forked from science-ation/science-ation
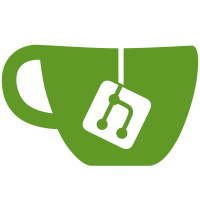
Fix tableeditor css to only modify anything in the tableeditor/tableview classes Fix saving language for volunteers Fix error message for saving phone numbers on organization and personal Fix schoolfeedback and schoolinfo pages Update school select tab, better html and school options list Fix css for tertiary menu to show difference between selected and non-selected
301 lines
8.8 KiB
PHP
301 lines
8.8 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2010 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require("../common.inc.php");
|
|
require("../user.inc.php");
|
|
superuser_required();
|
|
|
|
$level=0;
|
|
|
|
function doTree($id,$roleid,$ctype) {
|
|
global $level;
|
|
global $config;
|
|
|
|
$level++;
|
|
$q=mysql_query("SELECT * FROM rolestasks WHERE pid=$id AND roles_id='$roleid' AND conferencetype='".mysql_real_escape_string($ctype)."' ORDER BY ord");
|
|
echo "<ul class=\"rolelist\">";
|
|
while($r=mysql_fetch_object($q)) {
|
|
echo "<li class=\"roletask\" id=\"rolestasks_{$r->id}\">";
|
|
//can only delete if there's no children
|
|
$tq=mysql_query("SELECT * FROM rolestasks WHERE pid='$r->id' AND conferencetype='".mysql_real_escape_string($ctype)."'");
|
|
if(mysql_num_rows($tq)==0) {
|
|
echo "<a href=\"#\" onclick=\"return removeTask($r->id)\"><img src=\"{$config['SFIABDIRECTORY']}/images/16/button_cancel.png\" style=\"border: 0px;\"></a> ";
|
|
}
|
|
echo "<a href=\"#\" onclick=\"return editTask($r->id)\"><img src=\"{$config['SFIABDIRECTORY']}/images/16/edit.png\" style=\"border: 0px;\"></a> ";
|
|
echo "<span id=\"task_name_{$r->id}\">";
|
|
echo "$r->task";
|
|
echo "</span>\n";
|
|
echo " ";
|
|
echo "[";
|
|
echo "<span id=\"task_link_{$r->id}\">";
|
|
if($r->link) {
|
|
echo trim($r->link);
|
|
}
|
|
echo "</span>";
|
|
echo "]";
|
|
|
|
echo " ";
|
|
echo "«";
|
|
echo "<span id=\"task_navident_{$r->id}\">";
|
|
if($r->navident) {
|
|
echo trim($r->navident);
|
|
}
|
|
echo "</span>";
|
|
echo "»";
|
|
|
|
echo " ";
|
|
echo "<span id=\"task_save_{$r->id}\"></span>";
|
|
|
|
echo "</li>\n";
|
|
doTree($r->id,$roleid,$ctype);
|
|
}
|
|
$level--;
|
|
if($level<3) {
|
|
echo "<li class=\"roletask\" id=\"emptytasks_{$id}\"><form onsubmit=\"return addTask($id)\">";
|
|
echo "<input type=\"text\" name=\"newtask[$id]\" id=\"newtask_{$id}\">";
|
|
echo "<input type=\"submit\" value=\"Add\">";
|
|
// echo "<input type=\"button\" value=\"Add\" onclick=\"addTask($id)\">";
|
|
echo "</form></li>";
|
|
}
|
|
echo "</ul>";
|
|
}
|
|
|
|
if($_GET['action']=="load") {
|
|
if($_GET['roleid']) {
|
|
$roleid=intval($_GET['roleid']);
|
|
$ctype=trim($_GET['ctype']);
|
|
doTree(0,$roleid,$ctype);
|
|
}
|
|
exit;
|
|
}
|
|
|
|
if($_POST['action']=="addtask") {
|
|
$pid=intval($_POST['pid']);
|
|
$roleid=intval($_POST['roleid']);
|
|
$ctype=trim($_POST['ctype']);
|
|
if($pid==0)
|
|
$level=0;
|
|
else {
|
|
$q=mysql_query("SELECT * FROM rolestasks WHERE id='$pid' AND conferencetype='".mysql_real_escape_string($ctype)."'");
|
|
$r=mysql_fetch_object($q);
|
|
$level=$r->level+1;
|
|
}
|
|
|
|
$q=mysql_query("SELECT MAX(ord) AS o FROM rolestasks WHERE pid='$pid' AND conferencetype='".mysql_real_escape_string($ctype)."'");
|
|
$r=mysql_fetch_object($q);
|
|
$ord=$r->o+1;
|
|
|
|
mysql_query("INSERT INTO rolestasks (pid,roles_id,level,task,ord,link,conferencetype) VALUES (
|
|
'$pid',
|
|
'$roleid',
|
|
'$level',
|
|
'".mysql_real_escape_string($_POST['task'])."',
|
|
'$ord',
|
|
'',
|
|
'".mysql_real_escape_string($ctype)."'
|
|
)");
|
|
echo mysql_error();
|
|
|
|
exit;
|
|
}
|
|
if($_POST['action']=="removetask") {
|
|
$id=intval($_POST['id']);
|
|
//can only delete if there's no children
|
|
$tq=mysql_query("SELECT * FROM rolestasks WHERE pid='$id'");
|
|
if(mysql_num_rows($tq)==0) {
|
|
mysql_query("DELETE FROM rolestasks WHERE id='$id'");
|
|
}
|
|
exit;
|
|
}
|
|
|
|
if($_POST['action']=="savetask") {
|
|
$id=intval($_POST['id']);
|
|
mysql_query("UPDATE rolestasks SET
|
|
task='".mysql_real_escape_string($_POST['name'])."',
|
|
link='".mysql_real_escape_string($_POST['link'])."',
|
|
navident='".mysql_real_escape_string($_POST['navident'])."'
|
|
WHERE id='$id'");
|
|
exit;
|
|
}
|
|
|
|
if($_POST['action']=="movetask") {
|
|
|
|
list($junk,$dragid)=explode("_",$_POST['dragid']);
|
|
list($junk,$dropid)=explode("_",$_POST['dropid']);
|
|
if($junk=="emptytasks") {
|
|
//we actually want to drop it AFTER the last one in this set based on PID, not ID
|
|
$q=mysql_query("SELECT * FROM rolestasks WHERE pid='$dropid' AND id!='$dragid' ORDER BY ord");
|
|
$droppid=$dropid;
|
|
$dropid=100000;
|
|
}
|
|
else {
|
|
$dropq=mysql_query("SELECT * FROM rolestasks WHERE id='$dropid'");
|
|
$dropr=mysql_fetch_object($dropq);
|
|
$q=mysql_query("SELECT * FROM rolestasks WHERE pid='$dropr->pid' AND id!='$dragid' ORDER BY ord");
|
|
$droppid=$dropr->pid;
|
|
}
|
|
if($dragid) {
|
|
$dragq=mysql_query("SELECT * FROM rolestasks WHERE id='$dragid'");
|
|
$dragr=mysql_fetch_object($dragq);
|
|
$ord=0;
|
|
$updated=false;
|
|
echo mysql_error();
|
|
while($r=mysql_fetch_object($q)) {
|
|
echo "comparing {$r->id} with {$dropid} \n";
|
|
$droplevel=$r->level;
|
|
|
|
if($r->id==$dropid) {
|
|
$ord++;
|
|
//dropped on something, so put it at the same level as what we dropped
|
|
mysql_query("UPDATE rolestasks SET pid='$droppid', level='$droplevel', ord='$ord' WHERE id='$dragid'");
|
|
echo "found!";
|
|
$updated=true;
|
|
}
|
|
$ord++;
|
|
mysql_query("UPDATE rolestasks SET ord='$ord' WHERE id='$r->id'");
|
|
}
|
|
if(!$updated) {
|
|
echo "not found, putting it at the end";
|
|
$ord++;
|
|
mysql_query("UPDATE rolestasks SET pid='$droppid', level='$droplevel', ord='$ord' WHERE id='$dragid'");
|
|
}
|
|
echo mysql_error();
|
|
}
|
|
exit;
|
|
}
|
|
|
|
send_header("RoleTasks Setup");
|
|
?>
|
|
<br />
|
|
NOT intended for end-user usage. This tool exists only temporarily for developers to update the new UI structure
|
|
<br />
|
|
<style type="text/css">
|
|
.drop-below {
|
|
border-top: 2px solid black;
|
|
}
|
|
.roletask {
|
|
cursor: pointer;
|
|
}
|
|
</style>
|
|
|
|
<script type="text/javascript">
|
|
var roleid=0;
|
|
var ctype='';
|
|
function roleChange(o) {
|
|
roleid=o.value;
|
|
reloadTasks();
|
|
}
|
|
|
|
function typeChange(o) {
|
|
ctype=o.value;
|
|
reloadTasks();
|
|
}
|
|
|
|
function reloadTasks() {
|
|
if(roleid && ctype) {
|
|
$("#list").load("roletasks.php?action=load&roleid="+roleid+"&ctype="+ctype,null,function() {
|
|
$(".roletask").draggable({revert: 'invalid'});
|
|
$(".roletask").droppable({
|
|
hoverClass: 'drop-below',
|
|
drop: function(event, ui) {
|
|
$(this).addClass('ui-state-highlight');
|
|
var dragid=ui.draggable.attr('id');
|
|
var dropid=this.id;
|
|
$.post("roletasks.php",{action: 'movetask', dragid: dragid, dropid: dropid}, function() {
|
|
reloadTasks();
|
|
});
|
|
}
|
|
})
|
|
});
|
|
}
|
|
}
|
|
|
|
function addTask(id) {
|
|
var v=$("#newtask_"+id).val();
|
|
$.post("roletasks.php",{action: 'addtask', task: v, pid: id, roleid: roleid, ctype: ctype}, function() {
|
|
reloadTasks();
|
|
});
|
|
return false;
|
|
}
|
|
function removeTask(id) {
|
|
$.post("roletasks.php",{action: 'removetask', id: id}, function() {
|
|
reloadTasks();
|
|
});
|
|
return false;
|
|
}
|
|
function editTask(id) {
|
|
var v_link=$("#task_link_"+id).html();
|
|
var v_name=$("#task_name_"+id).html();
|
|
var v_navident=$("#task_navident_"+id).html();
|
|
|
|
$("#task_navident_"+id).html("<input type=\"text\" size=\"30\" value=\""+v_navident+"\" name=\"edit_task_navident\" id=\"edit_task_navident_"+id+"\">");
|
|
$("#task_link_"+id).html("<input type=\"text\" size=\"30\" value=\""+v_link+"\" name=\"edit_task_link\" id=\"edit_task_link_"+id+"\">");
|
|
$("#task_name_"+id).html("<input type=\"text\" size=\"30\" value=\""+v_name+"\" name=\"edit_task_name\" id=\"edit_task_name_"+id+"\">");
|
|
$("#task_save_"+id).html("<input type=\"button\" name=\"editlink_save\" value=\"Save\" onclick=\"saveTask("+id+")\">");
|
|
return false;
|
|
}
|
|
|
|
function saveTask(id) {
|
|
var v_link=$("#edit_task_link_"+id).val();
|
|
var v_name=$("#edit_task_name_"+id).val();
|
|
var v_navident=$("#edit_task_navident_"+id).val();
|
|
|
|
$("#task_save_"+id).html("");
|
|
|
|
$.post("roletasks.php",{action: 'savetask', id: id, link: v_link, name: v_name, navident: v_navident},function() {
|
|
$("#task_link_"+id).html(v_link);
|
|
$("#task_name_"+id).html(v_name);
|
|
$("#task_navident_"+id).html(v_navident);
|
|
});
|
|
|
|
}
|
|
|
|
</script>
|
|
<?
|
|
|
|
$q=mysql_query("SELECT * FROM roles ORDER BY name");
|
|
echo "<form method=\"get\" action=\"roletasks.php\" id=\"roleform\" name=\"roleform\">";
|
|
echo "<select name=\"rid\" onchange=\"roleChange(this)\">";
|
|
echo "<option value=\"\">Choose a role</option>\n";
|
|
while($r=mysql_fetch_object($q)) {
|
|
echo "<option value=\"$r->id\">$r->name</option>\n";
|
|
}
|
|
echo "</select>\n";
|
|
|
|
$types=array("sciencefair"=>"Science Fair", "scienceolympics"=>"Science Olympics");
|
|
|
|
echo "<select name=\"ctype\" onchange=\"typeChange(this)\">";
|
|
echo "<option value=\"\">Choose a type</option>\n";
|
|
foreach($types AS $t=>$tn) {
|
|
echo "<option value=\"$t\">$tn</option>\n";
|
|
}
|
|
echo "</select>\n";
|
|
echo "</form>\n";
|
|
|
|
|
|
echo "<div id=\"list\">";
|
|
echo "</div>";
|
|
|
|
send_footer();
|
|
?>
|