forked from science-ation/science-ation
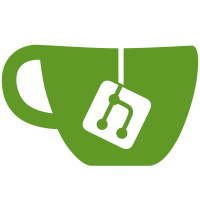
Added compvars.php, which is a test script for comparing data after it's been rolled over from one conference to another - used to test the rollover in super/conferences.php. Updated usage of the role "student", replacing it with "participant".
266 lines
7.8 KiB
PHP
266 lines
7.8 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
Copyright (C) 2007 David Grant <dave@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require_once('common.inc.php');
|
|
require_once('account.inc.php');
|
|
require_once('user.inc.php');
|
|
|
|
/* Don't do any login stuff if they're already logged in */
|
|
if(isset($_SESSION['accounts_id'])) {
|
|
/* They're already logged in, if they're not trying to logout, don't
|
|
* let them see the login page */
|
|
if($_GET['action'] != 'logout' && $_GET['action']!='switchconference') {
|
|
message_push(error(i18n('You are already logged in, please use the "Logout" link in the upper right to logout before logging in as different user')));
|
|
header("location: user_main.php");
|
|
exit;
|
|
}
|
|
}
|
|
|
|
$notice=$_GET['notice'];
|
|
|
|
$redirect = $_GET['redirect'];
|
|
$redirect_data = $_GET['redirectdata'];
|
|
|
|
switch($redirect) {
|
|
case 'roleadd':
|
|
$redirect_url = "&redirect=$redirect&redirectdata=$redirectdata";
|
|
break;
|
|
case 'roleattached':
|
|
$redirect_url = "&redirect=$redirect";
|
|
break;
|
|
default:
|
|
$redirect_url = '';
|
|
break;
|
|
}
|
|
|
|
/*
|
|
switch($role) {
|
|
case 'volunteer':
|
|
// returns "notopenyet", "closed", or "open"
|
|
$reg_open = user_volunteer_registration_status();
|
|
break;
|
|
case 'committee':
|
|
$reg_open = 'notpermitted';
|
|
break;
|
|
case 'judge':
|
|
$reg_open = user_judge_registration_status();
|
|
break;
|
|
case 'fair':
|
|
$reg_open = 'notpermitted';
|
|
break;
|
|
case 'sponsor':
|
|
$reg_open = 'notpermitted';
|
|
break;
|
|
case 'parent': case 'alumni': case 'principal': case 'mentor':
|
|
/* Always open, because they could have been auto-created
|
|
$reg_open = 'open';
|
|
break;
|
|
case 'participant':
|
|
default:
|
|
if($_GET['action']!="logout")
|
|
exit;
|
|
$reg_open = 'closed';
|
|
break;
|
|
}
|
|
*/
|
|
|
|
if($_POST['action']== 'login' ) {
|
|
|
|
$user = $_POST['username'];
|
|
$pass = $_POST['password'];
|
|
|
|
$accounts_id = try_login($user, $pass);
|
|
if($accounts_id == false) {
|
|
message_push(error(i18n("Invalid Email/Password")));
|
|
header("location: user_login.php");
|
|
exit;
|
|
}
|
|
|
|
$a = account_load($accounts_id);
|
|
$_SESSION['username']=$a['username'];
|
|
$_SESSION['email']=$a['email'];
|
|
$_SESSION['accounts_id']=$accounts_id;
|
|
$_SESSION['superuser'] = ($a['superuser'] == 'yes') ? 'yes' : 'no';
|
|
$_SESSION['roles']=array();
|
|
|
|
$val=null;
|
|
|
|
if($val=user_conference_load($accounts_id,$_SESSION['conferences_id'])) {
|
|
header("Location: $val");
|
|
}
|
|
else
|
|
header("Location: ".$config['SFIABDIRECTORY']."/index.php");
|
|
exit;
|
|
|
|
} else if($_GET['action']=="switchconference") {
|
|
//get rid of their current roles, and load their record for the new conference
|
|
if(is_array($_SESSION['roles'])) {
|
|
$_SESSION['roles']=array();
|
|
if($val=user_conference_load($_SESSION['accounts_id'],$_SESSION['conferences_id'])) {
|
|
header("Location: $val");
|
|
exit;
|
|
}
|
|
else {
|
|
header("Location: ".$config['SFIABDIRECTORY']."/index.php");
|
|
}
|
|
}
|
|
else {
|
|
header("Location: ".$config['SFIABDIRECTORY']."/index.php");
|
|
}
|
|
} else if($_GET['action']=='logout') {
|
|
/* Session keys to skip on logout */
|
|
$skip = array('debug', 'lang', 'messages');
|
|
|
|
/* Do these explicitly because i'm paranoid */
|
|
unset($_SESSION['name']);
|
|
unset($_SESSION['username']);
|
|
unset($_SESSION['email']);
|
|
unset($_SESSION['users_id']);
|
|
unset($_SESSION['accounts_id']);
|
|
unset($_SESSION['roles']);
|
|
unset($_SESSION['superuser']);
|
|
|
|
/* Take care of anything else */
|
|
$keys = array_diff(array_keys($_SESSION), $skip);
|
|
foreach($keys as $k) unset($_SESSION[$k]);
|
|
|
|
message_push(notice(i18n("You have been successfully logged out")));
|
|
header("Location: user_login.php{$redirect_url}");
|
|
exit;
|
|
|
|
} else if($_GET['action']=='recover') {
|
|
send_header("Password Recovery",
|
|
array("Login" => "user_login.php?role=$role"));
|
|
|
|
$recover_link = "user_login.php?role=$role&action=recover";
|
|
|
|
?>
|
|
<p><?=i18n('This form will have your password sent to the email address on your account. Please enter your email address below, and click on the \'Recover\' button. Sometimes the email takes a few minutes to send, so please be patient.')?></p>
|
|
<form method="post" action="user_login.php?role=<?=$role?>">
|
|
<input type="hidden" name="action" value="recoverconfirm" />
|
|
<?=i18n("Email")?>:</td><td><input type="text" size="20" name="email" />
|
|
<input type="submit" value="<?=i18n("Recover")?>" />
|
|
</form>
|
|
<div style="font-size: 0.75em; margin-top:2em">
|
|
<?=i18n('If you didn\'t register using an email address and you have lost your password, please contact the committee to have your password reset.')?></div><br />
|
|
<?
|
|
send_footer();
|
|
}
|
|
else if($_POST['action'] == "recoverconfirm")
|
|
{
|
|
/* Process a recover */
|
|
$email = $_POST['email'];
|
|
|
|
if(!isEmailAddress($email)) {
|
|
// not a valid email address
|
|
message_push(error(i18n("Email address error")));
|
|
header("Location: user_login.php");
|
|
exit;
|
|
}
|
|
|
|
$email = mysql_real_escape_string($email);
|
|
// let's see if we can find this email address on an account
|
|
$q = mysql_query("SELECT * FROM accounts WHERE email LIKE '$email'");
|
|
$r = mysql_fetch_object($q);
|
|
|
|
if(!$r){
|
|
// didn't find it that way. Let's try finding an unconfirmed e-mail
|
|
$q = mysql_query("SELECT * FROM accounts WHERE pendingemail LIKE '$email'");
|
|
$r = mysql_fetch_object($q);
|
|
}
|
|
|
|
if($r) {
|
|
|
|
// found the specified email address
|
|
/* volunteer_recover_password, judge_recover_password, student_recover_password,
|
|
committee_recover_password */
|
|
email_send("account_recover_password",
|
|
$email,
|
|
array("FAIRNAME"=>i18n($conference['name'])),
|
|
array( "PASSWORD"=>$r->password,
|
|
"USERNAME"=>$r->username)
|
|
);
|
|
|
|
message_push(notice(i18n("Your password has been sent to your email address")));
|
|
header("Location: user_login.php");
|
|
}else{
|
|
message_push(error(i18n("Could not find your email address for recovery")));
|
|
header("Location: user_login.php");
|
|
}
|
|
|
|
}
|
|
else
|
|
{
|
|
send_header("Login", array());
|
|
|
|
$recover_link = "user_login.php?role=$role&action=recover";
|
|
$new_link = "user_new.php?role=$role";
|
|
|
|
?>
|
|
<form method="post" action="user_login.php?role=<?="$role$redirect_url"?>">
|
|
<input type="hidden" name="action" value="login" />
|
|
<table><tr><td>
|
|
<?=i18n("Email")?>:</td><td><input type="text" size="20" name="username" />
|
|
</td></tr><tr><td>
|
|
<?=i18n("Password")?>:</td><td><input type="password" size="20" name="password" />
|
|
</td></tr>
|
|
<tr><td colspan=2>
|
|
<input type="submit" value=<?=i18n("Login")?> />
|
|
</td></tr>
|
|
</table>
|
|
</form>
|
|
|
|
<br />
|
|
<div style="font-size: 0.75em;">
|
|
<?=i18n("If you have lost or forgotten your password, or have misplaced the email with your initial password, please <a href=\"$recover_link\">click here to recover it</a>")?>.</div><br />
|
|
<br />
|
|
<?
|
|
switch($reg_open) {
|
|
case 'notopenyet':
|
|
echo i18n("Registration for %1 has not yet opened",
|
|
array($conference['name']),
|
|
array("Fair name")
|
|
);
|
|
break;
|
|
case 'open':
|
|
echo i18n("If you would like to register as a new {$roles[$role]['name']}, <a href=\"$new_link\">click here</a>.<br />");
|
|
break;
|
|
|
|
case 'closed':
|
|
echo i18n("Registration for the %1 is now closed",
|
|
array($conference['name']),
|
|
array("Fair name")
|
|
);
|
|
break;
|
|
case 'notpermitted':
|
|
default:
|
|
break;
|
|
}
|
|
|
|
send_footer();
|
|
}
|
|
?>
|
|
|