forked from science-ation/science-ation
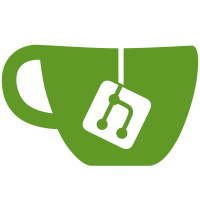
Fix tableeditor css to only modify anything in the tableeditor/tableview classes Fix saving language for volunteers Fix error message for saving phone numbers on organization and personal Fix schoolfeedback and schoolinfo pages Update school select tab, better html and school options list Fix css for tertiary menu to show difference between selected and non-selected
167 lines
4.7 KiB
PHP
167 lines
4.7 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
Copyright (C) 2007 David Grant <dave@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require_once('common.inc.php');
|
|
require_once('user.inc.php');
|
|
require_once('user_edit.inc.php');
|
|
|
|
/* Ensure they're logged in as something, anything */
|
|
user_auth_required();
|
|
|
|
$edit_id = isset($_GET['users_id']) ? intval($_GET['users_id']) : $_SESSION['users_id'];
|
|
if($edit_id != $_SESSION['users_id'])
|
|
user_auth_required('admin');
|
|
else
|
|
user_auth_required();
|
|
|
|
$u = user_load($edit_id);
|
|
|
|
|
|
/* Load the fields the user can edit, and theones that are required */
|
|
$fields = array();
|
|
$required = array();
|
|
foreach(array_keys($u['roles']) as $r) {
|
|
$fields = array_merge($fields, user_fields_enabled($r));
|
|
$required = array_merge($required, user_fields_required($r));
|
|
}
|
|
|
|
/* Filter fields, only the ones we care about */
|
|
$our_fields = array('organization', 'phonework','fax');
|
|
$fields = array_intersect($our_fields, $fields);
|
|
$required = array_intersect($our_fields, $required);
|
|
|
|
switch($_GET['action']) {
|
|
case 'save':
|
|
$save = true;
|
|
/* Set values */
|
|
foreach($fields as $f) {
|
|
$u[$f] = stripslashes($_POST[$f]);
|
|
/* Allow the user to clear a field regardless of regex */
|
|
if($u[$f] == '') continue;
|
|
|
|
}
|
|
|
|
if($save == true) {
|
|
user_save($u);
|
|
happy_("Organization information successfully updated");
|
|
}
|
|
|
|
//reload the user record because we dont know if we saved or didnt save above, we just want
|
|
//to know what the user looks like _now_
|
|
// $u = user_load($u['id']);
|
|
$newstatus=user_organization_status($u);
|
|
?>
|
|
<script type="text/javascript">
|
|
user_update_tab_status('organization','<?=$newstatus?>');
|
|
</script>
|
|
<?
|
|
exit;
|
|
}
|
|
|
|
?>
|
|
<h4><?=i18n("Organization")?> - <span class="status_organization"></span></h4>
|
|
<br/>
|
|
|
|
<form class="editor" id="orgform">
|
|
|
|
<table width="90%">
|
|
<tr><td style="text-align: left" colspan="2"><b><?=i18n('Organization')?></b><hr /></td></tr>
|
|
<tr><?=user_edit_item($u, 'Organization Name', 'organization')?></tr>
|
|
<tr><?=user_edit_item($u, 'Phone', 'phonework')?></tr>
|
|
<tr><?=user_edit_item($u, 'Fax', 'fax')?></tr>
|
|
</table>
|
|
|
|
<br />
|
|
<input type="submit" value="<?=i18n("Save Organization Information")?>" />
|
|
</form>
|
|
<br />
|
|
|
|
<?
|
|
function vreq($field)
|
|
{
|
|
global $required;
|
|
/* Return 'true' or 'false' as text for the
|
|
* validator plugin to use for the 'required' param */
|
|
if(in_array($field, $required)) return 'true';
|
|
return 'false';
|
|
}
|
|
?>
|
|
|
|
<script type="text/javascript">
|
|
|
|
function org_save()
|
|
{
|
|
$("#debug").load("<?=$config['SFIABDIRECTORY']?>/user_organization.php?action=save&users_id=<?=$edit_id?>", $("#orgform").serializeArray());
|
|
return false;
|
|
}
|
|
|
|
/* This method from the form validator additional methods script, modified to not
|
|
* allow spaces or parentheses */
|
|
jQuery.validator.addMethod("phoneUS", function(phone_number, element) {
|
|
phone_number = phone_number.replace(/\s+/g, "");
|
|
return this.optional(element) || phone_number.length > 9 &&
|
|
phone_number.match(/^[2-9]\d{2}-[2-9]\d{2}-\d{4}$/);
|
|
}, "Please specify a valid phone number (NNN-NNN-NNNN)");
|
|
|
|
$(document).ready(function() {
|
|
$("#orgform").validate({
|
|
rules: {
|
|
organization: { required: <?=vreq('firstname')?> },
|
|
phonework: {
|
|
required: <?=vreq('phonework')?>,
|
|
phoneUS: true
|
|
},
|
|
fax: {
|
|
required: <?=vreq('fax')?>,
|
|
phoneUS: true
|
|
}
|
|
},
|
|
messages: {
|
|
organization: { required: "<?=i18n('Please enter your company\'s name')?>" },
|
|
phonehome: {
|
|
required: "<?=i18n('Please enter your work phone number')?>",
|
|
phoneUS: "<?=i18n('Please enter a valid phone number of the form (NNN-NNN-NNNN)')?>"
|
|
},
|
|
phonecell: {
|
|
required: "<?=i18n('Please enter your work fax number')?>",
|
|
phoneUS: "<?=i18n('Please enter a valid phone number of the form (NNN-NNN-NNNN)')?>"
|
|
}
|
|
},
|
|
submitHandler: function() {
|
|
org_save();
|
|
return false;
|
|
},
|
|
cancelHandler: function() {
|
|
org_save();
|
|
return false;
|
|
}
|
|
});
|
|
|
|
user_update_tab_status('organization');
|
|
});
|
|
</script>
|
|
|
|
|