forked from science-ation/science-ation
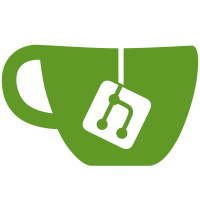
Make queries for communications easier, all you need is a users.id queried, the system will find everything else for you Add ability to use [PASSWORD], [USERNAME], [EMAIL] (tries accounts.email first, if its not there, it uses accounts.pendingemail), in _ANY_ email. [REGNUM] also added but will obviously only work for participants Add "all" section to the tabs list for user editor, so a user without any roles can still get the basic pages like "account", "roles", and "personal info" Put count on participant invitations for teachers (and superusers) Fix a bug where changing a password for a different user didnt work (it changed YOURS!)
380 lines
11 KiB
PHP
380 lines
11 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
Copyright (C) 2007 David Grant <dave@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require_once("common.inc.php");
|
|
require_once("account.inc.php");
|
|
|
|
/* Make sure the user is logged in with just an account (accounts_id is set),
|
|
* dont' call user_auth_required because they may not have a user */
|
|
if(!isset($_SESSION['accounts_id'])) {
|
|
message_push(error(i18n("You must login to view that page")));
|
|
header("location: {$config['SFIABDIRECTORY']}/index.php");
|
|
exit;
|
|
}
|
|
|
|
/* Superuser may edit this for any account, if the user is not a superuser, force
|
|
* the accounts_id to be whatever is in the session */
|
|
if($_SESSION['superuser']) {
|
|
if($_GET['accounts_id']) {
|
|
$accounts_id = intval($_GET['accounts_id']);
|
|
} else if($_GET['users_id']) {
|
|
$u=user_load(intval($_GET['users_id']));
|
|
$accounts_id=$u['accounts_id'];
|
|
}
|
|
else
|
|
$accounts_id = $_SESSION['accounts_id'];
|
|
} else {
|
|
$accounts_id = $_SESSION['accounts_id'];
|
|
}
|
|
|
|
function user_account_check_username($accounts_id, $username)
|
|
{
|
|
if(!account_valid_user($username)) return false;
|
|
|
|
$u = mysql_real_escape_string($username);
|
|
$q = mysql_query("SELECT id FROM accounts WHERE username='$u' AND deleted='no' AND id!=$accounts_id");
|
|
if(mysql_num_rows($q) != 0) return false;
|
|
|
|
return true;
|
|
}
|
|
|
|
switch($_GET['action']) {
|
|
case 'check_username':
|
|
$x = user_account_check_username($accounts_id, $_GET['username']);
|
|
echo json_encode(array('valid' => $x));
|
|
exit;
|
|
|
|
case 'save':
|
|
$a = account_load($accounts_id);
|
|
|
|
/* Since we're using input validation we dont' have to report errors back to the user, the validator
|
|
* should catch them all, so we'll just go ahead and save (or error out) */
|
|
// debug_(print_r($_POST), true);
|
|
|
|
$email = trim($_POST['email']);
|
|
$username_link = ($_POST['username_link'] == 'yes') ? true : false;
|
|
$username = $username_link ? $email : trim($_POST['username']);
|
|
|
|
if(array_key_exists('email', $_POST)) {
|
|
/* If this key doesn't exist, don't even try to update the email or the usename, the
|
|
* user is in a "must date their password" mode */
|
|
if($a['email'] != $email && $email != '') {
|
|
$save = true;
|
|
/* Change email */
|
|
if(!isEmailAddress($email)) {
|
|
error_('Invalid email address');
|
|
$save = false;
|
|
}
|
|
|
|
if($save) {
|
|
account_set_email($accounts_id,$email);
|
|
happy_("An email has been sent to %1 to confirm the new email address", array($email));
|
|
}
|
|
}
|
|
|
|
/* Update link */
|
|
$x = ($a['link_username_to_email'] == 'yes') ? true : false;
|
|
if($x != $username_link) {
|
|
$l = $username_link ? 'yes' : 'no';
|
|
mysql_query("UPDATE accounts SET link_username_to_email='$l' WHERE id=$accounts_id");
|
|
}
|
|
|
|
/* Update username */
|
|
if($a['username'] != $username) {
|
|
if(user_account_check_username($accounts_id, $username)) {
|
|
/* Update it */
|
|
$u = mysql_real_escape_string($username);
|
|
mysql_query("UPDATE accounts SET username='$u' WHERE id=$accounts_id");
|
|
happy_("Username updated");
|
|
}
|
|
}
|
|
}
|
|
|
|
$pass1 = $_POST['pass1'];
|
|
$pass2 = $_POST['pass2'];
|
|
if($pass1!='' || $pass2!='') {
|
|
$pass = mysql_escape_string($pass1);
|
|
//first, lets see if they choose the same password again (bad bad bad)
|
|
$q=mysql_query("SELECT password FROM accounts WHERE
|
|
id='$accounts_id' AND password='$pass'");
|
|
|
|
$save = false;
|
|
/* All of this, except matching the previous password, is checked
|
|
* by the form validator */
|
|
if(mysql_num_rows($q))
|
|
error_("You cannot choose the same password again. Please choose a different password");
|
|
else if($pass1 == '')
|
|
error_("New Password is required");
|
|
else if($pass1 != $pass2)
|
|
error_("Passwords do not match");
|
|
else if(account_valid_password($pass1) == false)
|
|
error_("The password contains invalid characters or is not long enough");
|
|
else {
|
|
account_set_password($accounts_id, $pass);
|
|
unset($_SESSION['password_expired']);
|
|
|
|
happy_('Password has been successfully updated');
|
|
}
|
|
}
|
|
/* Forward to the request_uri if it's set */
|
|
if(isset($_SESSION['request_uri'])) {
|
|
$link = $_SESSION['request_uri'];
|
|
unset($_SESSION['request_uri']);
|
|
?>
|
|
<script type="text/javascript">
|
|
window.document.location="<?=$link?>";
|
|
</script>
|
|
<?
|
|
}
|
|
|
|
exit;
|
|
}
|
|
|
|
// send_header("Account Information",
|
|
// array("Main" => "user_main.php")
|
|
// ,"change_password"
|
|
// );
|
|
|
|
$a = account_load($accounts_id);
|
|
|
|
$d = '';
|
|
$username_link = ($a['link_username_to_email'] == 'yes') ? 'checked="checked"' : '';
|
|
|
|
if($_SESSION['password_expired'] == true) {
|
|
echo error(i18n('Your password has expired. You must choose a new password now.'));
|
|
$d = 'disabled="disabled"';
|
|
$validator_passreq = 'required: true,';
|
|
echo "drect to: {$_SESSION['request_uri']}";;
|
|
}
|
|
|
|
?>
|
|
<h4><?=i18n("Account/Login Information")?></h4>
|
|
<br />
|
|
|
|
<form class="editor" name="account" id="accountform">
|
|
<table width="90%">
|
|
<tr>
|
|
<td style="text-align: left" colspan="2"><b>Email</b><hr /></td>
|
|
</tr>
|
|
<tr>
|
|
<td><label for="email"><?=i18n('Email')?>:</label></td>
|
|
<td><input id="email" <?=$d?> name="email" type="text" size="30" value="<?=$a['email']?>"></td>
|
|
</tr>
|
|
<? if($a['pendingemail']) {
|
|
?>
|
|
<tr>
|
|
<td><label for="email"><?=i18n('Pending Email')?>:</label></td>
|
|
<td><?=$a['pendingemail']?> is currently pending confirmation</td>
|
|
</tr>
|
|
<?
|
|
}
|
|
?>
|
|
<tr>
|
|
<td></td>
|
|
<td>
|
|
<div style="font-size: 0.75em;"><?=i18n('Changing the email address will cause a confirmation email to be sent to the new email address before the change will take effect.')?></div>
|
|
<br />
|
|
</td>
|
|
</tr>
|
|
<tr>
|
|
<td style="text-align: left" colspan="2"><b>Username</b><hr /></td>
|
|
</tr><tr>
|
|
<td><?=i18n('Username')?>:</td>
|
|
<td> <input <?=$ud?> <?=$d?> id="username" name=username type="text" size="20" value="<?=$a['username']?>"><br />
|
|
<input id="username_link" <?=$username_link?> <?=$d?> type="checkbox" name="username_link" value="yes" />
|
|
<?=i18n('Use the email address as the login username')?><br />
|
|
|
|
</td>
|
|
</tr>
|
|
<tr><td colspan="2"><br /></td></tr>
|
|
<tr>
|
|
<td style="text-align: left" colspan="2"><b>Password</b><hr /></td>
|
|
</tr>
|
|
<?
|
|
if($_SESSION['superuser']=="yes") {
|
|
?>
|
|
<tr>
|
|
<td><label for="pass1"><?=i18n('Current Password')?>:</label></td>
|
|
<td><? echo account_get_password($a['id']); ?></td>
|
|
</tr>
|
|
<?
|
|
}
|
|
?>
|
|
<tr>
|
|
<td><label for="pass1"><?=i18n('New Password')?>:</label></td>
|
|
<td><input id="pass1" name="pass1" type="password" size="20" value=""></td>
|
|
</tr>
|
|
<tr>
|
|
<td><label for="pass2"><?=i18n('Confirm New Password')?>:</label></td>
|
|
<td><input id="pass2" name="pass2" type="password" size="20" value=""></td>
|
|
</tr>
|
|
<tr>
|
|
<td></td>
|
|
<td>
|
|
<div style="font-size: 0.75em;"><?=i18n('Passwords must be be between 6 and 32 characters, and may NOT contain any quote or a backslash.')?></div>
|
|
</td>
|
|
</tr>
|
|
</table>
|
|
<br />
|
|
<br />
|
|
<input type="submit" value="<?=i18n("Save")?>" />
|
|
</form>
|
|
|
|
|
|
<br />
|
|
|
|
<script type="text/javascript">
|
|
var username_valid = true;
|
|
var username_checking = true;
|
|
var check_username_time = false;
|
|
|
|
function username_changed()
|
|
{
|
|
username_checking = false;
|
|
username_valid = true;
|
|
|
|
/* Immediately go to checking... */
|
|
$("#accountform").validate().element( "#username" );
|
|
$("#accountform").validate().element( "#email" );
|
|
|
|
if(check_username_time != false)
|
|
clearTimeout(check_username_time);
|
|
check_username_time = setTimeout(function() {
|
|
var username = $("#username").val();
|
|
username_checking = false;
|
|
$.getJSON("<?=$config['SFIABDIRECTORY']?>/user_account.php?action=check_username&accounts_id=<?=$accounts_id?>&username="+username,
|
|
function(json){
|
|
username_valid = (json.valid == 1) ? true : false;
|
|
username_checking = true;
|
|
$("#accountform").validate().element( "#username" );
|
|
$("#accountform").validate().element( "#email" );
|
|
});
|
|
}, 500);
|
|
|
|
}
|
|
|
|
function email_changed() {
|
|
if($("#username_link").is(":checked")) {
|
|
$("#username").val($('#email').val());
|
|
username_changed();
|
|
}
|
|
}
|
|
|
|
|
|
$.validator.addMethod("username_in_use",function(value, element) {
|
|
if(element.id == 'username') {
|
|
return username_valid;
|
|
} else {
|
|
if($("#username_link").is(":checked"))
|
|
return username_valid;
|
|
else
|
|
return true;
|
|
}
|
|
});
|
|
|
|
$.validator.addMethod("checking",function(value, element) {
|
|
return username_checking;
|
|
});
|
|
|
|
$(document).ready(function() {
|
|
$("#accountform").validate({
|
|
rules: {
|
|
email: {
|
|
required: true,
|
|
email: true,
|
|
username_in_use: true,
|
|
},
|
|
username: {
|
|
// required: "#username_link:checked",
|
|
username_in_use: true,
|
|
checking: true,
|
|
minlength: 4
|
|
},
|
|
pass1: {
|
|
<?=$validator_passreq?>
|
|
minlength: 6,
|
|
maxlength: 32
|
|
},
|
|
pass2: {
|
|
<?=$validator_passreq?>
|
|
minlength: 6,
|
|
maxlength: 32,
|
|
equalTo: "#pass1"
|
|
}
|
|
},
|
|
messages: {
|
|
email: {
|
|
required: "Please enter an email address",
|
|
email: "Please enter a valid email address",
|
|
username_in_use: "Email aready in use as a username, use a different email, or uncheck the username box below"
|
|
},
|
|
username: {
|
|
required: "Please enter a username",
|
|
minlength: "Your username must consist of at least 2 characters",
|
|
username_in_use: "Username is taken, please choose a different one",
|
|
checking: "Checking..."
|
|
},
|
|
pass1: {
|
|
required: "Please enter a password",
|
|
minlength: "Your password must be at least 6 characters long",
|
|
maxlength: "Your password must be at most 32 characters long"
|
|
},
|
|
pass2: {
|
|
required: "Please confirm the password",
|
|
minlength: "Your password must be at least 6 characters long",
|
|
maxlength: "Your password must be at most 32 characters long",
|
|
equalTo: "Please enter the same password as above"
|
|
}
|
|
},
|
|
submitHandler: function() {
|
|
$("#debug").load("user_account.php?action=save&accounts_id=<?=$accounts_id?>", $("#accountform").serializeArray());
|
|
}
|
|
});
|
|
|
|
|
|
<? if($_SESSION['password_expired'] == false) { ?>
|
|
/* Code to disable the username box, only included if the password hasn't expired */
|
|
var username_link = $("#username_link").is(":checked");
|
|
$("#username").attr("disabled", username_link);
|
|
$("#username_link").click(function() {
|
|
$("#username").attr("disabled", this.checked);
|
|
email_changed();
|
|
username_changed();
|
|
});
|
|
$("#email").change(email_changed);
|
|
$("#email").keyup(email_changed);
|
|
$("#username").change(username_changed);
|
|
$("#username").keyup(username_changed);
|
|
|
|
<? } ?>
|
|
|
|
});
|
|
</script>
|
|
|
|
|
|
<?
|
|
//send_footer();
|
|
?>
|