forked from science-ation/science-ation
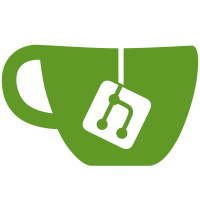
Make queries for communications easier, all you need is a users.id queried, the system will find everything else for you Add ability to use [PASSWORD], [USERNAME], [EMAIL] (tries accounts.email first, if its not there, it uses accounts.pendingemail), in _ANY_ email. [REGNUM] also added but will obviously only work for participants Add "all" section to the tabs list for user editor, so a user without any roles can still get the basic pages like "account", "roles", and "personal info" Put count on participant invitations for teachers (and superusers) Fix a bug where changing a password for a different user didnt work (it changed YOURS!)
280 lines
8.6 KiB
PHP
280 lines
8.6 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require_once('common.inc.php');
|
|
require_once('user.inc.php');
|
|
|
|
user_auth_required('teacher');
|
|
$schoolid=user_field_required("schools_id","user_edit.php?tab=school");
|
|
//include "judges.inc.php";
|
|
$type="participant";
|
|
|
|
/* AJAX query */
|
|
if(intval($_POST['ajax']) == 1) {
|
|
/* Do ajax processing for this file */
|
|
$email = mysql_real_escape_string($_POST['email']);
|
|
|
|
/* Sanity check type */
|
|
if(!array_key_exists($type, $roles)) {
|
|
echo "err\n";
|
|
exit;
|
|
}
|
|
|
|
//we use username='email' because if we are INVITING someone, then
|
|
//they pretty much have to user their email address as their username
|
|
//otherwise the system has no way to send them the details
|
|
$q = mysql_query("SELECT id,deleted FROM accounts WHERE username='$email' OR email='$email'");
|
|
if(mysql_num_rows($q) == 0) {
|
|
/* Account doesn't exist */
|
|
echo "notexist\n";
|
|
exit;
|
|
}
|
|
$account = mysql_fetch_assoc($q);
|
|
|
|
if($account['deleted'] == 'yes') {
|
|
echo "notexist\n";
|
|
exit;
|
|
}
|
|
|
|
$u = user_load_by_accounts_id($account['id']);
|
|
if(!$u) {
|
|
//user_load_by_accounts_id returns false if there is no user record for this account for this conference
|
|
echo "norole\n";
|
|
exit;
|
|
}
|
|
|
|
if(!array_key_exists($type, $u['roles'])) {
|
|
echo "norole\n";
|
|
exit;
|
|
}
|
|
|
|
if($u['conferences_id'] != $conference['id']) {
|
|
echo "noconference\n";
|
|
exit;
|
|
}
|
|
|
|
echo "exist\n";
|
|
exit;
|
|
}
|
|
|
|
//this is ajax too, but we dont explicitly say AJAX==1, thats silly :p
|
|
if($_POST['action']=="uninvite") {
|
|
if($_POST['userid']) {
|
|
echo "uninviting userid: ".$_POST['userid']." from type: ".$type."\n";
|
|
$r=user_uninvite(intval($_POST['userid']),$type);
|
|
//this will return the user object if valid, if its just a string, then its an error string
|
|
if(!is_array($r)) {
|
|
echo "error: $r";
|
|
}
|
|
}
|
|
exit;
|
|
}
|
|
|
|
|
|
|
|
|
|
send_header("Invite Participants",
|
|
array('Committee Main' => 'committee_main.php',
|
|
'Administration' => 'admin/index.php'
|
|
) );
|
|
|
|
?>
|
|
<script type="text/javascript">
|
|
var checkTimeout;
|
|
|
|
function checkEmailLater() {
|
|
clearTimeout(checkTimeout);
|
|
checkTimeout=setTimeout('checkEmail()',500);
|
|
}
|
|
function checkEmail() {
|
|
var url, email, role;
|
|
|
|
role = $("#role").val();
|
|
email = $("#email").val();
|
|
|
|
if(email.length < 3 || role == "") {
|
|
update_status("<?=i18n('Enter an email address')?>");
|
|
$("#button").attr('disabled',true);
|
|
$("#button").val('<?=i18n("Invite")?>');
|
|
return true;
|
|
}
|
|
update_status("Checking...");
|
|
$.post("participant_invite.php",{ajax: 1, email: email, role: role},function(d) {
|
|
var lines=d.split("\n");
|
|
var response=lines[0];
|
|
// alert(response);
|
|
switch(response) {
|
|
case "err":
|
|
update_status("<?=i18n('Enter an email address')?>");
|
|
$("#button").attr('disabled',true);
|
|
$("#button").val('<?=i18n("Invite")?>');
|
|
$("#action").val("err");
|
|
break;
|
|
case "notexist":
|
|
update_status("<?=i18n('User not found. Choose the \"Invite new participant\" button below to create an account for this user and send them an email invite.')?>");
|
|
$("#button").attr('disabled',false);
|
|
$("#button").val('<?=i18n("Invite new participant")?>');
|
|
$("#action").val("invitenew");
|
|
break;
|
|
case "norole":
|
|
update_status("<?=i18n('Account found without the selected role.<br />Choose the \"Invite existing account\" button below to add the participant role to this user\'s account and send them email notice of the change.')?>");
|
|
$("#button").attr('disabled',false);
|
|
$("#button").val('<?=i18n("Invite existing account to be a participant")?>');
|
|
$("#action").val("inviteexisting");
|
|
break;
|
|
case "exist":
|
|
update_status("<?=i18n('This user already exists as a participant. They cannot be invited.')?>");
|
|
$("#button").attr('disabled',true);
|
|
$("#button").val('<?=i18n("Cannot Invite")?>');
|
|
$("#action").val("err");
|
|
break;
|
|
}
|
|
|
|
});
|
|
return true;
|
|
}
|
|
|
|
function update_status(text) {
|
|
$("#status").html(text);
|
|
}
|
|
|
|
function uninvite(id) {
|
|
if(confirmClick('Are you sure you want to uninvite this participant?')) {
|
|
$.post("participant_invite.php",{action: 'uninvite', userid: id},function() {
|
|
// alert('done');
|
|
document.location.href='participant_invite.php';
|
|
});
|
|
}
|
|
}
|
|
|
|
</script>
|
|
<?
|
|
|
|
echo "<br />";
|
|
|
|
if( ($_POST['action']=="invitenew" || $_POST['action']=="inviteexisting") && trim($_POST['email']) && $type != '') {
|
|
$newUser=user_invite(trim($_POST['email']), null, trim($_POST['email']), $type);
|
|
if(is_array($newUser)) {
|
|
echo happy(i18n("%1 successfully invited to be a %2",array(trim($_POST['email']),$type)));
|
|
}
|
|
else {
|
|
echo error($newUser);
|
|
}
|
|
}
|
|
|
|
echo "<form method=\"post\" name=\"invite\" action=\"participant_invite.php\">\n";
|
|
echo "<input type=\"hidden\" name=\"action\" id=\"action\" value=\"invite\" />\n";
|
|
echo "<table>";
|
|
/*
|
|
echo "<tr><td>";
|
|
echo i18n("Select a Role: ");
|
|
echo "</td><td><select id=\"role\" name=\"type\" onChange=\"checkEmail();\">\n";
|
|
echo "<option value=\"\" >".i18n('Choose')."</option>\n";
|
|
$sel = ($type == 'judge') ? 'selected="selected"' : '';
|
|
echo "<option value=\"judge\" $sel >".i18n('Judge')."</option>\n";
|
|
$sel = ($type == 'volunteer') ? 'selected="selected"' : '';
|
|
echo "<option value=\"volunteer\" $sel >".i18n('Volunteer')."</option>\n";
|
|
echo "</select></td></tr>";
|
|
*/
|
|
echo "<tr><td>";
|
|
echo i18n("Enter an Email: ");
|
|
echo "</td><td><input type=\"text\" id=\"email\" name=\"email\" size=\"40\" onKeyUp=\"checkEmailLater();\" />";
|
|
echo "</td></tr></table>";
|
|
echo "<br />\n";
|
|
echo "<div class=\"notice\" id=\"status\">".i18n('Enter an email address')."</div>";
|
|
|
|
echo "<br />\n";
|
|
echo "<input id=\"button\" type=\"submit\" disabled=\"disabled\" value=\"".i18n("Invite")."\" />\n";
|
|
|
|
echo "</form>\n";
|
|
|
|
if($_SESSION['superuser']=="yes") {
|
|
$q=mysql_query("SELECT users.id, users.firstname, users.lastname, accounts.username, accounts.email, accounts.pendingemail, users.schools_id, schools.school, schools.conferences_id
|
|
FROM users
|
|
LEFT JOIN schools ON users.schools_id=schools.id
|
|
JOIN accounts on users.accounts_id=accounts.id
|
|
JOIN user_roles ON user_roles.users_id=users.id
|
|
JOIN roles ON user_roles.roles_id=roles.id
|
|
AND users.conferences_id='{$conference['id']}'
|
|
AND roles.type='participant'");
|
|
}
|
|
else {
|
|
$q=mysql_query("SELECT users.id, users.firstname, users.lastname, accounts.username, accounts.email, accounts.pendingemail FROM users
|
|
JOIN accounts on users.accounts_id=accounts.id
|
|
JOIN user_roles ON user_roles.users_id=users.id
|
|
JOIN roles ON user_roles.roles_id=roles.id
|
|
WHERE schools_id='$schoolid'
|
|
AND conferences_id='{$conference['id']}'
|
|
AND roles.type='participant'");
|
|
}
|
|
|
|
echo mysql_error();
|
|
echo "<br />";
|
|
echo "<br />";
|
|
if($_SESSION['superuser']=="yes") {
|
|
echo "<h2>".i18n("The following %1 participants have been invited from all schools (you're a superuser!)",array(mysql_num_rows($q)))."</h2>\n";
|
|
}
|
|
else {
|
|
echo "<h2>".i18n("The following %1 participants have been invited from your school",array(mysql_num_rows($q)))."</h2>\n";
|
|
}
|
|
echo "<table class=\"tableview\">\n";
|
|
echo "<thead>";
|
|
echo "<tr><th>Username</th><th>Email Address</th><th>First Name</th><th>Last Name</th>";
|
|
if($_SESSION['superuser']=="yes") {
|
|
echo "<th>School</th>";
|
|
}
|
|
echo "<th>Actions</th>";
|
|
echo "</tr>\n";
|
|
echo "</thead>";
|
|
echo "<tbody>";
|
|
while($r=mysql_fetch_object($q)) {
|
|
echo "<tr>";
|
|
echo " <td>$r->username</td>";
|
|
echo " <td>";
|
|
if($r->email) {
|
|
echo "$r->email";
|
|
}
|
|
else if($r->pendingemail) {
|
|
echo "<i>$r->pendingemail</i>";
|
|
}
|
|
echo "</td>";
|
|
echo " <td>$r->firstname</td>";
|
|
echo " <td>$r->lastname</td>";
|
|
if($_SESSION['superuser']=="yes") {
|
|
echo "<td>$r->school";
|
|
if($r->conferences_id!=$conference['id'] && $r->schools_id)
|
|
echo error("invalid school for this conference");
|
|
echo "</td>";
|
|
}
|
|
|
|
echo " <td><a href=\"#\" onclick=\"return uninvite($r->id)\">uninvite</a></td>";
|
|
echo "</tr>";
|
|
|
|
}
|
|
echo "</tbody>";
|
|
echo "</table>\n";
|
|
|
|
send_footer();
|
|
?>
|