forked from science-ation/science-ation
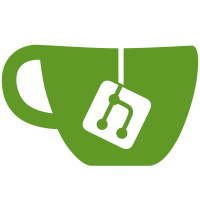
Fix special awards tab to load&save properly Fix volunteer positions tab to load&save properly
165 lines
4.4 KiB
PHP
165 lines
4.4 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
Copyright (C) 2007 David Grant <dave@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require_once("common.inc.php");
|
|
require_once("user.inc.php");
|
|
require_once("volunteer.inc.php");
|
|
|
|
// not sure if this needs to be retained for backwards compatability
|
|
if(array_key_exists('embed_edit_id', $_SESSION)){
|
|
$u = user_load($_SESSION['embed_edit_id']);
|
|
}else{
|
|
$u = user_load($_SESSION['users_id']);
|
|
}
|
|
|
|
if($_GET['action']=="save"){
|
|
$vals = '';
|
|
print_r($_POST);
|
|
if(is_array($_POST['posn'])) {
|
|
|
|
/* Load available IDs */
|
|
$posns = array();
|
|
$q = "SELECT * FROM volunteer_positions WHERE conferences_id='{$conference['id']}'";
|
|
$r = mysql_query($q);
|
|
while($p = mysql_fetch_object($r)) {
|
|
$posns[] = $p->id;
|
|
}
|
|
|
|
/* Match selections with available positions */
|
|
foreach($_POST['posn'] as $id=>$val) {
|
|
if(!in_array($id, $posns)) continue;
|
|
$vals[] = $id;
|
|
}
|
|
$u['volunteer_positions'] = $vals;
|
|
}
|
|
else {
|
|
//if they didnt select anything...
|
|
$u['volunteer_positions']=array();
|
|
}
|
|
user_save($u);
|
|
$newstatus = volunteer_status_position($u);
|
|
?>
|
|
<script type="text/javascript">
|
|
user_update_tab_status('volunteerpos','<?=$newstatus?>');
|
|
</script>
|
|
<?php
|
|
|
|
//message_push(notice(i18n("Volunteer Positions successfully updated")));
|
|
exit;
|
|
}
|
|
|
|
/* update overall status */
|
|
volunteer_status_update($u);
|
|
|
|
?>
|
|
|
|
<script type="text/javascript">
|
|
function volunteer_save()
|
|
{
|
|
$("#debug").load("<?=$config['SFIABDIRECTORY']?>/volunteer_position.php?action=save&users_id=<?=$u['id']?>", $("#volunteer_form").serializeArray());
|
|
return false;
|
|
}
|
|
|
|
$(document).ready(function() {
|
|
$("#volunteer_form").validate({
|
|
submitHandler: function() {
|
|
volunteer_save();
|
|
return false;
|
|
},
|
|
cancelHandler: function() {
|
|
volunteer_save();
|
|
return false;
|
|
}
|
|
});
|
|
|
|
user_update_tab_status('volunteerpos');
|
|
|
|
// $("#volunteer_form").valid();
|
|
});
|
|
</script>
|
|
|
|
<?php
|
|
|
|
echo "<div>";
|
|
display_messages();
|
|
echo "<h4>".i18n('Volunteer Positions')." - <span class=\"status_volunteerpos\"></span></h4>";
|
|
echo "</div>";
|
|
|
|
echo "<form id=\"volunteer_form\" class=\"editor\">\n";
|
|
echo "<table>\n";
|
|
|
|
/* Read current selections */
|
|
$checked_positions = array();
|
|
$r = mysql_query("SELECT * FROM volunteer_positions_signup WHERE users_id = '{$u['id']}' AND conferences_id='{$conference['id']}'");
|
|
while($p = mysql_fetch_object($r)) {
|
|
$checked_positions[] = $p->volunteer_positions_id;
|
|
}
|
|
|
|
/* Load available volunteer positions */
|
|
$q = "SELECT *,UNIX_TIMESTAMP(start) as ustart, UNIX_TIMESTAMP(end) as uend FROM volunteer_positions WHERE conferences_id = {$conference['id']}";
|
|
$r = mysql_query($q);
|
|
while($p = mysql_fetch_object($r)) {
|
|
|
|
echo '<tr><td>';
|
|
|
|
$checked = false;
|
|
|
|
if($_SESSION['lang'] == 'en') {
|
|
$sday = strftime("%a. %B %e, %Y", $p->ustart);
|
|
$stime = strftime("%H:%M", $p->ustart);
|
|
$eday = strftime("%a. %B %e, %Y", $p->uend);
|
|
$etime = strftime("%H:%M", $p->uend);
|
|
if($sday == $eday) {
|
|
$start = $stime;
|
|
$end = "$etime, $sday";
|
|
} else {
|
|
$start = "$sday, $stime";
|
|
$end = "$eday, $etime";
|
|
}
|
|
} else {
|
|
$start = $p->start;
|
|
$end = $p->end;
|
|
}
|
|
|
|
|
|
$ch = in_array($p->id, $checked_positions) ? 'checked="checked"' : '';
|
|
echo "<input $ch type=\"checkbox\" name=\"posn[$p->id]\" value=\"checked\" />";
|
|
|
|
echo '</td><td>';
|
|
echo '<b>'.i18n($p->name).'</b></td>' ;
|
|
|
|
echo "<td align=\"right\">($start - $end)</td></tr>";
|
|
echo '<tr><td></td><td colspan="2"><div style="font-size: 0.75em;">';
|
|
echo i18n($p->desc);
|
|
echo '<br /><br /></div></td></tr>';
|
|
}
|
|
|
|
echo "</table>";
|
|
echo "<input type=\"submit\" value=\"" . i18n("Save Information") . "\">";
|
|
echo "</form>";
|
|
|
|
echo "<br />";
|
|
?>
|