => 76.2 /*mm*/,
|
|
'table' => "projects.req_special"),
|
|
|
|
|
|
'school' => array(
|
|
'start_option_group' => 'School Information',
|
|
'name' => 'School -- Name',
|
|
'header' => 'School Name',
|
|
'width' => 57.15 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'schools.school' ),
|
|
|
|
'schooladdr' => array(
|
|
'name' => 'School -- Full Address',
|
|
'header' => 'School Address',
|
|
'width' => 76.2 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "CONCAT(schools.address, ', ', schools.city, ', ', schools.province_code, ', ', schools.postalcode)" ),
|
|
|
|
/*
|
|
'teacher' => array(
|
|
'name' => 'School -- Teacher Name (as entered by the student)',
|
|
'header' => 'Teacher',
|
|
'width' => 38.1 ,
|
|
'table' => 'students.teachername' ),
|
|
|
|
'teacheremail' => array(
|
|
'name' => 'School -- Teacher Email (as entered by the student)',
|
|
'header' => 'Teacher Email',
|
|
'width' => 50.8 ,
|
|
'table' => 'students.teacheremail' ),
|
|
*/
|
|
'school_phone' => array(
|
|
'name' => 'School -- Phone',
|
|
'header' => 'School Phone',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => 'schools.phone' ),
|
|
|
|
'school_fax' => array(
|
|
'name' => 'School -- Fax',
|
|
'header' => 'School Fax',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => 'schools.fax' ),
|
|
|
|
|
|
'school_address' => array(
|
|
'name' => 'School -- Street Address',
|
|
'header' => 'Address',
|
|
'width' => 50.8 /*mm*/,
|
|
'table' => 'schools.address'),
|
|
|
|
'school_city' => array(
|
|
'name' => 'School -- City',
|
|
'header' => 'City',
|
|
'width' => 38.1 /*mm*/,
|
|
'table' => 'schools.city' ),
|
|
|
|
'school_province' => array(
|
|
'name' => 'School -- '.$config['provincestate'],
|
|
'header' => $config['provincestate'],
|
|
'width' => 19.05 /*mm*/,
|
|
'table' => 'schools.province_code' ),
|
|
|
|
'school_city_prov' => array(
|
|
'name' => 'School -- City, '.$config['provincestate'].' (for mailing)',
|
|
'header' => 'City',
|
|
'width' => 38.1 /*mm*/,
|
|
'table' => "CONCAT(schools.city, ', ', schools.province_code)" ),
|
|
|
|
'school_postal' => array(
|
|
'name' => 'School -- '.$config['postalzip'],
|
|
'header' => $config['postalzip'],
|
|
'width' => 19.05 /*mm*/,
|
|
'table' => 'schools.postalcode' ),
|
|
|
|
'school_principal' => array(
|
|
'name' => 'School -- Principal',
|
|
'header' => 'Principal',
|
|
'width' => 31.75 /*mm*/,
|
|
'table' => 'schools.principal_uid',
|
|
'exec_function' => 'reports_students_school_principal'),
|
|
|
|
'school_board' => array(
|
|
'name' => 'School -- Board ID',
|
|
'header' => 'Board',
|
|
'width' => 19.05 /*mm*/,
|
|
'table' => 'schools.board' ),
|
|
|
|
'awards' => array(
|
|
'start_option_group' => 'Awards assigned to student (warning: duplicates student entries for multiple awards won!)',
|
|
'name' => 'Award -- Type + Name',
|
|
'header' => 'Award Name',
|
|
'width' => 101.6 /*mm*/,
|
|
'table' => "CONCAT(IF(award_types.type='Other','Special',award_types.type),' ', award_awards.name)",
|
|
'table_sort' => 'award_awards.name',
|
|
'components' => array('awards')),
|
|
|
|
'award_name' => array(
|
|
'name' => 'Award -- Name',
|
|
'header' => 'Award Name',
|
|
'width' => 101.6 /*mm*/,
|
|
'table' => 'award_awards.name',
|
|
'components' => array('awards')),
|
|
|
|
'award_excludefromac' => array(
|
|
'name' => 'Award -- Exclude from Award Ceremony (Yes/No)',
|
|
'header' => 'Exclude',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => "award_awards.excludefromac",
|
|
'value_map' => array ('no' => 'No', 'yes' => 'Yes')),
|
|
|
|
'order' => array(
|
|
'name' => 'Award -- Order',
|
|
'header' => 'Award Order',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => 'award_awards.order',
|
|
'table_sort' => 'award_awards.order',
|
|
'components' => array('awards')),
|
|
|
|
'award_type' => array(
|
|
'name' => 'Award -- Type (Divisional, Special, etc.)',
|
|
'header' => 'Award Type',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => 'award_types.type',
|
|
'components' => array('awards')),
|
|
|
|
'sponsor' => array(
|
|
'name' => 'Award -- Sponsor DB ID',
|
|
'header' => 'Award Sponsor',
|
|
'width' => 38.1 /*mm*/,
|
|
'table' => 'award_awards.sponsors_id',
|
|
'table_sort' => 'award_awards.sponsors_id',
|
|
'components' => array('awards')),
|
|
|
|
'pn_awards' => array(
|
|
'name' => 'Award -- Project Num + Award Name (will be unique for each award)',
|
|
'header' => 'Award Name',
|
|
'width' => 101.6 /*mm*/,
|
|
'table' => "CONCAT(projects.projectnumber,' ', award_awards.name)",
|
|
'table_sort' => 'award_awards.order',
|
|
'components' => array('awards')),
|
|
|
|
'award_prize_name' => array(
|
|
'name' => 'Award -- Prize Name',
|
|
'header' => 'Prize Name',
|
|
'width' => 50.8 /*mm*/,
|
|
'table' => 'award_prizes.prize',
|
|
'components' => array('awards')),
|
|
|
|
'award_prize_cash' => array(
|
|
'name' => 'Award -- Prize Cash Amount',
|
|
'header' => 'Cash',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => 'award_prizes.cash',
|
|
'components' => array('awards')),
|
|
|
|
'award_prize_scholarship' => array(
|
|
'name' => 'Award -- Prize Scholarship Amount',
|
|
'header' => 'Scholarship',
|
|
'width' => 19.05 /*mm*/,
|
|
'table' => 'award_prizes.scholarship',
|
|
'components' => array('awards')),
|
|
|
|
'award_prize_value' => array(
|
|
'name' => 'Award -- Prize Value Amount',
|
|
'header' => 'Value',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => 'award_prizes.value',
|
|
'components' => array('awards')),
|
|
|
|
'award_prize_fullname' => array(
|
|
'name' => 'Award -- Prize Name, Category, Division',
|
|
'header' => 'Prize Name',
|
|
'width' => 101.6 /*mm*/,
|
|
'table' => "CONCAT(award_prizes.prize,' in ',projectcategories.category,' ', projectdivisions.division)",
|
|
'table_sort' => 'award_prizes.order',
|
|
'components' => array('awards')),
|
|
|
|
'award_prize_trophy_any' => array(
|
|
'name' => 'Award -- Trophy (\'Yes\' if the award has a trophy)',
|
|
'header' => 'Trophy',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => "IF ( award_prizes.trophystudentkeeper=1
|
|
OR award_prizes.trophystudentreturn=1
|
|
OR award_prizes.trophyschoolkeeper=1
|
|
OR award_prizes.trophyschoolreturn=1, 'Yes', 'No')",
|
|
'components' => array('awards')),
|
|
|
|
'award_prize_trophy_return' => array(
|
|
'name' => 'Award -- Annual Trophy (\'Yes\' if the award has a school or student trophy that isn\'t a keeper)',
|
|
'header' => 'Trophy',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => "IF ( award_prizes.trophystudentreturn=1
|
|
OR award_prizes.trophyschoolreturn=1, 'Yes', 'No')",
|
|
'components' => array('awards')),
|
|
|
|
'award_prize_trophy_return_student' => array(
|
|
'name' => 'Award -- Annual Student Trophy (\'Yes\' if the award has astudent trophy that isn\'t a keeper)',
|
|
'header' => 'Ind.',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => "IF ( award_prizes.trophystudentreturn=1, 'Yes', 'No')",
|
|
'components' => array('awards')),
|
|
|
|
'award_prize_trophy_return_school' => array(
|
|
'name' => 'Award -- Annual School Trophy (\'Yes\' if the award has a school trophy that isn\'t a keeper)',
|
|
'header' => 'Sch.',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => "IF ( award_prizes.trophyschoolreturn=1, 'Yes', 'No')",
|
|
'components' => array('awards')),
|
|
|
|
'nom_awards' => array(
|
|
'start_option_group' => 'Nominated Awards (warning: duplicates student for multiple awards!)',
|
|
'name' => 'Award Nominations -- Award Name',
|
|
'header' => 'Award Name',
|
|
'width' => 101.6 /*mm*/,
|
|
'table' => "CONCAT(award_types.type,' -- ',award_awards.name)",
|
|
'table_sort' => 'award_awards.name',
|
|
'components' => array('awards_nominations')),
|
|
|
|
'nom_pn_awards' => array(
|
|
'name' => 'Award Nominations -- Project Num + Award Name(will be unique)',
|
|
'header' => 'Award Name',
|
|
'width' => 101.6 /*mm*/,
|
|
'table' => "CONCAT(projects.projectnumber,' ', award_awards.name)",
|
|
'table_sort' => 'award_awards.name',
|
|
'components' => array('awards_nominations')),
|
|
|
|
'nom_awards_name_1' => array(
|
|
'name' => 'Award Nominations -- Self-Nominated Special Award 1',
|
|
'header' => 'Award Name',
|
|
'width' => 76.2 /*mm*/,
|
|
'table' => 'projects.id',
|
|
'table_sort' => 'projects.id',
|
|
'exec_function' => 'reports_students_award_selfnom_1'),
|
|
|
|
'nom_awards_name_2' => array(
|
|
'name' => 'Award Nominations -- Self-Nominated Special Award 2',
|
|
'header' => 'Award Name',
|
|
'width' => 76.2 /*mm*/,
|
|
'table' => 'projects.id',
|
|
'table_sort' => 'projects.id',
|
|
'exec_function' => 'reports_students_award_selfnom_2'),
|
|
|
|
'nom_awards_name_3' => array(
|
|
'name' => 'Award Nominations -- Self-Nominated Special Award 3',
|
|
'header' => 'Award Name',
|
|
'width' => 76.2 /*mm*/,
|
|
'table' => 'projects.id',
|
|
'table_sort' => 'projects.id',
|
|
'exec_function' => 'reports_students_award_selfnom_3'),
|
|
|
|
'nom_awards_name_4' => array(
|
|
'name' => 'Award Nominations -- Self-Nominated Special Award 4',
|
|
'header' => 'Award Name',
|
|
'width' => 76.2 /*mm*/,
|
|
'table' => 'projects.id',
|
|
'table_sort' => 'projects.id',
|
|
'exec_function' => 'reports_students_award_selfnom_4'),
|
|
|
|
'nom_awards_name_5' => array(
|
|
'name' => 'Award Nominations -- Self-Nominated Special Award 5',
|
|
'header' => 'Award Name',
|
|
'width' => 76.2 /*mm*/,
|
|
'table' => 'projects.id',
|
|
'table_sort' => 'projects.id',
|
|
'exec_function' => 'reports_students_award_selfnom_5'),
|
|
|
|
/* Emergency Contact Info */
|
|
'emerg_name' => array(
|
|
'start_option_group' => 'Emergency Contact Information',
|
|
'name' => 'Emergency Contact -- Name',
|
|
'header' => 'Contact Name',
|
|
'width' => 38.1 /*mm*/,
|
|
'table' => "CONCAT(emergencycontact.firstname, ' ', emergencycontact.lastname)",
|
|
'components' => array('emergencycontacts')),
|
|
|
|
'emerg_relation' => array(
|
|
'name' => 'Emergency Contact -- Relationship',
|
|
'header' => 'Relation',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => "emergencycontact.relation",
|
|
'components' => array('emergencycontacts')),
|
|
|
|
'emerg_phone' => array(
|
|
'name' => 'Emergency Contact -- Phone',
|
|
'header' => 'Emrg.Phone',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => "CONCAT(emergencycontact.phone1, ' ', emergencycontact.phone2, ' ', emergencycontact.phone3, ' ', emergencycontact.phone4)",
|
|
'components' => array('emergencycontacts')),
|
|
|
|
/* Tour Information */
|
|
'tour_assign_name' => array(
|
|
'start_option_group' => 'Tour Information',
|
|
'name' => 'Tours -- Assigned Tour Name',
|
|
'header' => 'Tour',
|
|
'width' => 101.6 /*mm*/,
|
|
'table' => "tours.name",
|
|
'components' => array('tours')),
|
|
|
|
'tour_assign_num' => array(
|
|
'name' => 'Tours -- Assigned Tour Number',
|
|
'header' => 'Tour',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => "tours.num",
|
|
'components' => array('tours')),
|
|
|
|
'tour_assign_numname' => array(
|
|
'name' => 'Tours -- Assigned Tour Number and Name',
|
|
'header' => 'Tour',
|
|
'width' => 101.6 /*mm*/,
|
|
'table' => "CONCAT(tours.num,': ', tours.name)",
|
|
'table_sort' => 'tours.num',
|
|
'components' => array('tours')),
|
|
|
|
/* Mentor Information */
|
|
'mentor_name_proj' => array(
|
|
'start_option_group' => 'Mentor Information',
|
|
'name' => 'Mentor -- Project and Name (Distinct for each Project+Mentor pair)',
|
|
'header' => 'Mentor Name',
|
|
'width' => 44.45 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "CONCAT('projects.projectnumber', ' - ', mentors.firstname, ', ', mentors.lastname)",
|
|
'table_sort'=> 'mentors.lastname',
|
|
'components' => array('mentors')),
|
|
|
|
'mentor_last_name' => array(
|
|
'name' => 'Mentor -- Last Name',
|
|
'header' => 'Last Name',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => 'mentors.lastname',
|
|
'components' => array('mentors')),
|
|
|
|
'mentor_first_name' => array(
|
|
'name' => 'Mentor -- First Name',
|
|
'header' => 'First Name',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => 'mentors.firstname',
|
|
'components' => array('mentors')),
|
|
|
|
'mentor_name' => array(
|
|
'name' => 'Mentor -- Full Name (last, first)',
|
|
'header' => 'Mentor Name',
|
|
'width' => 44.45 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "CONCAT(mentors.lastname, ', ', mentors.firstname)",
|
|
'table_sort'=> 'mentors.lastname',
|
|
'components' => array('mentors')),
|
|
|
|
'mentor_namefl' => array(
|
|
'name' => 'Mentor -- Full Name (first last)',
|
|
'header' => 'Mentor Name',
|
|
'width' => 44.45 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => "CONCAT(mentors.firstname, ' ', mentors.lastname)",
|
|
'table_sort'=> 'mentors.lastname',
|
|
'components' => array('mentors')),
|
|
|
|
'mentor_email' => array(
|
|
'name' => 'Mentor -- Email',
|
|
'header' => 'Mentor Email',
|
|
'width' => 50.8 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'mentors.email',
|
|
'components' => array('mentors')),
|
|
|
|
'mentor_phone' => array(
|
|
'name' => 'Mentor -- Phone',
|
|
'header' => 'Mentor Phone',
|
|
'width' => 25.4 /*mm*/,
|
|
'table' => 'mentors.phone',
|
|
'components' => array('mentors')),
|
|
|
|
'mentor_organization' => array(
|
|
'name' => 'Mentor -- Organization',
|
|
'header' => 'Mentor Org.',
|
|
'width' => 38.1 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'mentors.organization',
|
|
'components' => array('mentors')),
|
|
|
|
'mentor_position' => array(
|
|
'name' => 'Mentor -- Position',
|
|
'header' => 'Position',
|
|
'width' => 25.4 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'mentors.position',
|
|
'components' => array('mentors')),
|
|
|
|
'mentor_description' => array(
|
|
'name' => 'Mentor -- Description of Help',
|
|
'header' => 'Description of Help',
|
|
'width' => 76.2 /*mm*/,
|
|
'scalable' => true,
|
|
'table' => 'mentors.description',
|
|
'components' => array('mentors')),
|
|
|
|
/* Fair Information */
|
|
'feeder_fair_name' => array (
|
|
'start_option_group' => 'Fair Information',
|
|
'name' => 'Feeder Fair -- Name',
|
|
'header' => 'Fair Name',
|
|
'width' => 38.1 /*mm*/,
|
|
'table' => 'fairs.name',
|
|
'components' => array('fairs')),
|
|
|
|
'feeder_fair_abbrv' => array (
|
|
'name' => 'Feeder Fair -- Abbreviation',
|
|
'header' => 'Fair',
|
|
'width' => 19.05 /*mm*/,
|
|
'table' => 'fairs.abbrv',
|
|
'components' => array('fairs')),
|
|
|
|
'conference_name' => array (
|
|
'name' => 'Conference -- Name',
|
|
'header' => 'Conference Name',
|
|
'width' => 76.2 /*mm*/,
|
|
'table' => "'".mysql_real_escape_string($conference['name'])."'"),
|
|
|
|
'conference_logo' => array(
|
|
'name' => 'Conference -- Logo (for Labels only)',
|
|
'header' => '',
|
|
'width' => 1 /*mm*/,
|
|
'table' => "CONCAT(' ')"),
|
|
|
|
/* Special/Misc/Other */
|
|
'static_text' => array (
|
|
'start_option_group' => 'Special Fields',
|
|
'name' => 'Label -- Static Text',
|
|
'header' => '',
|
|
'width' => 2.54 /*mm*/,
|
|
'table' => "CONCAT(' ')"),
|
|
|
|
'static_box' => array (
|
|
'name' => 'Label -- Static Box',
|
|
'header' => '',
|
|
'width' => 2.54 /*mm*/,
|
|
'table' => "CONCAT(' ')"),
|
|
|
|
'easyparse_allnames' => array(
|
|
'name' => "Easy Parse -- All Student Names (REQUIRES MYSQL 5.0) ",
|
|
'header' => 'Student(s)',
|
|
'width' => 76.2 /*mm*/,
|
|
'table' => "GROUP_CONCAT(users.lastname, ',', users.firstname ORDER BY users.lastname SEPARATOR ':')",
|
|
'group_by' => array('users.registrations_id')),
|
|
|
|
'special_tshirt_count' => array(
|
|
'name' => 'Special -- T-Shirt Size Count',
|
|
'header' => 'Count',
|
|
'width' => 12.7 /*mm*/,
|
|
'table' => 'COUNT(*)',
|
|
'total' => true,
|
|
'group_by' => array('users.tshirt')),
|
|
|
|
'safetyquestion_1' => array(
|
|
'start_option_group' => 'Project Safety Questions',
|
|
'name' => 'Project Safety -- Safety Question 1',
|
|
'header' => 'Q1',
|
|
'width' => 15 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'report_student_safety_question'),
|
|
|
|
'safetyquestion_2' => array(
|
|
'name' => 'Project Safety -- Safety Question 2',
|
|
'header' => 'Q2',
|
|
'width' => 15 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'report_student_safety_question'),
|
|
|
|
'safetyquestion_3' => array(
|
|
'name' => 'Project Safety -- Safety Question 3',
|
|
'header' => 'Q3',
|
|
'width' => 15 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'report_student_safety_question'),
|
|
|
|
'safetyquestion_4' => array(
|
|
'name' => 'Project Safety -- Safety Question 4',
|
|
'header' => 'Q4',
|
|
'width' => 15 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'report_student_safety_question'),
|
|
|
|
'safetyquestion_5' => array(
|
|
'name' => 'Project Safety -- Safety Question 5',
|
|
'header' => 'Q5',
|
|
'width' => 15 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'report_student_safety_question'),
|
|
|
|
'safetyquestion_6' => array(
|
|
'name' => 'Project Safety -- Safety Question 6',
|
|
'header' => 'Q6',
|
|
'width' => 15 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'report_student_safety_question'),
|
|
|
|
'safetyquestion_7' => array(
|
|
'name' => 'Project Safety -- Safety Question 7',
|
|
'header' => 'Q7',
|
|
'width' => 15 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'report_student_safety_question'),
|
|
|
|
'safetyquestion_8' => array(
|
|
'name' => 'Project Safety -- Safety Question 8',
|
|
'header' => 'Q8',
|
|
'width' => 15 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'report_student_safety_question'),
|
|
|
|
'safetyquestion_9' => array(
|
|
'name' => 'Project Safety -- Safety Question 9',
|
|
'header' => 'Q9',
|
|
'width' => 15 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'report_student_safety_question'),
|
|
|
|
'safetyquestion_10' => array(
|
|
'name' => 'Project Safety -- Safety Question 10',
|
|
'header' => 'Q10',
|
|
'width' => 15 /*mm*/,
|
|
'table' => 'users.registrations_id',
|
|
'exec_function' => 'report_student_safety_question'),
|
|
|
|
|
|
);
|
|
|
|
function report_students_fromwhere($report, $components)
|
|
{
|
|
global $config, $report_students_fields;
|
|
|
|
$fields = $report_students_fields;
|
|
$conferences_id = $report['conferences_id'];
|
|
|
|
$awards_join = '';
|
|
$awards_where = '';
|
|
|
|
if(in_array('awards', $components)) {
|
|
/* This requires some extra gymnastics and will duplicate
|
|
* students/projects if they have won multiple awards */
|
|
$awards_join = "LEFT JOIN winners ON winners.projects_id = projects.id
|
|
LEFT JOIN award_prizes ON award_prizes.id = winners.awards_prizes_id
|
|
LEFT JOIN award_awards ON award_awards.id = award_prizes.award_awards_id
|
|
LEFT JOIN award_types ON award_types.id=award_awards.award_types_id";
|
|
$awards_where = " AND winners.conferences_id='$conferences_id'
|
|
AND award_awards.conferences_id='$conferences_id'
|
|
AND award_prizes.conferences_id='$conferences_id'
|
|
AND award_types.conferences_id='$conferences_id' ";
|
|
}
|
|
|
|
if(in_array('awards_nominations', $components)) {
|
|
$awards_join = "LEFT JOIN project_specialawards_link
|
|
ON(projects.id=project_specialawards_link.projects_id),
|
|
award_awards,award_types";
|
|
$awards_where = " AND project_specialawards_link.award_awards_id=award_awards.id
|
|
AND award_types.id=award_awards.award_types_id
|
|
AND award_awards.conferences_id='$conferences_id'
|
|
AND award_types.conferences_id='$conferences_id' ";
|
|
}
|
|
|
|
$partner_join = '';
|
|
if(in_array('partner', $components)) {
|
|
$partner_join = "LEFT JOIN users AS students2
|
|
ON(students2.registrations_id=users.registrations_id
|
|
AND students2.id != users.id)";
|
|
}
|
|
|
|
$tour_join = '';
|
|
$tour_where = '';
|
|
if(in_array('tours', $components)) {
|
|
$tour_join = "LEFT JOIN tours_choice ON (users.id=tours_choice.students_id AND tours_choice.rank=0), tours";
|
|
$tour_where = "AND tours.conferences_id='$conferences_id'
|
|
AND tours.id=tours_choice.tour_id";
|
|
}
|
|
|
|
$emergencycontact_join = '';
|
|
if(in_array('emergencycontacts', $components)) {
|
|
/* No need to put the year in here, students.id is unique across years */
|
|
$emergencycontact_join = "LEFT JOIN emergencycontact ON
|
|
emergencycontact.users_id=users.id ";
|
|
}
|
|
|
|
$mentor_join = '';
|
|
$mentor_where = '';
|
|
if(in_array('mentors', $components)) {
|
|
$mentor_join = "LEFT JOIN mentors ON
|
|
mentors.registrations_id=users.registrations_id";
|
|
$mentor_where = "AND mentors.conferences_id='$conferences_id'";
|
|
}
|
|
|
|
$fairs_join = '';
|
|
if(in_array('fairs', $components)) {
|
|
$fairs_join = "LEFT JOIN fairs ON fairs.id=projects.fairs_id";
|
|
}
|
|
|
|
if($report['option']['include_incomplete_registrations'] == 'yes')
|
|
$reg_where = '';
|
|
else
|
|
$reg_where = "AND (registrations.status='complete' OR registrations.status='paymentpending')";
|
|
|
|
|
|
$q = " FROM users
|
|
JOIN user_roles ON users.id=user_roles.users_id
|
|
JOIN roles ON user_roles.roles_id=roles.id
|
|
JOIN accounts ON users.accounts_id=accounts.id
|
|
LEFT JOIN registrations ON registrations.id=users.registrations_id
|
|
LEFT JOIN schools ON schools.id=users.schools_id
|
|
LEFT JOIN projects ON projects.registrations_id=users.registrations_id
|
|
LEFT JOIN projectdivisions ON projectdivisions.id=projects.projectdivisions_id
|
|
LEFT JOIN projectcategories ON projectcategories.id=projects.projectcategories_id
|
|
$emergencycontact_join
|
|
$partner_join
|
|
$mentor_join
|
|
$tour_join
|
|
$awards_join
|
|
$fairs_join
|
|
WHERE
|
|
users.conferences_id='$conferences_id'
|
|
AND (projects.conferences_id='$conferences_id' OR projects.conferences_id IS NULL)
|
|
AND (registrations.conferences_id='$conferences_id' OR registrations.conferences_id IS NULL)
|
|
AND (projectcategories.conferences_id='$conferences_id' OR projectcategories.conferences_id IS NULL)
|
|
AND (projectdivisions.conferences_id='$conferences_id' OR projectdivisions.conferences_id IS NULL)
|
|
AND roles.type='participant'
|
|
$reg_where
|
|
$mentor_where
|
|
$awards_where
|
|
$tour_where
|
|
";
|
|
|
|
return $q;
|
|
}
|
|
|
|
?>
|