forked from science-ation/science-ation
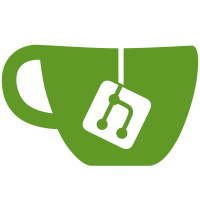
- Add jquery-ui to the scripts, it has support for drag and drop, as well as windows, will convert the popup window to the jquery-ui version next, so we don't have to maintain our own.
815 lines
31 KiB
PHP
815 lines
31 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require_once('../common.inc.php');
|
|
require_once('../user.inc.php');
|
|
user_auth_required('committee', 'admin');
|
|
|
|
switch($_GET['action']) {
|
|
case 'getawardinfo':
|
|
$id = intval($_GET['id']);
|
|
$q=mysql_query("SELECT award_awards.id,
|
|
award_awards.name,
|
|
award_awards.criteria,
|
|
award_awards.description,
|
|
award_awards.order,
|
|
award_awards.presenter,
|
|
award_awards.excludefromac,
|
|
award_awards.cwsfaward,
|
|
award_awards.self_nominate,
|
|
award_awards.schedule_judges,
|
|
award_types.id AS award_types_id,
|
|
award_types.type,
|
|
sponsors.id AS sponsors_id,
|
|
sponsors.organization
|
|
FROM
|
|
award_awards,
|
|
award_types,
|
|
sponsors
|
|
WHERE
|
|
award_awards.id='$id'
|
|
AND award_awards.sponsors_id=sponsors.id
|
|
AND award_awards.award_types_id=award_types.id
|
|
");
|
|
echo mysql_error();
|
|
$r = mysql_fetch_assoc($q);
|
|
$ret = array();
|
|
$ret['id'] = $r['id'];
|
|
$ret['name'] = $r['name'];
|
|
$ret['order'] = $r['order'];
|
|
$ret['criteria'] = $r['criteria'];
|
|
$ret['description'] = $r['description'];
|
|
$ret['presenter'] = $r['presenter'];
|
|
$ret['award_types_id'] = $r['award_types_id'];
|
|
$ret['sponsors_id'] = $r['sponsors_id'];
|
|
$ret['excludefromac'] = $r['excludefromac'];
|
|
$ret['cwsfaward'] = $r['cwsfaward'];
|
|
$ret['self_nominate'] = $r['self_nominate'];
|
|
$ret['schedule_judges'] = $r['schedule_judges'];
|
|
echo json_encode($ret);
|
|
exit;
|
|
case 'saveawardinfo':
|
|
print_r($_POST);
|
|
|
|
/*
|
|
$r=mysql_fetch_object($q);
|
|
$award_awards_id=$r->id;
|
|
$award_awards_name=$r->name;
|
|
$award_awards_order=$r->order;
|
|
$award_awards_criteria=$r->criteria;
|
|
$award_awards_description=$r->description;
|
|
$award_types_id=$r->award_types_id;
|
|
$award_type=$r->type;
|
|
$sponsors_id=$r->sponsors_id;
|
|
$award_sponsor=$r->organization;
|
|
$award_awards_presenter=$r->presenter;
|
|
$award_awards_excludefromac=$r->excludefromac;
|
|
$award_awards_cwsfaward=$r->cwsfaward;
|
|
$award_awards_self_nominate=$r->self_nominate;
|
|
$award_awards_schedule_judges=$r->schedule_judges;*/
|
|
|
|
exit;
|
|
case 'geteligibility':
|
|
$id = intval($_GET['id']);
|
|
//select the current categories that this award is linked to
|
|
$reg = array();
|
|
$q=mysql_query("SELECT * FROM award_awards_projectcategories WHERE award_awards_id='$id'");
|
|
while($r=mysql_fetch_assoc($q)) {
|
|
$ret['categories'][] = $r['projectcategories_id'];
|
|
}
|
|
|
|
//select the current categories that this award is linked to
|
|
$q=mysql_query("SELECT * FROM award_awards_projectdivisions WHERE award_awards_id='$id'");
|
|
while($r=mysql_fetch_assoc($q)) {
|
|
$ret['divisions'][] = $r['projectdivisions_id'];
|
|
}
|
|
echo json_encode($ret);
|
|
exit;
|
|
|
|
case 'orderprizes':
|
|
foreach ($_GET['listItem'] as $position => $item) {
|
|
$sql[] = "UPDATE `table` SET `position` = $position WHERE `id` = $item";
|
|
}
|
|
print_r($sql);
|
|
exit;
|
|
}
|
|
|
|
if($_GET['action']=="edit" || $_GET['action']=="add") {
|
|
send_header(($_GET['action']=="edit") ? "Edit Award" : "Add Award",
|
|
array('Committee Main' => 'committee_main.php',
|
|
'Administration' => 'admin/index.php',
|
|
'Awards Main' => 'admin/awards.php',
|
|
'Awards Management' => 'admin/award_awards.php') );
|
|
} else {
|
|
send_header("Awards Management",
|
|
array('Committee Main' => 'committee_main.php',
|
|
'Administration' => 'admin/index.php',
|
|
'Awards Main' => 'admin/awards.php') );
|
|
}
|
|
|
|
|
|
if($_GET['sponsors_id'] && $_GET['sponsors_id']!="all")
|
|
$_SESSION['sponsors_id']=$_GET['sponsors_id'];
|
|
else if($_GET['sponsors_id']=="all")
|
|
unset($_SESSION['sponsors_id']);
|
|
|
|
if($_GET['award_types_id'] && $_GET['award_types_id']!="all")
|
|
$_SESSION['award_types_id']=$_GET['award_types_id'];
|
|
else if($_GET['award_types_id']=="all")
|
|
unset($_SESSION['award_types_id']);
|
|
|
|
/*
|
|
if($_GET['award_sponsors_confirmed'] && $_GET['award_sponsors_confirmed']!="all")
|
|
$_SESSION['award_sponsors_confirmed']=$_GET['award_sponsors_confirmed'];
|
|
|
|
if($_GET['sponsors_id']=="all")
|
|
unset($_SESSION['sponsors_id']);
|
|
if($_GET['award_types_id']=="all")
|
|
unset($_SESSION['award_types_id']);
|
|
if($_GET['award_sponsors_confirmed']=="all")
|
|
unset($_SESSION['award_sponsors_confirmed']);
|
|
*/
|
|
|
|
$award_types_id=$_SESSION['award_types_id'];
|
|
$sponsors_id=$_SESSION['sponsors_id'];
|
|
//$award_sponsors_confirmed=$_SESSION['award_sponsors_confirmed'];
|
|
|
|
|
|
function popup_begin($name, $title, $width=0, $height=0)
|
|
{
|
|
$size= $width ? "style=\"width:$width%; height:$height%\"" : '';
|
|
echo "<div id=\"popup_{$name}_background\" class=\"popup_background\"></div>";
|
|
echo "<div id=\"popup_$name\" class=\"popup\" $size>
|
|
<a id=\"popup_{$name}_close\" class=\"popup_close\">x</a>
|
|
<h1>{$title}</h1>
|
|
<p id=\"popup_{$name}_body\" class=\"popup_body\">";
|
|
}
|
|
function popup_end()
|
|
{
|
|
echo "</p></div>";
|
|
}
|
|
|
|
|
|
|
|
require_once('../htabs.inc.php');
|
|
|
|
|
|
|
|
?>
|
|
<script type="text/javascript">
|
|
|
|
var award_id = 0;
|
|
function popup_editor(id)
|
|
{
|
|
award_id = id;
|
|
popup_open("editor");
|
|
htabs_open("editortabs");
|
|
|
|
}
|
|
|
|
function update_awardinfo()
|
|
{
|
|
var id = award_id;
|
|
// alert("id="+award_id);
|
|
$.getJSON("<?=$_SERVER['PHP_SELF']?>?action=getawardinfo&id="+id,
|
|
function(json){
|
|
$("#awardinfo_name").val(json.name);
|
|
$("#awardinfo_order").val(json.order);
|
|
$("#awardinfo_sponsors_id").val(json.sponsors_id);
|
|
$("#awardinfo_presenter").val(json.presenter);
|
|
$("#awardinfo_description").val(json.description);
|
|
$("#awardinfo_criteria").val(json.criteria);
|
|
$("#awardinfo_award_types_id").val(json.award_types_id);
|
|
// For some reason, with checkboxes, these have to be arrays
|
|
|
|
$("#awardinfo_excludefromac").val([json.excludefromac]);
|
|
$("#awardinfo_cwsfaward").val([json.cwsfaward]);
|
|
$("#awardinfo_selfnominate").val([json.self_nominate]);
|
|
$("#awardinfo_schedulejudges").val([json.schedule_judges]);
|
|
});
|
|
}
|
|
|
|
|
|
function save_awardinfo()
|
|
{
|
|
$.post("<?$_SERVER['PHP_SELF']?>?action=saveawardinfo", $("#awardinfo").serialize());
|
|
return 0;
|
|
}
|
|
|
|
function update_eligibility()
|
|
{
|
|
var id = award_id;
|
|
$.getJSON("<?=$_SERVER['PHP_SELF']?>?action=geteligibility&id="+id,
|
|
function(json){
|
|
$("[name=eligiblecategories]").val(json.categories);
|
|
$("[name=eligibledivisions]").val(json.divisions);
|
|
});
|
|
}
|
|
|
|
// When the document is ready set up our sortable with it's inherant function(s)
|
|
$(document).ready(function() {
|
|
$("#test-list").sortable({
|
|
handle : '.handle',
|
|
update : function () {
|
|
var order = $('#test-list').sortable('serialize');
|
|
$("#info").load("<?=$_SERVER['PHP_SELF']?>?action=orderprizes&order="+order);
|
|
}
|
|
});
|
|
});
|
|
|
|
</script>
|
|
<?
|
|
|
|
|
|
popup_begin('editor', "Award Editor", 80, 80);
|
|
|
|
htabs_begin('editortabs', array('awardinfo' => array('label' =>'Award',
|
|
'title' => 'Award Info',
|
|
'callback' => 'update_awardinfo'),
|
|
'eligibility' => array('label' =>'Eligibility',
|
|
'title' => 'Eligibility',
|
|
'callback' => 'update_eligibility'),
|
|
'prizes'=> array('label' => 'Prizes',
|
|
'title' => 'Prizes',
|
|
'callback' => ''),
|
|
),'awardinfo');
|
|
|
|
htabs_tab_begin('awardinfo');
|
|
echo "<form id=\"awardinfo\">";
|
|
echo "<table class=\"tableedit\">\n";
|
|
// echo "<tr><td class=\"left\"><hr /></td><td class=\"right\"><hr /></td></tr>\n";
|
|
echo "<tr><td class=\"left\">".i18n("Name").":</td><td class=\"right\"><input type=\"text\" id=\"awardinfo_name\" name=\"name\" value=\"Loading...\" size=\"50\" maxlength=\"128\"><script type=\"text/javascript\">translateButton('name');</script></td></tr>\n";
|
|
echo "<tr><td class=\"left\">".i18n("Order").":</td><td class=\"right\"><input type=\"text\" id=\"awardinfo_order\" name=\"order\" value=\"\" size=\"5\" maxlength=\"5\" />(".i18n("presentation order").")</td></tr>\n";
|
|
|
|
echo "<tr><td class=\"left\">".i18n("Sponsor").":</td><td class=\"right\">";
|
|
$sq=mysql_query("SELECT id,organization FROM sponsors ORDER BY organization");
|
|
echo "<select id=\"awardinfo_sponsors_id\" name=\"sponsors_id\">";
|
|
//only show the "choose a sponsor" option if we are adding,if we are editing, then they must have already chosen one.
|
|
echo $firstsponsor;
|
|
while($sr=mysql_fetch_object($sq)) {
|
|
echo "<option value=\"$sr->id\">".i18n($sr->organization)."</option>";
|
|
}
|
|
echo "</select></td></tr>";
|
|
echo "<tr><td class=\"left\">".i18n("Presenter").":</td><td class=\"right\"><input type=\"text\" id=\"awardinfo_presenter\" name=\"presenter\" value=\"Loading...\" size=\"50\" maxlength=\"128\" /></td></tr>\n";
|
|
|
|
echo "<tr><td class=\"left\">".i18n("Type").":</td><td class=\"right\">";
|
|
$tq=mysql_query("SELECT id,type FROM award_types WHERE year='{$config['FAIRYEAR']}' ORDER BY type");
|
|
echo "<select id=\"awardinfo_award_types_id\" name=\"award_types_id\">";
|
|
//only show the "choose a type" option if we are adding,if we are editing, then they must have already chosen one.
|
|
echo $firsttype;
|
|
while($tr=mysql_fetch_object($tq)) {
|
|
echo "<option value=\"$tr->id\">".i18n($tr->type)."</option>";
|
|
}
|
|
echo "</select>";
|
|
echo "</td></tr>";
|
|
|
|
echo "<tr><td class=\"left\">".i18n("Criteria").":</td><td class=\"right\"><textarea id=\"awardinfo_criteria\" name=\"criteria\" rows=\"3\" cols=\"50\">Loading...</textarea><script type=\"text/javascript\">translateButton('criteria');</script></td></tr>\n";
|
|
echo "<tr><td class=\"left\">".i18n("Description").":</td><td class=\"right\"><textarea id=\"awardinfo_description\" name=\"description\" rows=\"3\" cols=\"50\">Loading...</textarea><script type=\"text/javascript\">translateButton('description');</script></td></tr>\n";
|
|
echo "</table>";
|
|
echo "<h1>Options</h1>";
|
|
echo '<table class="tableedit">';
|
|
echo "<tr><td class=\"left\">";
|
|
echo "<input type=\"checkbox\" id=\"awardinfo_excludefromac\" name=\"excludefromac\" value=\"1\"></td><td class=\"right\">".i18n("Exclude this award from the award ceremony script")."</td></tr>";
|
|
echo "<tr><td class=\"left\">";
|
|
echo "<input type=\"checkbox\" id=\"awardinfo_cwsfaward\" name=\"cwsfaward\" value=\"1\"></td><td class=\"right\">".i18n("This award identifies the students that will be attending the Canada-Wide Science Fair")."</td></tr>";
|
|
echo "<tr><td class=\"left\">";
|
|
echo "<input type=\"checkbox\" id=\"awardinfo_selfnominate\" name=\"self_nominate\" value=\"yes\"></td><td class=\"right\">".i18n("Students can self-nominate for this award (this is usually checked for special awards)")."</td></tr>";
|
|
echo "<tr><td class=\"left\">";
|
|
echo "<input type=\"checkbox\" id=\"awardinfo_schedulejudges\" name=\"schedule_judges\" value=\"yes\"></td><td class=\"right\">".i18n("Allow the Automatic Judge Scheduler to assign judges to this award (usually checked)")."</td></tr>";
|
|
|
|
echo "</table>";
|
|
echo "</form>";
|
|
echo "<input type=\"submit\" onClick=\"save_awardinfo();\" value=\"Save\" />\n";
|
|
|
|
|
|
htabs_tab_end();
|
|
|
|
htabs_tab_begin('eligibility');
|
|
echo "<table class=\"tableedit\">";
|
|
echo "<tr><td class=\"left\">".i18n("Age Categories").":</td><td class=\"right\">";
|
|
// if(count($currentcategories)==0) $class="class=\"error\""; else $class="";
|
|
|
|
//now select all the categories so we can list them all
|
|
$cq=mysql_query("SELECT * FROM projectcategories WHERE year='{$config['FAIRYEAR']}' ORDER BY mingrade");
|
|
echo mysql_error();
|
|
while($cr=mysql_fetch_object($cq)) {
|
|
echo "<input type=\"checkbox\" id=\"eligibility_categories_{$cr->id}\" name=\"eligiblecategories\" value=\"$cr->id\" />".i18n($cr->category)."<br />";
|
|
}
|
|
echo "</td></tr>";
|
|
|
|
echo "<tr><td class=\"left\">".i18n("Divisions").":</td><td class=\"right\">";
|
|
$dq=mysql_query("SELECT * FROM projectdivisions WHERE year='{$config['FAIRYEAR']}' ORDER BY division");
|
|
echo mysql_error();
|
|
while($dr=mysql_fetch_object($dq)) {
|
|
echo "<input type=\"checkbox\" id=\"eligibility_divisions_{$dr->id}\" name=\"eligibledivisions\" value=\"$dr->id\" />".i18n($dr->division)."<br />";
|
|
}
|
|
|
|
echo "</td>";
|
|
echo "</tr>";
|
|
// if(count($currentcategories)==0 || count($currentdivisions)==0)
|
|
// echo "<tr><td colspan=\"2\" class=\"error\">".i18n("At least one age category and one division must be selected")."</td></tr>";
|
|
echo "</table>";
|
|
htabs_tab_end();
|
|
|
|
htabs_tab_begin('prizes');
|
|
?>
|
|
<pre>
|
|
<div id="info">Waiting for update</div>
|
|
</pre>
|
|
<ul id="test-list">
|
|
<li id="listItem_1">
|
|
<img src="arrow.png" alt="move" width="16" height="16" class="handle" />
|
|
<strong>Item 1 </strong>with a link to <a href="http://www.google.co.uk/" rel="nofollow">Google</a>
|
|
</li>
|
|
<li id="listItem_2">
|
|
<img src="arrow.png" alt="move" width="16" height="16" class="handle" />
|
|
<strong>Item 2</strong>
|
|
</li>
|
|
<li id="listItem_3">
|
|
<img src="arrow.png" alt="move" width="16" height="16" class="handle" />
|
|
<strong>Item 3</strong>
|
|
</li>
|
|
<li id="listItem_4">
|
|
<img src="arrow.png" alt="move" width="16" height="16" class="handle" />
|
|
<strong>Item 4</strong>
|
|
</li>
|
|
</ul>
|
|
<?
|
|
htabs_tab_end();
|
|
|
|
htabs_end();
|
|
|
|
|
|
popup_end();
|
|
|
|
|
|
if($_POST['save']=="edit" || $_POST['save']=="add")
|
|
{
|
|
if(!$_POST['award_types_id']) {
|
|
echo error(i18n("Award Type is required"));
|
|
$_GET['action']=$_POST['save'];
|
|
}
|
|
else if(!$_POST['sponsors_id']) {
|
|
echo error(i18n("Award Sponsor is required"));
|
|
$_GET['action']=$_POST['save'];
|
|
}
|
|
else
|
|
{
|
|
|
|
if($_POST['save']=="add")
|
|
{
|
|
$q=mysql_query("INSERT INTO award_awards (sponsors_id,award_types_id,year) VALUES ('".$_POST['sponsors_id']."','".$_POST['award_types_id']."','".$config['FAIRYEAR']."')");
|
|
$id=mysql_insert_id();
|
|
}
|
|
else
|
|
$id=$_POST['id'];
|
|
|
|
$self_nominate = ($_POST['self_nominate'] == 'yes') ? 'yes' : 'no';
|
|
$schedule_judges = ($_POST['schedule_judges'] == 'yes') ? 'yes' : 'no';
|
|
$exec="UPDATE award_awards SET ".
|
|
"name='".mysql_escape_string(stripslashes($_POST['name']))."', ".
|
|
"`order`='".mysql_escape_string(stripslashes($_POST['order']))."', ".
|
|
"sponsors_id='".mysql_escape_string(stripslashes($_POST['sponsors_id']))."', ".
|
|
"award_types_id='".mysql_escape_string(stripslashes($_POST['award_types_id']))."', ".
|
|
"presenter='".mysql_escape_string(stripslashes($_POST['presenter']))."', ".
|
|
"excludefromac='".mysql_escape_string(stripslashes($_POST['excludefromac']))."', ".
|
|
"cwsfaward='".mysql_escape_string(stripslashes($_POST['cwsfaward']))."', ".
|
|
"self_nominate='$self_nominate', ".
|
|
"schedule_judges='$schedule_judges', ".
|
|
"criteria='".mysql_escape_string(stripslashes($_POST['criteria']))."', ".
|
|
"description='".mysql_escape_string(stripslashes($_POST['description']))."' ".
|
|
"WHERE id='$id'";
|
|
|
|
mysql_query($exec);
|
|
echo mysql_error();
|
|
|
|
//whipe out any old award-category links
|
|
mysql_query("DELETE FROM award_awards_projectcategories WHERE award_awards_id='$id'");
|
|
|
|
//now add the new ones
|
|
if(is_array($_POST['eligiblecategories']))
|
|
{
|
|
foreach($_POST['eligiblecategories'] AS $cat)
|
|
{
|
|
mysql_query("INSERT INTO award_awards_projectcategories (award_awards_id,projectcategories_id,year) VALUES ('$id','$cat','".$config['FAIRYEAR']."')");
|
|
}
|
|
}
|
|
|
|
//whipe out any old award-divisions links
|
|
mysql_query("DELETE FROM award_awards_projectdivisions WHERE award_awards_id='$id'");
|
|
|
|
//now add the new ones
|
|
if(is_array($_POST['eligibledivisions']))
|
|
{
|
|
foreach($_POST['eligibledivisions'] AS $div)
|
|
{
|
|
mysql_query("INSERT INTO award_awards_projectdivisions (award_awards_id,projectdivisions_id,year) VALUES ('$id','$div','".$config['FAIRYEAR']."')");
|
|
}
|
|
}
|
|
|
|
if($_POST['save']=="add")
|
|
echo happy("Award successfully added");
|
|
else
|
|
echo happy("Successfully saved changes to award");
|
|
}
|
|
}
|
|
|
|
if($_POST['action']=="reorder")
|
|
{
|
|
if(is_array($_POST['reorder']))
|
|
{
|
|
foreach($_POST['reorder'] AS $key=>$val)
|
|
{
|
|
mysql_query("UPDATE award_awards SET `order`='$val' WHERE id='$key'");
|
|
}
|
|
echo happy("Awards successfully reordered");
|
|
}
|
|
}
|
|
|
|
if($_GET['action']=="delete" && $_GET['delete'])
|
|
{
|
|
mysql_query("DELETE FROM award_awards WHERE id='".$_GET['delete']."'");
|
|
echo happy("Award successfully deleted");
|
|
}
|
|
|
|
if($_GET['action']=="edit" || $_GET['action']=="add")
|
|
{
|
|
//define these here so we dont forget :)
|
|
$currentcategories=array();
|
|
$currentdivisions=array();
|
|
|
|
if($_GET['action']=="edit")
|
|
{
|
|
$buttontext="Save Award";
|
|
|
|
$q=mysql_query("SELECT
|
|
award_awards.id,
|
|
award_awards.name,
|
|
award_awards.criteria,
|
|
award_awards.description,
|
|
award_awards.order,
|
|
award_awards.presenter,
|
|
award_awards.excludefromac,
|
|
award_awards.cwsfaward,
|
|
award_awards.self_nominate,
|
|
award_awards.schedule_judges,
|
|
award_types.id AS award_types_id,
|
|
award_types.type,
|
|
sponsors.id AS sponsors_id,
|
|
sponsors.organization
|
|
|
|
FROM
|
|
award_awards,
|
|
award_types,
|
|
sponsors
|
|
WHERE
|
|
award_awards.year='".$config['FAIRYEAR']."'
|
|
AND award_awards.id='".$_GET['edit']."'
|
|
AND award_awards.sponsors_id=sponsors.id
|
|
AND award_awards.award_types_id=award_types.id
|
|
");
|
|
|
|
|
|
echo mysql_error();
|
|
$r=mysql_fetch_object($q);
|
|
$award_awards_id=$r->id;
|
|
$award_awards_name=$r->name;
|
|
$award_awards_order=$r->order;
|
|
$award_awards_criteria=$r->criteria;
|
|
$award_awards_description=$r->description;
|
|
$award_types_id=$r->award_types_id;
|
|
$award_type=$r->type;
|
|
$sponsors_id=$r->sponsors_id;
|
|
$award_sponsor=$r->organization;
|
|
$award_awards_presenter=$r->presenter;
|
|
$award_awards_excludefromac=$r->excludefromac;
|
|
$award_awards_cwsfaward=$r->cwsfaward;
|
|
$award_awards_self_nominate=$r->self_nominate;
|
|
$award_awards_schedule_judges=$r->schedule_judges;
|
|
|
|
|
|
//select the current categories that this award is linked to
|
|
$ccq=mysql_query("SELECT * FROM award_awards_projectcategories WHERE award_awards_id='$r->id'");
|
|
while($ccr=mysql_fetch_object($ccq))
|
|
$currentcategories[]=$ccr->projectcategories_id;
|
|
|
|
//select the current categories that this award is linked to
|
|
$cdq=mysql_query("SELECT * FROM award_awards_projectdivisions WHERE award_awards_id='$r->id'");
|
|
while($cdr=mysql_fetch_object($cdq))
|
|
$currentdivisions[]=$cdr->projectdivisions_id;
|
|
|
|
|
|
|
|
}
|
|
else if($_GET['action']=="add")
|
|
{
|
|
$buttontext="Add Award";
|
|
$firstsponsor="<option value=\"\">".i18n("Choose a sponsor")."</option>\n";
|
|
$firsttype="<option value=\"\">".i18n("Choose an award type")."</option>\n";
|
|
/* We want these two on by default for new
|
|
* awards */
|
|
$award_awards_self_nominate = 'yes';
|
|
$award_awards_schedule_judges = 'yes';
|
|
}
|
|
$buttontext=i18n($buttontext);
|
|
|
|
//if we have POST values, then they should be used instead of the db values
|
|
//esp for adding, if there is an error then the POST values will be redisplayed
|
|
if($_POST['name']) $award_awards_name=$_POST['name'];
|
|
if($_POST['order']) $award_awards_order=$_POST['order'];
|
|
if($_POST['criteria']) $award_awards_criteria=$_POST['criteria'];
|
|
if($_POST['description']) $award_awards_criteria=$_POST['description'];
|
|
if($_POST['award_types_id']) $award_types_id=$_POST['award_types_id'];
|
|
if($_POST['sponsors_id']) $sponsors_id=$_POST['sponsors_id'];
|
|
if($_POST['eligiblecategories']) $currentcategories=$_POST['eligiblecategories'];
|
|
if($_POST['eligibledivisions']) $currentdivisions=$_POST['eligibledivisions'];
|
|
if($_POST['presenter']) $award_awards_presenter=$_POST['presenter'];
|
|
if($_POST['excludefromac']) $award_awards_excludefromac=$_POST['excludefromac'];
|
|
if($_POST['cwsfaward']) $award_awards_cwsfaward=$_POST['cwsfaward'];
|
|
if($_POST['self_nominate']) $award_awards_self_nominate=$_POST['self_nominate'];
|
|
if($_POST['schedule_judges']) $award_awards_schedule_judges=$_POST['schedule_judges'];
|
|
|
|
|
|
echo "<form method=\"post\" action=\"award_awards.php\">\n";
|
|
echo "<input type=\"hidden\" name=\"save\" value=\"".$_GET['action']."\">\n";
|
|
|
|
if($_GET['action']=="edit")
|
|
echo "<input type=\"hidden\" name=\"id\" value=\"".$_GET['edit']."\">\n";
|
|
|
|
echo "<table class=\"tableedit\">\n";
|
|
echo "<tr><td>".i18n("Name")."</td><td><input type=\"text\" id=\"name\" name=\"name\" value=\"".htmlspecialchars($award_awards_name)."\" size=\"50\" maxlength=\"128\" /><script type=\"text/javascript\">translateButton('name');</script></td></tr>\n";
|
|
echo "<tr><td>".i18n("Order")."</td><td><input type=\"text\" name=\"order\" value=\"".htmlspecialchars($award_awards_order)."\" size=\"5\" maxlength=\"5\" />(".i18n("presentation order").")</td></tr>\n";
|
|
echo "<tr><td>".i18n("Sponsor")."</td><td>";
|
|
$sq=mysql_query("SELECT id,organization FROM sponsors ORDER BY organization");
|
|
echo "<select name=\"sponsors_id\">";
|
|
//only show the "choose a sponsor" option if we are adding,if we are editing, then they must have already chosen one.
|
|
echo $firstsponsor;
|
|
while($sr=mysql_fetch_object($sq))
|
|
{
|
|
if($sr->id == $sponsors_id)
|
|
$sel="selected=\"selected\"";
|
|
else
|
|
$sel="";
|
|
echo "<option $sel value=\"$sr->id\">".i18n($sr->organization)."</option>";
|
|
}
|
|
echo "</select>";
|
|
echo "</td></tr>";
|
|
|
|
echo "<tr><td>".i18n("Presenter")."</td><td><input type=\"text\" name=\"presenter\" value=\"".htmlspecialchars($award_awards_presenter)."\" size=\"50\" maxlength=\"128\" /></td></tr>\n";
|
|
echo "<tr><td>".i18n("Type")."</td><td>";
|
|
$tq=mysql_query("SELECT id,type FROM award_types WHERE year='{$config['FAIRYEAR']}' ORDER BY type");
|
|
echo "<select name=\"award_types_id\">";
|
|
//only show the "choose a type" option if we are adding,if we are editing, then they must have already chosen one.
|
|
echo $firsttype;
|
|
while($tr=mysql_fetch_object($tq))
|
|
{
|
|
if($tr->id == $award_types_id)
|
|
$sel="selected=\"selected\"";
|
|
else
|
|
$sel="";
|
|
echo "<option $sel value=\"$tr->id\">".i18n($tr->type)."</option>";
|
|
}
|
|
echo "</select>";
|
|
echo "</td></tr>";
|
|
|
|
echo "<tr><td>".i18n("Criteria")."</td><td><textarea id=\"criteria\" name=\"criteria\" rows=\"3\" cols=\"50\">".htmlspecialchars($award_awards_criteria)."</textarea><script type=\"text/javascript\">translateButton('criteria');</script></td></tr>\n";
|
|
echo "<tr><td>".i18n("Description")."</td><td><textarea id=\"description\" name=\"description\" rows=\"3\" cols=\"50\">".htmlspecialchars($award_awards_description)."</textarea><script type=\"text/javascript\">translateButton('description');</script></td></tr>\n";
|
|
echo "<tr><td>".i18n("Eligibility")."</td><td>";
|
|
|
|
echo "<table>";
|
|
echo "<tr>";
|
|
echo "<th>".i18n("Age Categories")."</th>";
|
|
echo "<th>".i18n("Divisions")."</th>";
|
|
echo "</tr>";
|
|
if(count($currentcategories)==0) $class="class=\"error\""; else $class="";
|
|
echo "<tr><td $class>";
|
|
|
|
//now select all the categories so we can list them all
|
|
$cq=mysql_query("SELECT * FROM projectcategories WHERE year='".$config['FAIRYEAR']."' ORDER BY mingrade");
|
|
echo mysql_error();
|
|
while($cr=mysql_fetch_object($cq))
|
|
{
|
|
if(in_array($cr->id,$currentcategories))
|
|
$ch="checked=\"checked\"";
|
|
else
|
|
$ch="";
|
|
|
|
echo "<input $ch type=\"checkbox\" name=\"eligiblecategories[]\" value=\"$cr->id\" />".i18n($cr->category)."<br />";
|
|
}
|
|
echo "</td>";
|
|
if(count($currentdivisions)==0) $class="class=\"error\""; else $class="";
|
|
echo "<td $class>";
|
|
|
|
$dq=mysql_query("SELECT * FROM projectdivisions WHERE year='".$config['FAIRYEAR']."' ORDER BY division");
|
|
echo mysql_error();
|
|
while($dr=mysql_fetch_object($dq))
|
|
{
|
|
if(in_array($dr->id,$currentdivisions))
|
|
$ch="checked=\"checked\"";
|
|
else
|
|
$ch="";
|
|
echo "<input $ch type=\"checkbox\" name=\"eligibledivisions[]\" value=\"$dr->id\" />".i18n($dr->division)."<br />";
|
|
}
|
|
|
|
echo "</td>";
|
|
echo "</tr>";
|
|
if(count($currentcategories)==0 || count($currentdivisions)==0)
|
|
echo "<tr><td colspan=\"2\" class=\"error\">".i18n("At least one age category and one division must be selected")."</td></tr>";
|
|
echo "</table>";
|
|
|
|
echo "</td></tr>";
|
|
|
|
echo "<tr><td align=\"right\">";
|
|
if($award_awards_excludefromac==1) $ch="checked=\"checked\""; else $ch="";
|
|
echo "<input $ch type=\"checkbox\" name=\"excludefromac\" value=\"1\"></td><td>".i18n("Exclude this award from the award ceremony script")."</td></tr>";
|
|
echo "<tr><td align=\"right\">";
|
|
if($award_awards_cwsfaward==1) $ch="checked=\"checked\""; else $ch="";
|
|
echo "<input $ch type=\"checkbox\" name=\"cwsfaward\" value=\"1\"></td><td>".i18n("This award identifies the students that will be attending the Canada-Wide Science Fair")."</td></tr>";
|
|
echo "<tr><td align=\"right\">";
|
|
$ch = ($award_awards_self_nominate=='yes') ? "checked=\"checked\"" : '';
|
|
echo "<input $ch type=\"checkbox\" name=\"self_nominate\" value=\"yes\"></td><td>".i18n("Students can self-nominate for this award (this is usually checked for special awards)")."</td></tr>";
|
|
echo "<tr><td align=\"right\">";
|
|
$ch = ($award_awards_schedule_judges=='yes') ? "checked=\"checked\"" : '';
|
|
echo "<input $ch type=\"checkbox\" name=\"schedule_judges\" value=\"yes\"></td><td>".i18n("Allow the Automatic Judge Scheduler to assign judges to this award (usually checked)")."</td></tr>";
|
|
|
|
echo "<tr><td colspan=\"2\" align=\"center\"><input type=\"submit\" value=\"$buttontext\" /></td></tr>\n";
|
|
|
|
echo "</table>\n";
|
|
echo "</form>\n";
|
|
}
|
|
else
|
|
{
|
|
|
|
|
|
echo "<br />";
|
|
echo i18n("Filter By:");
|
|
echo "<form method=\"get\" action=\"award_awards.php\" name=\"filterchange\">";
|
|
echo "<table><tr><td colspan=\"2\">";
|
|
|
|
$q=mysql_query("SELECT id,organization FROM sponsors ORDER BY organization");
|
|
echo "<select name=\"sponsors_id\" onchange=\"document.forms.filterchange.submit()\">";
|
|
echo "<option value=\"all\">".i18n("All Sponsors")."</option>";
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
if($r->id == $sponsors_id)
|
|
{
|
|
$sel="selected=\"selected\"";
|
|
$sponsors_organization=$r->organization;
|
|
}
|
|
else
|
|
$sel="";
|
|
echo "<option $sel value=\"$r->id\">".i18n($r->organization)."</option>";
|
|
}
|
|
echo "</select>";
|
|
echo "</td></tr>";
|
|
echo "<tr><td>";
|
|
|
|
$q=mysql_query("SELECT id,type FROM award_types WHERE year='{$config['FAIRYEAR']}' ORDER BY type");
|
|
echo "<select name=\"award_types_id\" onchange=\"document.forms.filterchange.submit()\">";
|
|
echo "<option value=\"all\">".i18n("All Award Types")."</option>";
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
if($r->id == $award_types_id)
|
|
{
|
|
$sel="selected=\"selected\"";
|
|
$award_types_type=$r->type;
|
|
}
|
|
else
|
|
$sel="";
|
|
echo "<option $sel value=\"$r->id\">".i18n($r->type)."</option>";
|
|
}
|
|
echo "</select>";
|
|
echo "</td><td>";
|
|
|
|
/*
|
|
//FIXME: 'confirmed' no longer exists, we need to lookup their sponsorship record and check the status there, either pending, confirmed or received, dunno if it makes sense to put that here or not..
|
|
|
|
echo "<select name=\"award_sponsors_confirmed\" onchange=\"document.forms.filterchange.submit()\">";
|
|
if($award_sponsors_confirmed=="all") $sel="selected=\"selected\""; else $sel="";
|
|
echo "<option value=\"all\">".i18n("Any Status")."</option>";
|
|
if($award_sponsors_confirmed=="yes") $sel="selected=\"selected\""; else $sel="";
|
|
echo "<option $sel value=\"yes\">".i18n("Confirmed Only")."</option>";
|
|
if($award_sponsors_confirmed=="no") $sel="selected=\"selected\""; else $sel="";
|
|
echo "<option $sel value=\"no\">".i18n("Unconfirmed Only")."</option>";
|
|
echo "</select>";
|
|
*/
|
|
echo "</form>";
|
|
echo "</td></tr>";
|
|
echo "</table>";
|
|
|
|
|
|
|
|
echo "<br />";
|
|
echo "<a href=\"award_awards.php?sponsors_id=$sponsors_id&award_types_id=$award_types_id&action=add\">".i18n("Add New Award")."</a>\n";
|
|
echo "<br />";
|
|
|
|
if($sponsors_id) $where_asi="AND sponsors_id='$sponsors_id'";
|
|
if($award_types_id) $where_ati="AND award_types_id='$award_types_id'";
|
|
// if($award_sponsors_confirmed) $where_asc="AND award_sponsors.confirmed='$award_sponsors_confirmed'";
|
|
|
|
if(!$orderby) $orderby="order";
|
|
|
|
$q=mysql_query("SELECT
|
|
award_awards.id,
|
|
award_awards.name,
|
|
award_awards.order,
|
|
award_awards.award_source_fairs_id,
|
|
award_types.type,
|
|
sponsors.organization
|
|
|
|
FROM
|
|
award_awards,
|
|
award_types,
|
|
sponsors
|
|
WHERE
|
|
award_awards.year='".$config['FAIRYEAR']."'
|
|
$where_asi
|
|
$where_ati
|
|
$where_asc
|
|
AND award_awards.sponsors_id=sponsors.id
|
|
AND award_awards.award_types_id=award_types.id
|
|
AND award_types.year='".$config['FAIRYEAR']."'
|
|
ORDER BY `$orderby`");
|
|
|
|
echo mysql_error();
|
|
|
|
if(mysql_num_rows($q))
|
|
{
|
|
echo "<form method=\"post\" action=\"award_awards.php\">";
|
|
echo "<input type=\"hidden\" name=\"action\" value=\"reorder\">";
|
|
|
|
echo "<table class=\"tableview\">";
|
|
echo "<tr>";
|
|
echo " <th>".i18n("Order")."</th>";
|
|
echo " <th>".i18n("Sponsor")."</th>";
|
|
echo " <th>".i18n("Type")."</th>";
|
|
echo " <th>".i18n("Name")."</th>";
|
|
echo " <th>".i18n("Prizes")."</th>";
|
|
echo " <th>".i18n("Actions")."</th>";
|
|
echo "</tr>\n";
|
|
|
|
|
|
$hasexternal=false;
|
|
while($r=mysql_fetch_object($q))
|
|
{
|
|
if($r->award_source_fairs_id){ $cl="class=\"externalaward\""; $hasexternal=true; } else $cl="";
|
|
echo "<tr $cl>\n";
|
|
echo " <td><input type=\"text\" name=\"reorder[$r->id]\" value=\"$r->order\" size=\"3\" /></td>\n";
|
|
echo " <td>$r->organization</td>\n";
|
|
echo " <td>$r->type</td>\n";
|
|
echo " <td><a onclick=\"popup_editor({$r->id});\">$r->name</a></td>\n";
|
|
|
|
$numq=mysql_query("SELECT COUNT(id) AS num FROM award_prizes WHERE award_awards_id='$r->id'");
|
|
$numr=mysql_fetch_object($numq);
|
|
$numprizes=$numr->num;
|
|
|
|
|
|
echo " <td align=\"center\" valign=\"top\">";
|
|
echo "$numprizes ";
|
|
echo "<a href=\"award_prizes.php?award_awards_id=$r->id\"><img alt=\"view\" border=\"0\" src=\"".$config['SFIABDIRECTORY']."/images/16/viewmag.".$config['icon_extension']."\"></a>";
|
|
echo "</td>";
|
|
|
|
echo " <td align=\"center\">";
|
|
echo "<a href=\"award_awards.php?action=edit&edit=$r->id\"><img border=\"0\" src=\"".$config['SFIABDIRECTORY']."/images/16/edit.".$config['icon_extension']."\"></a>";
|
|
echo " ";
|
|
echo "<a onclick=\"return confirmClick('Are you sure you want to remove this award?')\" href=\"award_awards.php?action=delete&delete=$r->id\"><img border=0 src=\"".$config['SFIABDIRECTORY']."/images/16/button_cancel.".$config['icon_extension']."\"></a>";
|
|
|
|
echo " </td>\n";
|
|
echo "</tr>\n";
|
|
}
|
|
if($hasexternal)
|
|
echo "<tr class=\"externalaward\"><td colspan=\"6\">".i18n("Indicates award imported from an external source")."</td></tr>";
|
|
echo "</table>\n";
|
|
echo "<input type=\"submit\" value=\"".i18n("Re-order awards")."\" />";
|
|
echo "</form>";
|
|
}
|
|
echo "<br />";
|
|
echo "<a href=\"award_prizes.php?award_awards_id=-1\">Edit prizes for the generic prize template</a>";
|
|
|
|
}
|
|
|
|
send_footer();
|
|
|
|
?>
|