forked from science-ation/science-ation
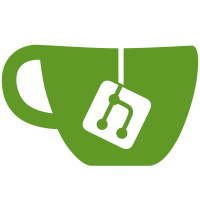
Added compvars.php, which is a test script for comparing data after it's been rolled over from one conference to another - used to test the rollover in super/conferences.php. Updated usage of the role "student", replacing it with "participant".
128 lines
4.6 KiB
PHP
Executable File
128 lines
4.6 KiB
PHP
Executable File
#!/usr/bin/php
|
|
<?
|
|
/*
|
|
This is a test script to compare the data that is stored for two conferences.
|
|
It's raison d'etre is to ensure that the new conference rollover process is
|
|
working properly.
|
|
*/
|
|
|
|
require_once("../common.inc.php");
|
|
if($_SERVER['DOCUMENT_ROOT'] != "") {
|
|
echo "This script must be run from a shell";
|
|
exit();
|
|
}
|
|
|
|
if($argc != 3 || !is_numeric($argv[1]) || !is_numeric($argv[2])){
|
|
echo "This script is used to compare the variables stored for two separate conferences.\n";
|
|
echo "\tUsage:\n\tcompvars confid1 confid2\n";
|
|
exit();
|
|
}
|
|
|
|
$confid1 = $argv[1];
|
|
$confid2 = $argv[2];
|
|
|
|
compTable('config', array('var'), array('val', 'category', 'type'));
|
|
compTable('dates', array('name'), array());
|
|
compTable('award_types', array('id'), array('type', 'order'));
|
|
compTable('pagetext', array('textname'), array('textdescription', 'text'));
|
|
compTable('projectcategories', array('id', 'category'), array('category_shortform', 'mingrade', 'maxgrade'));
|
|
compTable('projectdivisions', array('id', 'division'), array('division_shortform', 'cwsfdivisionid'));
|
|
compTable('projectsubdivisions', array('id'), array('subdivision', 'projectdivisions_id'));
|
|
compTable('safetyquestions', array('ord'), array('question', 'type', 'required'));
|
|
compTable('award_awards', array('order'), array());
|
|
compTable('award_types', array('type'), array('order'));
|
|
//compTable('award_awards_projectcategories', array('award_awards_id', 'projectcategories_id'), array());
|
|
//compTable('award_awards_projectdivisions', array('award_awards_id', 'projectdivisions_id'), array());
|
|
//compTable('award_prizes JOIN award_awards ON award_awards.id = award_prizes.award_awards_id', array('order', 'prize'), array());
|
|
compTable('schools', array('accesscode'), array('school', 'address', 'postalcode'));
|
|
compTable('questions', array('ord', 'db_heading'), array('section', 'question', 'type'));
|
|
compTable('regfee_items', array('name'), array());
|
|
compTable('volunteer_positions', array('name'), array('start', 'end', 'desc', 'meet_place'));
|
|
compTable('judges_timeslots', array('name'), array());
|
|
|
|
|
|
function compTable($tableName, $keys, $fields){
|
|
global $confid1, $confid2;
|
|
$selection = array_merge($fields, array_diff($keys, $fields));
|
|
|
|
// get data from our conferences
|
|
$data1 = selectDat($tableName, $selection, $keys, $confid1);
|
|
$data2 = selectDat($tableName, $selection, $keys, $confid2);
|
|
|
|
echo "\n --- Comparing the table '$tableName' for conferences $confid1 and $confid2, checking field(s): {" . implode(', ', $fields) . "}, using keys {" . implode(':', $keys) . "} ---\n";
|
|
|
|
$keys1 = array_keys($data1);
|
|
$keys2 = array_keys($data2);
|
|
|
|
// show whether or not any key values are set in this table for one of these conferences but not the other
|
|
$keyDiff = array_diff($keys1, $keys2);
|
|
if(count($keyDiff) > 0){
|
|
echo "The following key values are used in conference #$confid1, but not conference #$confid2:\n";
|
|
echo implode(', ', $keyDiff) . "\n";
|
|
}else{
|
|
echo "conference #$confid2 has all of the key values used in conference #$confid1 in table $tableName.\n";
|
|
}
|
|
|
|
$keyDiff = array_diff($keys2, $keys1);
|
|
if(count($keyDiff) > 0){
|
|
echo "The following key values are used in conference #$confid2, but not conference #$confid1:\n";
|
|
echo implode(', ', $keyDiff) . "\n";
|
|
}else{
|
|
echo "conference #$confid1 has all of the key values used in conference #$confid2 in table $tableName.\n";
|
|
}
|
|
|
|
|
|
// now show if any of the records have different field values in the same key index
|
|
if(count($fields) > 0){
|
|
$saidMessage = false;
|
|
$commonkeys = array_intersect($keys1, $keys2);
|
|
$numfields = count($fields);
|
|
foreach($commonkeys as $key){
|
|
$badFields = array();
|
|
for($n = 0; $n < $numfields; $n++){
|
|
if($data1[$key][$fields[$n]] != $data2[$key][$fields[$n]]){
|
|
$badFields[] = $fields[$n];
|
|
}
|
|
}
|
|
if(count($badFields) > 0){
|
|
if(!$saidMessage){
|
|
echo "The following records have different values for the same keys:\n";
|
|
$saidMessage = true;
|
|
}
|
|
|
|
echo "$key : ";
|
|
foreach($badFields as $fieldName){
|
|
echo "$fieldName: ({$data1[$key][$fieldName]} != {$data2[$key][$fieldName]}) ";
|
|
}
|
|
echo "\n";
|
|
}
|
|
}
|
|
|
|
if(!$saidMessage){
|
|
echo "All common key names have matching values for these two sets.\n";
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
function selectDat($tableName, $selection, $keys, $confid){
|
|
$query = "SELECT `" . implode('`,`', $selection) . "` FROM $tableName WHERE conferences_id = $confid";
|
|
$q = mysql_query($query);
|
|
$data = array();
|
|
|
|
if(mysql_error() != ''){
|
|
echo "$query\n" . mysql_error() . "\n";
|
|
print_r(debug_backtrace());
|
|
}
|
|
|
|
while($row = mysql_fetch_assoc($q)){
|
|
$keyVals = array();
|
|
foreach($keys as $k){
|
|
$keyVals[] = $row[$k];
|
|
unset($row[$k]);
|
|
}
|
|
$data[implode(':', $keyVals)] = $row;
|
|
}
|
|
return $data;
|
|
}
|