forked from science-ation/science-ation
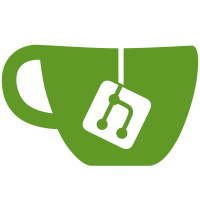
Make queries for communications easier, all you need is a users.id queried, the system will find everything else for you Add ability to use [PASSWORD], [USERNAME], [EMAIL] (tries accounts.email first, if its not there, it uses accounts.pendingemail), in _ANY_ email. [REGNUM] also added but will obviously only work for participants Add "all" section to the tabs list for user editor, so a user without any roles can still get the basic pages like "account", "roles", and "personal info" Put count on participant invitations for teachers (and superusers) Fix a bug where changing a password for a different user didnt work (it changed YOURS!)
319 lines
8.3 KiB
PHP
319 lines
8.3 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require_once('common.inc.php');
|
|
require_once('user.inc.php');
|
|
require_once('user_edit.inc.php');
|
|
require_once('judge.inc.php');
|
|
require_once('volunteer.inc.php');
|
|
require_once('activities.inc.php');
|
|
|
|
$edit_id = isset($_GET['users_id']) ? intval($_GET['users_id']) : $_SESSION['users_id'];
|
|
if($edit_id != $_SESSION['users_id'])
|
|
user_auth_required('admin');
|
|
else
|
|
user_auth_required();
|
|
|
|
if(array_key_exists('join', $_GET)){
|
|
|
|
// this is a request to join this conference
|
|
// get the corresponding account id to go with this user id
|
|
if(!$edit_id) {
|
|
// this will happen if they have no user record
|
|
$edit_accounts_id = $_SESSION['accounts_id'];
|
|
}else{
|
|
$q = mysql_query("SELECT accounts_id FROM users WHERE id='$edit_id'");
|
|
if(!$q){
|
|
echo mysql_error();
|
|
exit();
|
|
}
|
|
$result = mysql_fetch_assoc($q);
|
|
$edit_accounts_id = $result['accounts_id'];
|
|
}
|
|
|
|
// find out if they're already a member of this conference
|
|
$query = "SELECT COUNT(*) FROM users WHERE conferences_id = {$_SESSION['conferences_id']}"
|
|
. " AND accounts_id = " . $edit_accounts_id;
|
|
$data = mysql_fetch_array(mysql_query($query));
|
|
if($data[0] == 0){
|
|
// apparently not - let's go ahead and hook them up
|
|
$u = user_create($edit_accounts_id, $_SESSION['conferences_id']);
|
|
user_save($u);
|
|
$edit_id = $u['id'];
|
|
}
|
|
else {
|
|
$u=user_load_by_accounts_id($edit_accounts_id);
|
|
$edit_id=$u['id'];
|
|
//echo "we have {$data[0]} users already";
|
|
}
|
|
}else{
|
|
$joinConference = false;
|
|
if($edit_id == ''){
|
|
// the account in question does not have a users record
|
|
$joinConference = true;
|
|
}else{
|
|
$query = "SELECT COUNT(*) FROM users WHERE conferences_id = {$_SESSION['conferences_id']} AND ";
|
|
$query .= "id = $edit_id";
|
|
$data = mysql_fetch_array(mysql_query($query));
|
|
if($data[0] == 0){
|
|
// the user exists, but they are not linked to this conference
|
|
$joinConference = true;
|
|
}
|
|
}
|
|
|
|
if($joinConference){
|
|
send_header(i18n("User Editor").": {$u['name']}");
|
|
echo '<a href="user_edit.php?join=' . $_SESSION['conferences_id'] . '">' . i18n('Join this conferenece') . '</a>';
|
|
exit;
|
|
}
|
|
}
|
|
|
|
require_once("user_edit_tabs.inc.php");
|
|
|
|
$u = user_load($edit_id);
|
|
if(!$u) {
|
|
echo "Could not load user id $edit_id";
|
|
exit;
|
|
}
|
|
$types = array_keys($u['roles']);
|
|
$selected = $_GET['tab'];
|
|
if(!array_key_exists($selected, $tabs)) {
|
|
if(in_array('fair', $types) )
|
|
$selected = 'fairinfo';
|
|
else
|
|
$selected = '';
|
|
}
|
|
$types[]="all";
|
|
|
|
$fields = array();
|
|
$required = array();
|
|
foreach(array_keys($u['roles']) as $r) {
|
|
$fields = array_merge($fields, user_fields_enabled($r));
|
|
$required = array_merge($required, user_fields_required($r));
|
|
}
|
|
|
|
/* Disable some of the tabs */
|
|
if($config['judges_availability_enable'] != 'yes') $tabs['judgeavailability']['disabled'] = true;
|
|
$a = array_intersect(array('organization','phonework','fax'), $fields);
|
|
if(count($a) == 0) {
|
|
/* No organization stuff is enabled */
|
|
$tabs['organization']['disabled'] = true;
|
|
}
|
|
|
|
|
|
send_header($u['name']);
|
|
|
|
/* Setup tabs */
|
|
echo '<div id="tabs">';
|
|
echo '<ul>';
|
|
/* Always show a registration summary */
|
|
echo '<li><a href="#user_summary">'.i18n('Registration Summary').'</a></li>';
|
|
$index = 1;
|
|
$selected_index = 0;
|
|
/* Show all other enabled tabs */
|
|
foreach($tabs as $k=>$t) {
|
|
/* Make sure the tab is enabled */
|
|
if($t['disabled'] == true) continue;
|
|
|
|
// Make sure the tab is applicable to this conference
|
|
if(array_key_exists('conference_types', $t)){
|
|
if(!in_array($conference['type'], $t['conference_types'])){
|
|
continue;
|
|
}
|
|
}
|
|
|
|
/* Make sure the user has the right type to see the tab */
|
|
$i = array_intersect($t['types'], $types);
|
|
if(count($i) == 0) {
|
|
/* Turn off the tab, so in future iterations of the tabs
|
|
* list we only ahve to check enabled */
|
|
$tabs[$k]['disabled'] = true;
|
|
continue;
|
|
}
|
|
|
|
$tabs[$k]['index'] = $index;
|
|
$tabs_key[$index] = $k;
|
|
|
|
if($k == $selected) $selected_index = $index;
|
|
$index++;
|
|
|
|
/* Show the tab */
|
|
$href = "{$t['file']}?id=$edit_id";
|
|
echo "<li><a href=\"$href\"><span>".i18n($t['label'])."</span></a></li>";
|
|
}
|
|
|
|
?>
|
|
</ul>
|
|
<div id="user_summary">
|
|
|
|
<h4><?=i18n("Registration Summary")?></h4>
|
|
<br/>
|
|
|
|
<table border="0" cellpadding="2">
|
|
<?
|
|
foreach($tabs as $k=>$t) {
|
|
/* Enabled has been modified now for this user */
|
|
if($t['disabled'] == true) continue;
|
|
|
|
if($t['status_func'] == false) {
|
|
/* No status func, skip in the "todo list" */
|
|
continue;
|
|
}
|
|
|
|
// Make sure the tab is applicable to this conference
|
|
if(array_key_exists('conference_types', $t)){
|
|
if(!in_array($conference['type'], $t['conference_types'])){
|
|
continue;
|
|
}
|
|
}
|
|
|
|
/* Get the status */
|
|
if(is_callable($t['status_func'])) {
|
|
$s = call_user_func($t['status_func'], &$u);
|
|
$tabs[$k]['status'] = ($s == 'complete') ? 'complete' : 'incomplete';
|
|
} else {
|
|
$tabs[$k]['status'] = 'incomplete';
|
|
}
|
|
|
|
/* Link to switch to the tab */
|
|
$n = ($t['name'] != '') ? $t['name'] : $t['label'];
|
|
?>
|
|
<tr>
|
|
<td><a href="#" onclick="return linkto_click(<?=$t['index']?>);"><?=i18n($n)?></a></td>
|
|
<td><span class="status_<?=$k?>"></span></td>
|
|
</tr>
|
|
<?
|
|
}
|
|
?>
|
|
<tr><td colspan="2"><hr /></td></tr>
|
|
<tr> <td><?=i18n('Overall Status')?></td>
|
|
<td><span class="status_overall"></span></td>
|
|
</tr>
|
|
</table>
|
|
|
|
<br />
|
|
<br />
|
|
<b>Other Options and Things To Do:</b><br />
|
|
<ul>
|
|
<?
|
|
foreach($tabs as $k=>$t) {
|
|
/* Enabled has been modified now for this user */
|
|
if($t['disabled'] == true) continue;
|
|
|
|
if($t['status_func'] != false) {
|
|
/* Now we want all the other links not part of the status */
|
|
continue;
|
|
}
|
|
/* Link to switch to the tab */
|
|
$n = ($t['name'] != '') ? $t['name'] : $t['label'];
|
|
?>
|
|
<li><a href="#" onclick="return linkto_click(<?=$t['index']?>);"><?=i18n($n)?></a></li>
|
|
<?
|
|
}
|
|
?>
|
|
</ul>
|
|
|
|
|
|
</div>
|
|
|
|
</div>
|
|
|
|
<script type="text/javascript">
|
|
|
|
var stat = new Array();
|
|
<?
|
|
/* An array of all tabs and current status for each one */
|
|
$tally = 0;
|
|
foreach($tabs as $k=>$t) {
|
|
/* Enabled has been modified now for this user */
|
|
if($t['disabled'] == true) continue;
|
|
if($tally != 0) echo ", ";
|
|
$tally++;
|
|
echo "stat['$k'] = '{$t['status']}'\n";
|
|
}
|
|
?>
|
|
|
|
$(document).ready(function() {
|
|
$("#tabs").tabs({
|
|
selected: <?=$selected_index?>
|
|
});
|
|
|
|
/* Update each tab for complete/incomplete */
|
|
for(var key in stat) {
|
|
user_update_tab_status(key);
|
|
}
|
|
});
|
|
|
|
function linkto_click(index)
|
|
{
|
|
$("#tabs").tabs('select', index);
|
|
return false;
|
|
}
|
|
|
|
function user_update_tab_status(tabkey,newstatus)
|
|
{
|
|
// var curr_tab = $("#tabs").tabs('option', 'selected');
|
|
if(!newstatus) {
|
|
/* Keep the status the same if not specified */
|
|
newstatus = stat[tabkey];
|
|
}
|
|
if(newstatus!='complete') {
|
|
$(".status_"+tabkey).html('<?=i18n("Incomplete")?>');
|
|
$(".status_"+tabkey).removeClass('happy');
|
|
$(".status_"+tabkey).addClass('error');
|
|
} else {
|
|
$(".status_"+tabkey).html('<?=i18n("Complete")?>');
|
|
$(".status_"+tabkey).removeClass('error');
|
|
$(".status_"+tabkey).addClass('happy');
|
|
}
|
|
stat[tabkey] = newstatus;
|
|
|
|
/* See if the user is overall complete, server-side this is done, but the
|
|
* server never sends back the overall result to the client, and doesn't need to
|
|
* because we can easily figure it out (assuming the complete/incomplete status
|
|
* of each tab doesn't get out of sync) */
|
|
var overall = 'complete';
|
|
for(var key in stat) {
|
|
if(stat[key] != '' && stat[key] != 'complete') {
|
|
overall = 'incomplete';
|
|
}
|
|
}
|
|
if(overall!='complete') {
|
|
$(".status_overall").html('<?=i18n("Incomplete")?>');
|
|
$(".status_overall").removeClass('happy');
|
|
$(".status_overall").addClass('error');
|
|
} else {
|
|
$(".status_overall").html('<?=i18n("Complete")?>');
|
|
$(".status_overall").removeClass('error');
|
|
$(".status_overall").addClass('happy');
|
|
}
|
|
}
|
|
|
|
|
|
</script>
|
|
|
|
<?
|
|
send_footer();
|
|
?>
|