forked from science-ation/science-ation
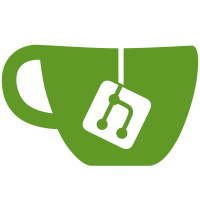
to just call the function - Rework the fair editor for user friendliness. - Beginnings of sfiab->sfiab award download (and winner upload)
213 lines
7.3 KiB
PHP
213 lines
7.3 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
Copyright (C) 2007 David Grant <dave@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require_once("common.inc.php");
|
|
require_once("user.inc.php");
|
|
require_once("fair.inc.php");
|
|
|
|
$fair_type = array('feeder' => 'Feeder Fair', 'sfiab' => 'SFIAB Upstream', 'ysf' => 'YSF/CWSF Upstream');
|
|
|
|
function yesno($name, $val)
|
|
{
|
|
echo "<select name=\"$name\">";
|
|
$sel = ($val == 'yes') ? 'selected="selected"' : '';
|
|
echo "<option $sel value=\"yes\">".i18n("Yes")."</option>";
|
|
$sel = ($val == 'no') ? 'selected="selected"' : '';
|
|
echo "<option $sel value=\"no\">".i18n("No")."</option>";
|
|
echo "</select>";
|
|
}
|
|
|
|
|
|
if($_SESSION['embed'] == true) {
|
|
$u = user_load($_SESSION['embed_edit_id']);
|
|
} else {
|
|
user_auth_required('fair');
|
|
$u = user_load($_SESSION['users_id']);
|
|
}
|
|
|
|
|
|
if($_POST['action']=="save")
|
|
{
|
|
$id = $_POST['id'];
|
|
if(trim($id) == '') {
|
|
$q = mysql_query("INSERT INTO fairs(`id`,`name`) VALUES('','new entry')");
|
|
$id = mysql_insert_id();
|
|
} else {
|
|
$id = intval($id);
|
|
}
|
|
|
|
$name = mysql_escape_string(stripslashes($_POST['name']));
|
|
$abbrv = mysql_escape_string(stripslashes($_POST['abbrv']));
|
|
$url = mysql_escape_string($_POST['url']);
|
|
$website = mysql_escape_string($_POST['website']);
|
|
$type = array_key_exists($_POST['type'], $fair_type) ? $_POST['type'] : '';
|
|
$username = mysql_escape_string(stripslashes($_POST['username']));
|
|
$password = mysql_escape_string(stripslashes($_POST['password']));
|
|
$enable_stats = ($_POST['enable_stats'] == 'yes') ? 'yes' : 'no';
|
|
$enable_awards = ($_POST['enable_awards'] == 'yes') ? 'yes' : 'no';
|
|
$enable_winners = ($_POST['enable_winners'] == 'yes') ? 'yes' : 'no';
|
|
|
|
$q = mysql_query("UPDATE fairs SET `name`='$name',
|
|
`abbrv`='$abbrv', `url`='$url',
|
|
`website`='$website',
|
|
`type`='$type' , `username`='$username',
|
|
`password`='$password',
|
|
`enable_stats`='$enable_stats',
|
|
`enable_awards`='$enable_awards',
|
|
`enable_winners`='$enable_winners'
|
|
WHERE id=$id");
|
|
|
|
$u['fairs_id'] = $id;
|
|
user_save($u);
|
|
message_push(notice(i18n("Fair Informaiton successfully updated")));
|
|
}
|
|
|
|
/* update overall status */
|
|
fair_status_update($u);
|
|
|
|
if($_SESSION['embed'] != true) {
|
|
//output the current status
|
|
$newstatus=fair_status_info($u);
|
|
if($newstatus!='complete')
|
|
message_push(error(i18n("Fair Information Incomplete")));
|
|
else
|
|
message_push(happy(i18n("Fair Information Complete")));
|
|
}
|
|
|
|
if($_SESSION['embed'] == true) {
|
|
echo "<br />";
|
|
display_messages();
|
|
echo "<h3>".i18n('Fair Information')."</h3>";
|
|
echo "<br />";
|
|
} else {
|
|
//send the header
|
|
send_header("Fair Information",
|
|
array("Science Fair Main" => "fair_main.php")
|
|
);
|
|
}
|
|
/* Load the fair info */
|
|
$q = mysql_query("SELECT * FROM fairs WHERE id={$u['fairs_id']}");
|
|
if(mysql_num_rows($q)) {
|
|
$f = mysql_fetch_assoc($q);
|
|
} else {
|
|
$f = array();
|
|
}
|
|
|
|
$s = ($_SESSION['embed'] == true) ? $_SESSION['embed_submit_url'] : 'fair_info.php';
|
|
echo "<form name=\"fairinfo\" method=\"post\" action=\"$s\">\n";
|
|
echo "<input type=\"hidden\" name=\"action\" value=\"save\" />\n";
|
|
echo "<input type=\"hidden\" name=\"id\" value=\"{$f['id']}\" />\n";
|
|
echo "<table class=\"usereditor\">\n";
|
|
echo '<tr><td class="left">'.i18n('Fair Type').':</td><td class="right">';
|
|
echo "<select name=\"type\" id=\"type\" >";
|
|
foreach($fair_type as $k=>$o) {
|
|
$s = ($f['type'] == $k) ? 'selected="selected"' : '';
|
|
echo "<option value=\"$k\" $s >".i18n($o)."</option>";
|
|
}
|
|
echo "</select></td></tr>";
|
|
echo '<tr><td class="left">'.i18n('Fair Name').':</td><td class="right">';
|
|
echo "<input type=\"text\" name=\"name\" value=\"{$f['name']}\" size=\"40\" />";
|
|
echo '<tr><td class="left">'.i18n('Fair Abbreviation').':</td><td class="right">';
|
|
echo "<input type=\"text\" name=\"abbrv\" value=\"{$f['abbrv']}\" size=\"7\" />";
|
|
echo '<tr><td class="left">'.i18n('Fair Website').':</td><td class="right">';
|
|
if($f['website'] == '') $f['website'] = 'http://';
|
|
echo "<input type=\"text\" name=\"website\" value=\"{$f['website']}\" size=\"40\" />";
|
|
echo '</td></tr>';
|
|
echo '</table>';
|
|
|
|
/* All upstream stuff */
|
|
echo '<div id="upstream">';
|
|
echo "<table class=\"usereditor\">\n";
|
|
echo '<tr><td class="left">'.i18n('Upstream URL').':</td><td class="right">';
|
|
if($f['url'] == '') $f['url'] = 'http://';
|
|
echo "<input type=\"text\" name=\"url\" value=\"{$f['url']}\" size=\"40\" />";
|
|
echo '</td></tr>';
|
|
echo '<tr><td class="left">'.i18n('Upstream Username').':</td><td class="right">';
|
|
echo "<input type=\"text\" name=\"username\" value=\"{$f['username']}\" size=\"20\" />";
|
|
echo '</td></tr>';
|
|
echo '<tr><td class="left">'.i18n('Upstream Password').':</td><td class="right">';
|
|
echo "<input type=\"text\" name=\"password\" value=\"{$f['password']}\" size=\"15\" />";
|
|
echo '</td></tr>';
|
|
echo '<tr><td class="left">'.i18n('Enable stats upload').':</td><td class="right">';
|
|
yesno('enable_stats', $f['enable_stats']);
|
|
echo '</td></tr>';
|
|
echo '<tr><td class="left">'.i18n('Enable awards download').':</td><td class="right">';
|
|
yesno('enable_awards', $f['enable_awards']);
|
|
echo '</td></tr>';
|
|
echo '<tr><td class="left">'.i18n('Enable winners upload').':</td><td class="right">';
|
|
yesno('enable_winners', $f['enable_winners']);
|
|
echo '</td></tr>';
|
|
/* End upstream stuff */
|
|
echo "</table>";
|
|
|
|
echo i18n('* Use the \'Personal\' tab to specify contact information for someone at this fair.');
|
|
echo '</div>';
|
|
echo '<div id="feeder">';
|
|
echo i18n('* The feeder fair must login to this SFIAB to download award lists
|
|
and upload statistics and winners. Use the \'Personal\' tab to specify an
|
|
email and password for the feeder fair, use the email address of a contact at
|
|
the feeder fair. Then give the email/password to that person so they can configure
|
|
their own SFIAB to upload data to this SFIAB.'); echo '</div>';
|
|
|
|
echo "<br />";
|
|
echo "<input type=\"submit\" value=\"".i18n("Save Fair Information")."\" />\n";
|
|
echo "</form>";
|
|
|
|
echo "<br />";
|
|
|
|
|
|
|
|
if($_SESSION['embed'] != true) send_footer();
|
|
|
|
?>
|
|
<script language="javascript" type="text/javascript">
|
|
<!--
|
|
|
|
var fairtype=document.getElementById("type");
|
|
fairtype.onchange=function() { /* Hook onto the onchange */
|
|
var type = this.options[this.selectedIndex].value;
|
|
var upstream_div = document.getElementById("upstream");
|
|
var feeder_div = document.getElementById("feeder");
|
|
if(type == "feeder") {
|
|
upstream_div.style.display="none";
|
|
feeder_div.style.display="block";
|
|
} else if(type == "sfiab") {
|
|
upstream_div.style.display="block";
|
|
feeder_div.style.display="none";
|
|
} else {
|
|
upstream_div.style.display="block";
|
|
feeder_div.style.display="none";
|
|
}
|
|
return true;
|
|
}
|
|
|
|
|
|
fairtype.onchange();
|
|
-->
|
|
</script>
|
|
<?
|
|
|
|
?>
|