forked from science-ation/science-ation
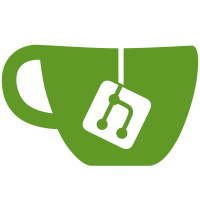
Add min/max judges/volunteers/teams Add defaults Update science olympic tab display Add Teams/Volunteers/Judges status to admin schedule view
507 lines
15 KiB
PHP
507 lines
15 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require("common.inc.php");
|
|
require_once("schedule.inc.php");
|
|
require_once("user.inc.php");
|
|
|
|
user_auth_required("teacher");
|
|
$schoolid=user_field_required("schools_id","user_edit.php?tab=school");
|
|
|
|
$q=mysql_query("SELECT * FROM schools WHERE id='".$schoolid."'");
|
|
echo mysql_error();
|
|
$school=mysql_fetch_object($q);
|
|
if(!$school) exit;
|
|
|
|
if($_GET['action']=="loadschedule") {
|
|
$date = $_POST['date'];
|
|
if(!eregi("[0-9]{4}-[0-9]{2}-[0-9]{2}",$date)) {
|
|
echo "Invalid date";
|
|
exit;
|
|
}
|
|
|
|
$eventList = getEventList($conference['id'], $school->id);
|
|
|
|
// get the minimum and maximum hours from the schedule
|
|
$starthour = $endhour = null;
|
|
foreach($eventList as $event){
|
|
if($event['date'] == $date){
|
|
$sh = $event['hour'];
|
|
$eh = $sh + ceil($event['duration'] / 60);
|
|
if($endhour === null || $endhour < $eh) $endhour = $eh;
|
|
if($starthour === null || $starthour > $sh) $starthour = $sh;
|
|
}
|
|
}
|
|
|
|
//do some sanity checks
|
|
if($starthour<0 || $starthour>24) $starthour=8;
|
|
if($endhour<$starthour)
|
|
$endhour=$starthour+10;
|
|
if($endhour<0 || $endhour>24) $endhour=15;
|
|
|
|
//minute increment
|
|
$increment=15;
|
|
|
|
echo "<h3>".i18n("Schedule for %1",array(format_date($date)))."</h3>";
|
|
$q=mysql_query("SELECT * FROM locations WHERE conferences_id='{$conference['id']}' ORDER BY name");
|
|
while($r=mysql_fetch_object($q)) {
|
|
$locations[$r->id]=$r->name;
|
|
}
|
|
if(!count($locations)) {
|
|
echo error(i18n("There are no locations defined."));
|
|
exit;
|
|
}
|
|
|
|
echo "<table class=\"schedule\" id=\"schedule\">\n";
|
|
echo "<tr>";
|
|
echo "<th style=\"width: 50px;\"> </th>";
|
|
foreach($locations AS $id=>$name) {
|
|
echo " <th>$name</th>\n";
|
|
}
|
|
for($h=$starthour;$h<$endhour;$h++) {
|
|
for($m=0;$m<60;$m+=$increment) {
|
|
echo "<tr>";
|
|
echo " <td class=\"scheduletime\">";
|
|
if($m==0) {
|
|
echo format_time("$h:$m");
|
|
}
|
|
echo "</td>";
|
|
foreach($locations AS $id=>$name) {
|
|
echo "<td id=\"{$h}_{$m}_{$id}\"><div>";
|
|
echo "</div></td>";
|
|
}
|
|
echo "</tr>";
|
|
}
|
|
}
|
|
echo "</table>\n";
|
|
|
|
$js="var eventdivs=new Array();\n";
|
|
|
|
//now make all our DIV's for the events that are scheduled in the database
|
|
//they will be moved by javascript after the fact
|
|
if(is_array($eventList)){
|
|
foreach($eventList as $event){
|
|
// skip this event if it's not on the day we're looking at
|
|
if($event['date'] != $date) continue;
|
|
|
|
// some variables for convenience. Used repeatedly
|
|
$eid = $event['id'];
|
|
$hour = $event['hour'];
|
|
$minute = $event['minute'];
|
|
$location = $event['locations_id'];
|
|
$duration = $event['duration'];
|
|
$eventType = $event['eventtype'];
|
|
$title = $event['title'];
|
|
$starttime = strtotime($hour . ":" . $minute);
|
|
$endtime = $starttime + $duration * 60;
|
|
|
|
echo "<div class=\"scheduleevent scheduleevent_$eventType\" id=\"event_$eid\" onclick=\"viewEvent($eid)\">";
|
|
echo "<span class=\"scheduleevent_title\">";
|
|
echo $title;
|
|
echo "</span>";
|
|
echo "<br />";
|
|
echo format_time($starttime);
|
|
echo " to ";
|
|
echo format_time($endtime);
|
|
echo "<br />";
|
|
|
|
if($eventType == "scienceolympic") {
|
|
echo i18n("Capacity").": ";
|
|
$regteams = getNumRegistrations($eid);
|
|
$maxteams= $event['somaxteams'];
|
|
echo i18n("%1 of %2", array($regteams, $maxteams)) . "<br />";
|
|
foreach($event['registrations'] as $regr){
|
|
echo "<div style=\"padding: 2px; border: 1px solid black; background-color: yellow;\" onclick=\"handleTeamClick(event, {$regr['id']}, $eid);\">";
|
|
echo $regr['name'];
|
|
echo "</div>";
|
|
}
|
|
}
|
|
|
|
echo "</div>";
|
|
$js .= "eventdivs[$eid]={hour:$hour,minute:$minute,location:$location,duration:$duration,title:'".rawurlencode($title)."',eventtype:'$eventType'};\n";
|
|
}
|
|
}else{
|
|
// it's actually an error message
|
|
echo $eventList;
|
|
}
|
|
echo "<script type=\"text/javascript\">\n";
|
|
echo $js;
|
|
echo "</script>";
|
|
}else if($_GET['action']=="loadevent") {
|
|
|
|
}else if($_GET['action']=="saveevent") {
|
|
|
|
}else if($_GET['action'] == "saveteamlist"){
|
|
// print_r($_POST);
|
|
|
|
// get a list of all students that could be put on this team
|
|
$query =
|
|
"SELECT * FROM users"
|
|
. " JOIN user_roles ON user_roles.users_id = users.id"
|
|
. " JOIN roles ON roles.id = user_roles.roles_id"
|
|
. " WHERE schools_id = $schoolid"
|
|
. " AND conferences_id = {$conference['id']}"
|
|
. " AND deleted = 'no'"
|
|
. " AND roles.type = 'student'";
|
|
$availList = array();
|
|
while($record = mysql_fetch_array($results)){
|
|
$availList[$record['accounts_id']] = $record['firstname'] . ' ' . $record['lastname'];
|
|
}
|
|
|
|
// let's empty the current list of students for this team
|
|
$results=mysql_query("DELETE FROM schedule_registrations_users_link
|
|
WHERE users_uid IN (".implode(',', array_keys($availList)).")
|
|
AND schedule_registrations_id = ".intval($_POST['regId']));
|
|
|
|
// and now we'll insert only those that were selected
|
|
$query = "INSERT INTO schedule_registrations_users_link (schedule_registrations_id, users_uid)";
|
|
$query .= " VALUES ";
|
|
$valueSet = array();
|
|
for($n = 0; $n < $_POST['numSelected']; $n++){
|
|
$valueSet[] = '(' . (int)$_POST['regId'] . ',' . (int)$_POST[$n] .')';
|
|
}
|
|
$query .= implode(',', $valueSet);
|
|
$results = mysql_query($query);
|
|
|
|
}else if($_GET['action'] == "getteamlist"){
|
|
// first we'll get the basic info, and do a quick check that the selected team is in this user's domain
|
|
if(!array_key_exists('regId', $_POST)){
|
|
echo "error\n";
|
|
return;
|
|
}
|
|
$query = "SELECT sr.*, so_teams.name as name FROM schedule_registrations sr";
|
|
$query .= " JOIN so_teams ON so_teams.id = sr.so_teams_id";
|
|
$query .= " WHERE sr.id=" . $_POST['regId'];
|
|
$query .= " AND so_teams.schools_id = " . $schoolid;
|
|
|
|
$results = mysql_query($query);
|
|
if(!$results){
|
|
echo 'team not found';
|
|
return;
|
|
}
|
|
$record = mysql_fetch_array($results);
|
|
$teamName = $record['name'];
|
|
|
|
// get a list of all students that could be put on this team
|
|
|
|
$query =
|
|
"SELECT * FROM users"
|
|
. " JOIN user_roles ON user_roles.users_id = users.id"
|
|
. " JOIN roles ON roles.id = user_roles.roles_id"
|
|
. " WHERE schools_id = $schoolid"
|
|
. " AND conferences_id = {$conference['id']}"
|
|
. " AND deleted = 'no'"
|
|
. " AND roles.type = 'student'";
|
|
$results = mysql_query($query);
|
|
$availList = array();
|
|
while($record = mysql_fetch_array($results)){
|
|
$availList[$record['accounts_id']] = $record['firstname'] . ' ' . $record['lastname'];
|
|
}
|
|
|
|
// now find out which of these students are already selected
|
|
$query = "SELECT * FROM schedule_registrations_users_link srul";
|
|
$query .= " WHERE users_uid IN (";
|
|
$query .= implode(',', array_keys($availList)) . ')';
|
|
$query .= " AND schedule_registrations_id = " . $_POST['regId'];
|
|
echo "\n[[[ $query ]]]\n";
|
|
$results = mysql_query($query);
|
|
$selectedList = array();
|
|
if($results){
|
|
while($record = mysql_fetch_array($results)){
|
|
$idx = $record['users_uid'];
|
|
$selectedList[$idx] = $availList[$idx];
|
|
unset($availList[$idx]);
|
|
}
|
|
}
|
|
|
|
// get the necessary data about the event itself
|
|
$query = "SELECT sominteamsize, somaxteamsize, title FROM schedule JOIN"
|
|
. " schedule_registrations sr ON sr.schedule_id = schedule.id WHERE"
|
|
. " sr.id = " . $_POST['regId'];
|
|
$results = mysql_fetch_array(mysql_query($query));
|
|
$minTeamSize = $results['sominteamsize'];
|
|
$maxTeamSize = $results['somaxteamsize'];
|
|
$eventName = $results['title'];
|
|
|
|
// and let's put out the script to pass this data back to the browser
|
|
echo '<script type="text/javascript">';
|
|
echo "minTeamSize = $minTeamSize;";
|
|
echo "maxTeamSize = $maxTeamSize;";
|
|
$caption = i18n(
|
|
'Select team members for team "%1" in the event "%2"',
|
|
array(0 => $teamName, 1 => $eventName)
|
|
);
|
|
echo "caption = '$caption';";
|
|
// build our student lists
|
|
echo "available={";
|
|
$n = 0;
|
|
foreach($availList as $id => $name){
|
|
if($n > 0) echo ',';
|
|
echo "$id:\"$name\"";
|
|
$n++;
|
|
}
|
|
echo '};';
|
|
|
|
echo "selected={";
|
|
$n = 0;
|
|
foreach($selectedList as $id => $name){
|
|
if($n > 0) echo ',';
|
|
echo "$id:\"$name\"";
|
|
$n++;
|
|
}
|
|
echo '};';
|
|
|
|
// pass the additional parameters
|
|
/*
|
|
$query = "SELECT default_min_team_size, default_max_team_size FROM events WHERE id=" . $_POST['eventId'];
|
|
$results = mysql_fetch_array(mysql_query($query));
|
|
echo "min_size=" . $results['default_min_team_size'] . ';';
|
|
echo "max_size=" . $results['default_max_team_size'] . ';';
|
|
*/
|
|
echo '</script>';
|
|
}else{
|
|
|
|
send_header("Event Registration",
|
|
array('School Home' => 'schoolaccess.php'),
|
|
"events_scheduling" );
|
|
echo "<br />";
|
|
?>
|
|
<script type="text/javascript" src="js/listSelector.js"></script>
|
|
<script type="text/javascript">
|
|
|
|
$(document).ready(function() {
|
|
$(".date").datepicker({ dateFormat: 'yy-mm-dd' });
|
|
changeDate();
|
|
|
|
/* Setup the editor dialog */
|
|
$("#event_dialog").dialog({
|
|
bgiframe: true, autoOpen: false,
|
|
modal: true, resizable: false,
|
|
draggable: false,
|
|
buttons: {
|
|
"<?=i18n('Close')?>": function() {
|
|
$(this).dialog("close");
|
|
changeDate();
|
|
}
|
|
}
|
|
});
|
|
$(window).resize(function() {
|
|
placeEvents();
|
|
}
|
|
);
|
|
});
|
|
|
|
function handleTeamClick(event, regId, eventId){
|
|
var e = event || window.event;
|
|
e.stopPropagation();
|
|
params={
|
|
'regId' : regId,
|
|
'eventId' : eventId
|
|
};
|
|
|
|
$("#debug").load("schoolschedule.php?action=getteamlist", params, function(response){
|
|
// data returned will be:
|
|
// - selected: an array of students already assigned to this event
|
|
// - available: an array of students not assigned to this event
|
|
// - caption: text instructions briefly explaining the window
|
|
// - minTeamSize: the minimum allowable size for a team in the event
|
|
// - maxTeamSize: the maximum allowable size for a team in the event
|
|
|
|
// create a list selector that can use these lists
|
|
var n;
|
|
selector = new listSelector(selected, available, 'selector');
|
|
selector.setStyle({'height' : '70%', 'margin-top' : '7%'});
|
|
selector.leftTitle = '<?=i18n("assigned");?>';
|
|
selector.rightTitle = '<?=i18n("available");?>';
|
|
// highlight the lists appropriately
|
|
for(n = 0; n < minTeamSize; n++){
|
|
selector.leftColours[n] = '#AFA';
|
|
}
|
|
for(;n < maxTeamSize; n++){
|
|
selector.leftColours[n] = '#FFA';
|
|
}
|
|
// add our validator to limit the number that can be selected
|
|
selector.leftValidator = function(){
|
|
// note that in this case "this" refers to the selector
|
|
leftTally = 0;
|
|
for(var n in this.items){
|
|
if(this.items[n].data('column') == 'left'){
|
|
leftTally ++;
|
|
}
|
|
}
|
|
return leftTally < maxTeamSize;
|
|
}
|
|
|
|
// create the legend
|
|
var legend = $('<div></div>');
|
|
legend.append($('<strong><?=i18n("Legend")?>:</strong>'));
|
|
legend.append(' <span style="background-color:#AFA"><?=i18n('Required'); ?></span>');
|
|
legend.append(' <span style="background-color:#FFA"><?=i18n('Optional'); ?></span>');
|
|
legend.css({
|
|
'background-color' : '#FFF',
|
|
'display' : 'inline',
|
|
'border' : 'solid',
|
|
'border-width' : '1px',
|
|
'padding' : '5px',
|
|
'line-height': '2em',
|
|
});
|
|
legend = $('<div></div>').append(legend);
|
|
|
|
// now put all the parts together into a dialog
|
|
var sdialog = $('<div style="text-align:center"></div>');
|
|
sdialog.append(caption);
|
|
sdialog.append(selector.draw());
|
|
sdialog.append(legend);
|
|
sdialog.dialog({
|
|
title : '<?=i18n('Team Member Selector');?>',
|
|
width : 600,
|
|
height : 400,
|
|
resizable: false,
|
|
buttons: {
|
|
"<?=i18n("Save")?>": function() {
|
|
saveTeamList($(this), regId, eventId);
|
|
},
|
|
"<?=i18n('Close')?>": function() {
|
|
$(this).dialog("close");
|
|
changeDate();
|
|
}
|
|
}
|
|
});
|
|
});
|
|
}
|
|
|
|
// save the selected team members for the selected team in the selected event
|
|
function saveTeamList(ddiv, regId, eventId){
|
|
selectedList = selector.getLeftList();
|
|
params = {
|
|
'regId' : regId,
|
|
'eventId' : eventId,
|
|
'numSelected' : selectedList.length
|
|
};
|
|
|
|
for(var n in selectedList){
|
|
params[n] = selectedList[n];
|
|
}
|
|
|
|
$("#debug").load("schoolschedule.php?action=saveteamlist", params, function(response){
|
|
ddiv.dialog("close");
|
|
});
|
|
|
|
}
|
|
|
|
function changeDate() {
|
|
$("#schedulediv").load("schoolschedule.php?action=loadschedule",{date:$("#date").val()},function() {
|
|
placeEvents();
|
|
});
|
|
}
|
|
|
|
function placeEvents() {
|
|
$('.scheduleevent').each(function(idx,item) {
|
|
var eventid=item.id.substr(6);
|
|
var eventobj=eventdivs[eventid];
|
|
|
|
var tablecellid=eventobj.hour+'_'+eventobj.minute+'_'+eventobj.location;
|
|
|
|
if($("#"+tablecellid).length) {
|
|
var eheight=((eventobj.duration/15)*<?=$ROWHEIGHT?>)-<? echo $BORDERSIZE*2; ?>;
|
|
var ewidth=$("#"+tablecellid).width()-<? echo $BORDERSIZE; ?>;
|
|
var p=$("#"+tablecellid).offset();
|
|
$("#"+item.id).css(p);
|
|
$("#"+item.id).show();
|
|
$("#"+item.id).height(eheight);
|
|
$("#"+item.id).width(ewidth);
|
|
}
|
|
else {
|
|
$("#"+item.id).hide();
|
|
}
|
|
}
|
|
);
|
|
}
|
|
|
|
var scheduleId;
|
|
function viewEvent(id) {
|
|
scheduleId=id;
|
|
var eventobj=eventdivs[id];
|
|
|
|
if(id) {
|
|
var eventobj=eventdivs[id];
|
|
$("#event_dialog").dialog('option','title',unescape(eventobj.title));
|
|
$("#event_dialog").dialog('option','width',600);
|
|
$("#event_dialog").dialog('option','height',400);
|
|
$("#event_dialog").dialog('open');
|
|
$("#event_tabs").tabs('select',0);
|
|
|
|
if(eventobj.eventtype=='scienceolympic')
|
|
$("#event_tabs").tabs('option','disabled',[]);
|
|
else
|
|
$("#event_tabs").tabs('option','disabled',[1]);
|
|
update_tab_summary();
|
|
|
|
}
|
|
|
|
}
|
|
|
|
function saveEvent() {
|
|
var o=$("#edit_event_form").serializeArray();
|
|
//alert(o);
|
|
$("#debug").load("schoolschedule.php?action=saveevent",o,function() {
|
|
changeDate();
|
|
});
|
|
}
|
|
</script>
|
|
|
|
<?
|
|
$q=mysql_query("SELECT DISTINCT(date) AS date FROM schedule WHERE conferences_id='{$conference['id']}' ORDER BY date");
|
|
$dates=array();
|
|
while($r=mysql_fetch_object($q)) {
|
|
$dates[]=$r->date;
|
|
}
|
|
if(count($dates))
|
|
$editdate=$dates[0];
|
|
else
|
|
list($editdate,$bla)=explode(" ",$config['dates']['fairdate']);
|
|
|
|
echo "<form method=\"post\">\n";
|
|
echo i18n("Schedule date").": ";
|
|
echo "<select id=\"date\" name=\"date\" onchange=\"changeDate()\">";
|
|
foreach($dates AS $d) {
|
|
echo "<option value=\"$d\">".format_date($d)."</option>\n";
|
|
}
|
|
echo "</select>";
|
|
echo "</form>\n";
|
|
?>
|
|
<hr />
|
|
<div id="schedulediv">
|
|
</div>
|
|
<div id="event_dialog">
|
|
<? include "schoolschedule_event_dialog.php"; ?>
|
|
</div>
|
|
<div id="selector_dialog"></div>
|
|
<?
|
|
|
|
send_footer();
|
|
|
|
}
|
|
?>
|