forked from science-ation/science-ation
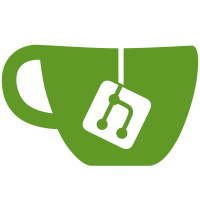
- Put a total at the top of the judge manager list - Add to the table editor: - Change the filter to accept a single argument, so we're not restricted to the `$k`='$v' syntax, we want to be able to filter on a table JOIN. - Add a additionalListTable variable, and additionalListTable() function, to specify additional tables that should be part of the table select statement. I guess ideally the class would implmenent their own if alternative behaviour was desired. But this way is pretty generic. The table editor can now, for example, select judges by year.
295 lines
7.3 KiB
PHP
295 lines
7.3 KiB
PHP
<?
|
|
|
|
/* Just the fields in the judges table, we use this twice */
|
|
$judges_fields = array( 'firstname' => 'First Name',
|
|
'lastname' => 'Last Name',
|
|
'email' => 'Email Address',
|
|
'password' => 'Password',
|
|
'passwordexpiry' => 'Password Expiry',
|
|
'phonehome' => 'Phone (Home)',
|
|
'phonecell' => 'Phone (Cell)',
|
|
'phonework' => 'Phone (Work)',
|
|
'phoneworkext' => 'Phone Ext. (Work)',
|
|
'organization' => 'Organization',
|
|
'created' => 'Created',
|
|
'lastlogin' => 'Last Login',
|
|
'address' =>"Address 1",
|
|
'address2' =>"Address 2",
|
|
'city' => 'City',
|
|
'province' => 'Province',
|
|
'postalcode' => 'Postal Code',
|
|
'deleted' => 'Deleted',
|
|
'deleteddatetime' => 'Deleted Date/Time',
|
|
'expertise_other' => 'Other Expertise/Notes',
|
|
'complete' => "Complete");
|
|
|
|
|
|
class person {
|
|
var $id;
|
|
|
|
function person($person_id=NULL)
|
|
{
|
|
if($person_id == NULL) {
|
|
print("Empty constructor called\n");
|
|
$this->id = FALSE;
|
|
} else {
|
|
print("ID $person_id construction called\n");
|
|
$this->id = $person_id;
|
|
}
|
|
}
|
|
};
|
|
|
|
|
|
class judge extends person {
|
|
|
|
/* Static members for the table editor */
|
|
function tableEditorSetup($editor)
|
|
{
|
|
global $judges_fields;
|
|
global $config;
|
|
|
|
$cat = array();
|
|
$catf = array();
|
|
$q=mysql_query("SELECT * FROM projectcategories WHERE year='".$config['FAIRYEAR']."' ORDER BY id");
|
|
while($r=mysql_fetch_object($q)) {
|
|
$cat[$r->id]=$r->category;
|
|
$catf["catpref_{$r->id}"] = "Category Pref|{$r->category}:";
|
|
}
|
|
|
|
$div = array();
|
|
$diff = array();
|
|
$q=mysql_query("SELECT * FROM projectdivisions WHERE year='".$config['FAIRYEAR']."' ORDER BY id");
|
|
while($r=mysql_fetch_object($q)) {
|
|
// $divshort[$r->id]=$r->division_shortform;
|
|
$div[$r->id]=$r->division;
|
|
/* yes, catf */
|
|
$catf["divpref_{$r->id}"] = "Expertise|{$r->division}:";
|
|
}
|
|
|
|
|
|
|
|
/* Setup the table editor with the fields we want to display
|
|
* when displaying a list of judges, and also the type of each
|
|
* field where required */
|
|
$l = array( 'id' => 'ID',
|
|
'firstname' => 'First Name',
|
|
'lastname' => 'Last Name',
|
|
'complete' => 'Complete'
|
|
|
|
);
|
|
|
|
/* Most of these should be moved to the base class, as they
|
|
* will be the same for all person groups */
|
|
$e = array_merge($judges_fields,
|
|
array( 'language' => 'Language(s)',
|
|
));
|
|
|
|
$e = array_merge($e, $catf);
|
|
|
|
$editor->setTable('judges');
|
|
$editor->setListFields($l);
|
|
$editor->setEditFields($e);
|
|
|
|
// print_r($e);
|
|
print("<br>\n");
|
|
/* Build an array of langauges that we support */
|
|
$langs = array();
|
|
$q=mysql_query("SELECT * FROM languages WHERE active='Y'");
|
|
while($r=mysql_fetch_object($q)) {
|
|
$langs[$r->lang] = $r->langname;
|
|
}
|
|
$editor->setFieldOptions('language', $langs);
|
|
$editor->setFieldInputType('language', 'multicheck');
|
|
|
|
|
|
/* Pulled these out of register_judges.inc.php */
|
|
$preferencechoices=array(
|
|
array('key' => -2, 'val' => "Very Low"),
|
|
array('key' => -1, 'val' => "Low"),
|
|
array('key' => 0, 'val' => "Indifferent"),
|
|
array('key' => 1, 'val' => "Medium"),
|
|
array('key' => 2, 'val' => "High") );
|
|
|
|
foreach($cat as $cid=>$category) {
|
|
$editor->setFieldOptions("catpref_$cid", $preferencechoices);
|
|
$editor->setFieldInputType("catpref_$cid", 'select');
|
|
}
|
|
$expertisechoices=array(
|
|
array('key' => 1, 'val' => "(1) Low"),
|
|
array('key' => 2, 'val' => "(2) Med-Low"),
|
|
array('key' => 3, 'val' => "(3) Medium"),
|
|
array('key' => 4, 'val' => "(4) Med-High"),
|
|
array('key' => 5, 'val' => "(5) High") );
|
|
|
|
foreach($div as $did=>$division) {
|
|
$editor->setFieldOptions("divpref_$did", $expertisechoices);
|
|
$editor->setFieldInputType("divpref_$did", 'select');
|
|
}
|
|
}
|
|
|
|
/* Functions for $this */
|
|
|
|
|
|
function judge($judge_id=NULL)
|
|
{
|
|
global $judges_fields;
|
|
person::person($judge_id);
|
|
}
|
|
|
|
function tableEditorLoad()
|
|
{
|
|
global $config;
|
|
|
|
$id = $this->id;
|
|
|
|
print("Loading Judge ID $id\n");
|
|
|
|
$q=mysql_query("SELECT judges.*
|
|
FROM judges
|
|
WHERE judges.id='$id'");
|
|
echo mysql_error();
|
|
|
|
|
|
/* We assume that the field names in the array we want to return
|
|
* are the same as those in the database, so we'll turn the entire
|
|
* query into a single associative array */
|
|
$j = mysql_fetch_assoc($q);
|
|
|
|
/* Now turn on the ones this judge has selected */
|
|
$q=mysql_query("SELECT languages_lang
|
|
FROM judges_languages
|
|
WHERE judges_id='$id'");
|
|
$j['language'] = array();
|
|
if(mysql_num_rows($q)) {
|
|
while($r=mysql_fetch_object($q)) {
|
|
$j['language'][$r->languages_lang] = 1;
|
|
}
|
|
}
|
|
|
|
$q=mysql_query("SELECT *
|
|
FROM judges_catpref
|
|
WHERE judges_id='$id'
|
|
AND year='{$config['FAIRYEAR']}'");
|
|
if(mysql_num_rows($q)) {
|
|
while($r=mysql_fetch_object($q)) {
|
|
$j["catpref_{$r->projectcategories_id}"] = $r->rank;
|
|
}
|
|
}
|
|
$q=mysql_query("SELECT *
|
|
FROM judges_expertise
|
|
WHERE judges_id='$id'
|
|
AND year='{$config['FAIRYEAR']}'");
|
|
if(mysql_num_rows($q)) {
|
|
while($r=mysql_fetch_object($q)) {
|
|
$j["divpref_{$r->projectdivisions_id}"] = $r->val;
|
|
}
|
|
}
|
|
|
|
// print_r($j);
|
|
|
|
return $j;
|
|
}
|
|
|
|
function tableEditorSave($data)
|
|
{
|
|
/* If $this->id == false, then we need to INSERT a new record.
|
|
* if it's a number, then we want an UPDATE statement */
|
|
global $judges_fields;
|
|
global $config;
|
|
|
|
$query = "";
|
|
|
|
/* Construct an insert query if we have to */
|
|
if($this->id == false) {
|
|
$query = "INSERT INTO judges (id) VALUES ('')";
|
|
mysql_query($query);
|
|
$this->id = mysql_insert_id();
|
|
}
|
|
|
|
/* Now just update the record */
|
|
$query="UPDATE `judges` SET ";
|
|
|
|
foreach($judges_fields AS $f=>$n) {
|
|
$n = $data[$f];
|
|
$query .= "`$f`=$n,";
|
|
}
|
|
//rip off the last comma
|
|
$query=substr($query,0,-1);
|
|
|
|
$query .= " WHERE id='{$this->id}'";
|
|
|
|
echo $query;
|
|
mysql_query($query);
|
|
|
|
|
|
/* judges_languages */
|
|
/* First delete all the languages, then insert the ones the judge
|
|
* has selected */
|
|
$query = "DELETE FROM judges_languages WHERE judges_id='{$this->id}'";
|
|
mysql_query($query);
|
|
|
|
// print_r($data['language']);
|
|
$keys = array_keys($data['language']);
|
|
foreach($keys as $k) {
|
|
$query = "INSERT INTO
|
|
judges_languages (judges_id,languages_lang)
|
|
VALUES ('{$this->id}','$k')";
|
|
print("$query");
|
|
mysql_query($query);
|
|
}
|
|
|
|
/* judges_catpref */
|
|
$query = "DELETE FROM judges_catpref WHERE judges_id='{$this->id}'";
|
|
// print($query);
|
|
mysql_query($query);
|
|
|
|
/* Find all the catpref_[number] keys */
|
|
$keys = array_keys($data);
|
|
foreach($keys as $k) {
|
|
if(ereg("^catpref_([0-9]*)$", $k, $regs)) {
|
|
if($data[$k] == "''") continue;
|
|
$query = "INSERT INTO judges_catpref
|
|
(judges_id,projectcategories_id,rank,year)
|
|
values ('{$this->id}','{$regs[1]}',{$data[$k]},'{$config['FAIRYEAR']}')";
|
|
// print($query."<br>\n");
|
|
mysql_query($query);
|
|
|
|
}
|
|
}
|
|
/* Find all the divpref_[number] keys */
|
|
$query = "DELETE FROM judges_expertise WHERE judges_id='{$this->id}'";
|
|
// print($query);
|
|
mysql_query($query);
|
|
$keys = array_keys($data);
|
|
foreach($keys as $k) {
|
|
if(ereg("^divpref_([0-9]*)$", $k, $regs)) {
|
|
if($data[$k] == "''") continue;
|
|
$query = "INSERT INTO judges_expertise
|
|
(judges_id,projectdivisions_id,val,year)
|
|
values ('{$this->id}','{$regs[1]}',{$data[$k]},'{$config['FAIRYEAR']}')";
|
|
print($query."<br>\n");
|
|
mysql_query($query);
|
|
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
function tableEditorDelete()
|
|
{
|
|
global $config;
|
|
|
|
$id = $this->id;
|
|
|
|
mysql_query("DELETE FROM judges_teams_link WHERE judges_id='$id' AND year=".$config['FAIRYEAR']."'");
|
|
mysql_query("DELETE FROM judges_years WHERE judges_id='$id' AND year='".$config['FAIRYEAR']."'");
|
|
|
|
echo happy(i18n("Successfully removed judge from this year's fair"));
|
|
}
|
|
|
|
|
|
|
|
};
|
|
|
|
?>
|