forked from science-ation/science-ation
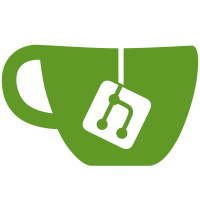
Update LPDF to add a "..." to a table field if all of the text didnt fit in the table field
594 lines
18 KiB
PHP
594 lines
18 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
class lpdf
|
|
{
|
|
var $pdf;
|
|
var $yloc=10.25;
|
|
var $page_header;
|
|
var $page_subheader;
|
|
var $pagenumber;
|
|
var $logoimage;
|
|
|
|
var $page_style="normal";
|
|
|
|
var $page_margin=0.75;
|
|
//these are defaults, they get overwritten with the first call to newPage(width,height)
|
|
var $page_width=8.5;
|
|
var $page_height=11;
|
|
|
|
//all of these are overwritten by setNametagDimensions(width,height);
|
|
var $nametag_width=4;
|
|
var $nametag_height=3;
|
|
var $nametags_per_row=2;
|
|
var $nametags_per_column=3;
|
|
var $nametags_per_page=6;
|
|
|
|
var $nametags_start_xpos;
|
|
var $nametags_start_ypos;
|
|
|
|
var $current_nametag_index=0;
|
|
var $current_nametag_col_index=0;
|
|
var $current_nametag_row_index=1;
|
|
|
|
var $currentFontSize=12;
|
|
|
|
function loc($inch)
|
|
{
|
|
return $inch*72;
|
|
}
|
|
|
|
|
|
function addHeaderAndFooterToPage()
|
|
{
|
|
//The title of the fair
|
|
$this->yloc=$this->page_height-$this->page_margin;
|
|
$height['title']=0.25;
|
|
$height['subtitle']=0.22;
|
|
|
|
pdf_setfont($this->pdf,$this->headerfont,18);
|
|
pdf_show_boxed($this->pdf,$this->page_header,$this->loc($this->page_margin),$this->loc($this->yloc),$this->loc($this->content_width),$this->loc($height['title']),"center",null);
|
|
$this->yloc-=$height['title'];
|
|
|
|
pdf_setfont($this->pdf,$this->headerfont,14);
|
|
pdf_show_boxed($this->pdf,$this->page_subheader,$this->loc($this->page_margin),$this->loc($this->yloc),$this->loc($this->content_width),$this->loc($height['subtitle']),"center",null);
|
|
$this->yloc-=$height['subtitle'];
|
|
|
|
//only put the logo on the page if we actually have the logo
|
|
if($this->logoimage)
|
|
{
|
|
//now place the logo image in the top-left-ish
|
|
pdf_place_image($this->pdf,$this->logoimage,$this->loc($this->page_margin),$this->loc($this->yloc+.02),.20);
|
|
}
|
|
|
|
//header line
|
|
pdf_moveto($this->pdf,$this->loc($this->page_margin-0.25),$this->loc($this->yloc));
|
|
pdf_lineto($this->pdf,$this->loc($this->page_width-$this->page_margin+0.25),$this->loc($this->yloc));
|
|
pdf_stroke($this->pdf);
|
|
$this->yloc-=0.20;
|
|
|
|
//now put a nice little footer at the bottom
|
|
$footertext=date("Y-m-d h:ia")." - ".$this->page_header." - ".$this->page_subheader;
|
|
|
|
$footerwidth=pdf_stringwidth($this->pdf,$footertext,$this->normalfont,9);
|
|
pdf_setfont($this->pdf,$this->normalfont,9);
|
|
pdf_show_xy($this->pdf,$footertext,$this->loc($this->page_width/2)-$footerwidth/2,$this->loc(0.5));
|
|
|
|
//footer line
|
|
pdf_moveto($this->pdf,$this->loc($this->page_margin-0.25),$this->loc($this->page_margin-0.05));
|
|
pdf_lineto($this->pdf,$this->loc($this->page_width-$this->page_margin+0.25),$this->loc($this->page_margin-0.05));
|
|
pdf_stroke($this->pdf);
|
|
}
|
|
|
|
function newPage($width="",$height="")
|
|
{
|
|
if($width && $height)
|
|
{
|
|
$this->page_width=$width;
|
|
$this->page_height=$height;
|
|
$this->content_width=$width-(2*$this->page_margin);
|
|
}
|
|
|
|
if($this->pagenumber>0)
|
|
pdf_end_page($this->pdf);
|
|
$this->pagenumber++;
|
|
|
|
//Letter size (8.5 x 11) is 612,792
|
|
pdf_begin_page($this->pdf,$this->loc($this->page_width),$this->loc($this->page_height));
|
|
pdf_setlinewidth($this->pdf,0.3);
|
|
|
|
if($this->page_style=="normal")
|
|
{
|
|
$this->addHeaderAndFooterToPage();
|
|
//make sure we set the font back to whatever it used to be
|
|
//because adding header/footer changes the fontsize
|
|
$this->setFontSize($this->currentFontSize);
|
|
}
|
|
}
|
|
|
|
function vspace($space)
|
|
{
|
|
$this->yloc-=$space;
|
|
}
|
|
|
|
function setFontSize($size)
|
|
{
|
|
$this->currentFontSize=$size;
|
|
pdf_setfont($this->pdf,$this->normalfont,$size);
|
|
$leading=round($size*1.3);
|
|
pdf_set_value($this->pdf,"leading",$leading);
|
|
}
|
|
|
|
function addText($text,$align="left")
|
|
{
|
|
$fontsize=pdf_get_value($this->pdf,"fontsize",0);
|
|
$lineheight=ceil($fontsize*1.3);
|
|
//the line height should be 1.2 * fontsize (approx)
|
|
$stringwidth=pdf_stringwidth($this->pdf,$text,$this->normalfont,$fontsize);
|
|
|
|
$textstr=$text;
|
|
|
|
$nr=0;
|
|
$prevnr=-1;
|
|
do
|
|
{
|
|
//echo "textstr=$textstr";
|
|
$len=strlen($textstr);
|
|
// echo "(lh:$lineheight nr:$nr len:$len)".$textstr;
|
|
|
|
$nl=false;
|
|
//now lets handle a newline at the beginning, just rip it off and move the yloc ourself
|
|
while($textstr[0]=="\n")
|
|
{
|
|
$textstr=substr($textstr,1);
|
|
$this->yloc-=$lineheight/72;
|
|
$nl=true;
|
|
}
|
|
|
|
if(!$nl)
|
|
$this->yloc-=$lineheight/72;
|
|
|
|
|
|
$nr=pdf_show_boxed($this->pdf,$textstr, $this->loc($this->page_margin),$this->loc($this->yloc),$this->loc($this->content_width),$lineheight,$align,null);
|
|
if($this->yloc< (0.9 + $lineheight/72) )
|
|
$this->newPage();
|
|
|
|
if($nr==$prevnr)
|
|
{
|
|
echo "breaking because nr==prevnr ($nr==$prevnr) trying to output [$textstr] (debug: fontsize=$fontsize, lineheight=$lineheight, stringwidth=$stringwidth, left=".$this->loc(0.75).", top=".$this->loc($this->yloc).", width=".$this->loc(7).", height=$lineheight)\n";
|
|
break;
|
|
}
|
|
|
|
$prevnr=$nr;
|
|
// printf("x=%f y=%f w=%f h=%f",$this->loc(0.75),$this->loc($this->yloc),$this->loc(7),$lineheight);
|
|
// echo "$nr didnt fit";
|
|
// echo "<br>doing: substr($textstr,-$nr) <br>";
|
|
$textstr=substr($textstr,-$nr);
|
|
// echo "nr=$nr";
|
|
} while($nr>0);
|
|
|
|
// pdf_rect($this->pdf,$this->loc(0.75),$this->loc($this->yloc),$this->loc(7),$height);
|
|
|
|
}
|
|
|
|
function addNametagText($Y,$text,$align="center")
|
|
{
|
|
$fontsize=pdf_get_value($this->pdf,"fontsize",0);
|
|
$lineheight=ceil($fontsize*1.3);
|
|
|
|
$textstr=$text;
|
|
|
|
$texty=$this->nametag_current_ypos-$Y;
|
|
|
|
$nr=0;
|
|
$prevnr=-1;
|
|
do
|
|
{
|
|
//echo "textstr=$textstr";
|
|
$len=strlen($textstr);
|
|
// echo "(lh:$lineheight nr:$nr len:$len)".$textstr;
|
|
|
|
$nl=false;
|
|
//now lets handle a newline at the beginning, just rip it off and move the yloc ourself
|
|
while($textstr[0]=="\n")
|
|
{
|
|
$textstr=substr($textstr,1);
|
|
$texty-=$lineheight/72;
|
|
$nl=true;
|
|
}
|
|
|
|
if(!$nl)
|
|
$texty-=$lineheight/72;
|
|
$nr=pdf_show_boxed($this->pdf,$textstr, $this->loc($this->nametag_current_xpos),$this->loc($texty),$this->loc($this->nametag_width),$lineheight,$align,null);
|
|
|
|
if($nr==$prevnr)
|
|
{
|
|
echo "in addNametagText - breaking because nr==prevnr ($nr==$prevnr) ";
|
|
break;
|
|
}
|
|
|
|
$prevnr=$nr;
|
|
// printf("x=%f y=%f w=%f h=%f",$this->loc(0.75),$this->loc($this->yloc),$this->loc(7),$lineheight);
|
|
// echo "$nr didnt fit";
|
|
// echo "<br>doing: substr($textstr,-$nr) <br>";
|
|
$textstr=substr($textstr,-$nr);
|
|
// echo "nr=$nr";
|
|
} while($nr>0);
|
|
|
|
|
|
/*
|
|
$this->nametag_current_ypos-=$lineheight/72;
|
|
pdf_show_boxed($this->pdf,$text,
|
|
$this->loc($this->nametag_current_xpos),
|
|
$this->loc($this->nametag_current_ypos),
|
|
$this->loc($this->nametag_width),
|
|
$lineheight,
|
|
"center",
|
|
null);
|
|
*/
|
|
}
|
|
|
|
function newNametag()
|
|
{
|
|
if($this->current_nametag_index==$this->nametags_per_page)
|
|
{
|
|
|
|
$this->newPage();
|
|
$this->current_nametag_index=1;
|
|
$this->current_nametag_col_index=0;
|
|
$this->current_nametag_row_index=1;
|
|
}
|
|
else
|
|
{
|
|
$this->current_nametag_index++;
|
|
}
|
|
|
|
if($this->current_nametag_col_index==$this->nametags_per_row)
|
|
{
|
|
|
|
$this->current_nametag_col_index=1;
|
|
$this->current_nametag_row_index++;
|
|
}
|
|
else
|
|
{
|
|
$this->current_nametag_col_index++;
|
|
}
|
|
|
|
$this->nametag_current_ypos=$this->nametags_start_ypos-(($this->current_nametag_row_index-1)*$this->nametag_height)-$this->nametag_height;
|
|
$this->nametag_current_xpos=$this->nametags_start_xpos+(($this->current_nametag_col_index-1)*$this->nametag_width);
|
|
|
|
pdf_rect($this->pdf,
|
|
$this->loc($this->nametag_current_xpos),
|
|
$this->loc($this->nametag_current_ypos),
|
|
$this->loc($this->nametag_width),
|
|
$this->loc($this->nametag_height));
|
|
pdf_stroke($this->pdf);
|
|
|
|
$this->nametag_current_ypos+=$this->nametag_height-0.25;
|
|
|
|
//only put the logo on the nametag if we actually have the logo
|
|
if($this->logoimage)
|
|
{
|
|
//now place the logo image in the top-left-ish
|
|
pdf_place_image($this->pdf,$this->logoimage,
|
|
$this->loc($this->nametag_current_xpos+0.05),
|
|
$this->loc($this->nametag_current_ypos-0.50),0.2);
|
|
}
|
|
|
|
$height['title']=0.50;
|
|
$this->nametag_current_ypos-=$height['title'];
|
|
pdf_setfont($this->pdf,$this->headerfont,14);
|
|
pdf_show_boxed($this->pdf,$this->page_header,
|
|
$this->loc($this->nametag_current_xpos+0.65),
|
|
$this->loc($this->nametag_current_ypos),
|
|
$this->loc($this->nametag_width-0.70),
|
|
$this->loc($height['title']),
|
|
"center",
|
|
null);
|
|
pdf_setfont($this->pdf,$this->normalfont,10);
|
|
|
|
|
|
}
|
|
|
|
function addTextX($text,$xpos)
|
|
{
|
|
pdf_show_xy($this->pdf,$text,$this->loc($xpos),$this->loc($this->yloc));
|
|
}
|
|
|
|
function nextLine()
|
|
{
|
|
$this->yloc-=$this->currentFontSize*1.4/72;
|
|
}
|
|
|
|
function hr()
|
|
{
|
|
pdf_moveto($this->pdf,$this->loc($this->page_margin-0.25),$this->loc($this->yloc));
|
|
pdf_lineto($this->pdf,$this->loc($this->page_width-$this->page_margin+0.25),$this->loc($this->yloc));
|
|
pdf_stroke($this->pdf);
|
|
$this->yloc-=0.25;
|
|
}
|
|
|
|
function hline($x1,$x2)
|
|
{
|
|
pdf_moveto($this->pdf,$this->loc($x1),$this->loc($this->yloc));
|
|
pdf_lineto($this->pdf,$this->loc($x2),$this->loc($this->yloc));
|
|
pdf_stroke($this->pdf);
|
|
}
|
|
|
|
function heading($text)
|
|
{
|
|
//if we are close to the bottom, lets just move the whole heading to the next page.
|
|
//no point putting the heading here then the new text on the next page.
|
|
|
|
//12/72 is height of the heading
|
|
//4/72 is the space under the heading
|
|
if($this->yloc< (1.1 + 12/72 + 4/72) )
|
|
$this->newPage();
|
|
|
|
pdf_setfont($this->pdf,$this->headerfont,12);
|
|
//move down the full line height
|
|
$this->yloc-=12/72;
|
|
pdf_show_xy($this->pdf,$text,$this->loc($this->page_margin),$this->loc($this->yloc));
|
|
pdf_setfont($this->pdf,$this->normalfont,$this->currentFontSize);
|
|
//now leave some space under the heading (4 is 1/3 of 12, so 1/3 of the line height we leave)
|
|
$this->yloc-=4/72;
|
|
|
|
}
|
|
|
|
|
|
function addTable($table,$align="center")
|
|
{
|
|
if(is_array($table['header']))
|
|
{
|
|
$table_cols=count($table['header']);
|
|
$height['tableheader']=0.2;
|
|
}
|
|
else
|
|
{
|
|
$table_cols=count($table['data']);
|
|
$height['tableheader']=0;
|
|
}
|
|
$height['tabledata']=0.18;
|
|
|
|
$this->yloc-=$height['tableheader'];
|
|
$top_of_table=$this->yloc;
|
|
|
|
$table_width=array_sum($table['widths']);
|
|
$table_padding=0.03;
|
|
|
|
switch($align)
|
|
{
|
|
case "center"; $xpos_of_table=($this->page_width-$table_width)/2; break;
|
|
case "left"; $xpos_of_table=$this->page_margin; break;
|
|
case "right"; $xpos_of_table=$this->page_width-$this->page_margin-$table_width; break;
|
|
}
|
|
|
|
//draw the top line of the table (above the table header)
|
|
pdf_moveto($this->pdf,$this->loc($xpos_of_table),$this->loc($this->yloc+$height['tableheader']));
|
|
pdf_lineto($this->pdf,$this->loc($xpos_of_table+$table_width),$this->loc($this->yloc+$height['tableheader']));
|
|
pdf_stroke($this->pdf);
|
|
|
|
//do the header first
|
|
if(is_array($table['header']))
|
|
{
|
|
//draw the top line of the table (below the table header)
|
|
pdf_moveto($this->pdf,$this->loc($xpos_of_table),$this->loc($this->yloc));
|
|
pdf_lineto($this->pdf,$this->loc($xpos_of_table+$table_width),$this->loc($this->yloc));
|
|
pdf_stroke($this->pdf);
|
|
|
|
$xpos=$xpos_of_table;
|
|
pdf_setfont($this->pdf,$this->headerfont,12);
|
|
|
|
for($c=0;$c<$table_cols;$c++)
|
|
{
|
|
$head=$table['header'][$c];
|
|
$width=$table['widths'][$c];
|
|
|
|
pdf_show_boxed($this->pdf,$head,$this->loc($xpos),$this->loc($this->yloc),$this->loc($width),$this->loc($height['tableheader']),"center",null);
|
|
$xpos+=$width;
|
|
}
|
|
|
|
}
|
|
|
|
//now do the data in the table
|
|
if($table['data'])
|
|
{
|
|
pdf_setfont($this->pdf,$this->normalfont,10);
|
|
foreach($table['data'] AS $dataline)
|
|
{
|
|
$this->yloc-=$height['tabledata'];
|
|
$xpos=$xpos_of_table;
|
|
for($c=0;$c<$table_cols;$c++)
|
|
{
|
|
$width=$table['widths'][$c];
|
|
|
|
$notfit=pdf_show_boxed($this->pdf,$dataline[$c],$this->loc($xpos+$table_padding),$this->loc($this->yloc),$this->loc($width-2*$table_padding),$this->loc($height['tabledata']),$table['dataalign'][$c],null);
|
|
|
|
//put a little "..." at the end of the field
|
|
if($notfit)
|
|
{
|
|
pdf_setfont($this->pdf,$this->normalfont,8);
|
|
pdf_show_boxed($this->pdf,"...",$this->loc($xpos+$width-0.10),$this->loc($this->yloc-0.05),$this->loc(0.10),$this->loc($height['tabledata']),$table['dataalign'][$c],null);
|
|
pdf_setfont($this->pdf,$this->normalfont,10);
|
|
}
|
|
|
|
$xpos+=$width;
|
|
}
|
|
|
|
//draw the line below the table data)
|
|
pdf_moveto($this->pdf,$this->loc($xpos_of_table),$this->loc($this->yloc));
|
|
pdf_lineto($this->pdf,$this->loc($xpos_of_table+$table_width),$this->loc($this->yloc));
|
|
pdf_stroke($this->pdf);
|
|
|
|
if($this->yloc<1.1)
|
|
{
|
|
//now draw all the vertical lines
|
|
$xpos=$xpos_of_table;
|
|
for($c=0;$c<$table_cols;$c++)
|
|
{
|
|
$width=$table['widths'][$c];
|
|
//draw the line below the table data)
|
|
pdf_moveto($this->pdf,$this->loc($xpos),$this->loc($top_of_table+$height['tableheader']));
|
|
pdf_lineto($this->pdf,$this->loc($xpos),$this->loc($this->yloc));
|
|
pdf_stroke($this->pdf);
|
|
$xpos+=$width;
|
|
}
|
|
|
|
//and the final line on the right side of the table:
|
|
pdf_moveto($this->pdf,$this->loc($xpos),$this->loc($top_of_table+$height['tableheader']));
|
|
pdf_lineto($this->pdf,$this->loc($xpos),$this->loc($this->yloc));
|
|
pdf_stroke($this->pdf);
|
|
|
|
$this->newPage($this->page_width,$this->page_height);
|
|
|
|
$this->yloc-=$height['tableheader'];
|
|
$top_of_table=$this->yloc;
|
|
|
|
//draw the top line of the table (above the table header)
|
|
pdf_moveto($this->pdf,$this->loc($xpos_of_table),$this->loc($this->yloc+$height['tableheader']));
|
|
pdf_lineto($this->pdf,$this->loc($xpos_of_table+$table_width),$this->loc($this->yloc+$height['tableheader']));
|
|
pdf_stroke($this->pdf);
|
|
|
|
//draw the top line of the table (below the table header)
|
|
pdf_moveto($this->pdf,$this->loc($xpos_of_table),$this->loc($this->yloc));
|
|
pdf_lineto($this->pdf,$this->loc($xpos_of_table+$table_width),$this->loc($this->yloc));
|
|
pdf_stroke($this->pdf);
|
|
|
|
//do the header first
|
|
if($table['header'])
|
|
{
|
|
pdf_setfont($this->pdf,$this->headerfont,12);
|
|
|
|
$xpos=$xpos_of_table;
|
|
|
|
for($c=0;$c<$table_cols;$c++)
|
|
{
|
|
$head=$table['header'][$c];
|
|
$width=$table['widths'][$c];
|
|
|
|
pdf_show_boxed($this->pdf,$head,$this->loc($xpos),$this->loc($this->yloc),$this->loc($width),$this->loc($height['tableheader']),"center",null);
|
|
$xpos+=$width;
|
|
}
|
|
|
|
}
|
|
pdf_setfont($this->pdf,$this->normalfont,10);
|
|
}
|
|
}
|
|
}
|
|
|
|
//now draw all the vertical lines
|
|
$xpos=$xpos_of_table;
|
|
for($c=0;$c<$table_cols;$c++)
|
|
{
|
|
$width=$table['widths'][$c];
|
|
//draw the line below the table data)
|
|
pdf_moveto($this->pdf,$this->loc($xpos),$this->loc($top_of_table+$height['tableheader']));
|
|
pdf_lineto($this->pdf,$this->loc($xpos),$this->loc($this->yloc));
|
|
pdf_stroke($this->pdf);
|
|
$xpos+=$width;
|
|
}
|
|
|
|
//and the final line on the right side of the table:
|
|
pdf_moveto($this->pdf,$this->loc($xpos),$this->loc($top_of_table+$height['tableheader']));
|
|
pdf_lineto($this->pdf,$this->loc($xpos),$this->loc($this->yloc));
|
|
pdf_stroke($this->pdf);
|
|
|
|
}
|
|
|
|
//page styles: "normal" "nametags" "empty"
|
|
function setPageStyle($style="normal")
|
|
{
|
|
$this->page_style=$style;
|
|
}
|
|
|
|
function setNametagDimensions($width,$height)
|
|
{
|
|
$this->nametag_width=$width;
|
|
$this->nametag_height=$height;
|
|
|
|
$this->nametags_per_row=floor($this->page_width/$width);
|
|
$this->nametags_per_column=floor($this->page_height/$height);
|
|
$this->nametags_per_page=$this->nametags_per_row * $this->nametags_per_column;
|
|
|
|
$this->nametags_start_xpos=($this->page_width-$this->nametags_per_row*$width)/2;
|
|
$this->nametags_start_ypos=$this->page_height-($this->page_height-$this->nametags_per_column*$height)/2;
|
|
|
|
}
|
|
|
|
|
|
function output()
|
|
{
|
|
pdf_end_page($this->pdf);
|
|
|
|
//only close the image if it was opened to begin with
|
|
if($this->logoimage)
|
|
pdf_close_image($this->pdf,$this->logoimage);
|
|
|
|
pdf_close($this->pdf);
|
|
$pdfdata=pdf_get_buffer($this->pdf);
|
|
|
|
$filename=strtolower($this->page_subheader);
|
|
$filename=ereg_replace("[^a-z0-9]","_",$filename);
|
|
|
|
header("Content-type: application/pdf");
|
|
header("Content-disposition: inline; filename=sfiab_".$filename.".pdf");
|
|
header("Content-length: ".strlen($pdfdata));
|
|
echo $pdfdata;
|
|
}
|
|
|
|
function lpdf($header,$subheader,$logo)
|
|
{
|
|
$this->pdf=pdf_new();
|
|
pdf_open_file($this->pdf,null);
|
|
|
|
//calculate this now, becauasae aparently we cant calculated up top in the class definition
|
|
$this->content_width=$this->page_width-($this->page_margin*2);
|
|
|
|
//open up the first page
|
|
//Letter size (8.5 x 11) is 612,792
|
|
// pdf_begin_page($this->pdf,612,792);
|
|
// pdf_set_parameter($this->pdf, "FontOutline", "Arial=/home/sfiab/www.sfiab.ca/sfiab/arial.ttf");
|
|
//$arial=pdf_findfont($this->pdf,"Arial","host",1);
|
|
$this->normalfont=pdf_findfont($this->pdf,"Times-Roman","host",0);
|
|
$this->headerfont=pdf_findfont($this->pdf,"Times-Bold","host",0);
|
|
|
|
if(file_exists($logo))
|
|
$this->logoimage=pdf_open_image_file($this->pdf,"gif",$logo,"",0);
|
|
|
|
pdf_set_info($this->pdf,"Author","SFIAB");
|
|
pdf_set_info($this->pdf,"Creator","SFIAB");
|
|
pdf_set_info($this->pdf,"Title","SFIAB - $subheader");
|
|
pdf_set_info($this->pdf,"Subject","$subheader");
|
|
|
|
$this->page_header=$header;
|
|
$this->page_subheader=$subheader;
|
|
$this->pagenumber=0;
|
|
|
|
|
|
//add the stuff to the first page
|
|
// $this->addHeaderAndFooterToPage();
|
|
}
|
|
}
|
|
?>
|