forked from science-ation/science-ation
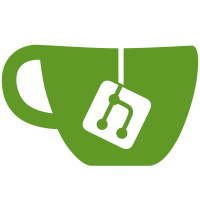
Modified user code to properly save the volunteer availability data Updated the UI to properly use the user_save function to update judge and volunteer info
209 lines
6.7 KiB
PHP
209 lines
6.7 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require_once('common.inc.php');
|
|
require_once('user.inc.php');
|
|
require_once('judge.inc.php');
|
|
require_once("questions.inc.php");
|
|
require_once('user_edit.inc.php');
|
|
|
|
/* Ensure they're logged in as a judge or admin */
|
|
user_auth_required(array(), array('judge','admin'));
|
|
|
|
$edit_id = isset($_GET['users_id']) ? intval($_GET['users_id']) : $_SESSION['users_id'];
|
|
if($edit_id != $_SESSION['users_id'])
|
|
user_auth_required('admin');
|
|
else
|
|
user_auth_required();
|
|
|
|
$u = user_load($edit_id);
|
|
|
|
// Load the judging rounds
|
|
$times = get_judging_timeslots($conference['id']);
|
|
|
|
switch($_GET['action']) {
|
|
case 'save':
|
|
if(!is_array($_POST['languages'])) $_POST['languages']=array();
|
|
|
|
$u['languages'] = array();
|
|
foreach($_POST['languages'] AS $val)
|
|
$u['languages'][] = $val;
|
|
|
|
$u['special_award_only'] = ($_POST['special_award_only'] == 'yes') ? 'yes' : 'no';
|
|
$u['willing_chair'] = ($_POST['willing_chair'] == 'yes') ? 'yes' : 'no';
|
|
$u['years_school'] = intval($_POST['years_school']);
|
|
$u['years_regional'] = intval($_POST['years_regional']);
|
|
$u['years_national'] = intval($_POST['years_national']);
|
|
$u['highest_psd'] = stripslashes($_POST['highest_psd']);
|
|
if(is_array($_POST['time'])){
|
|
$u['available_times'] = $_POST['time'];
|
|
}else if(array_key_exists('available_times', $u)){
|
|
$u['available_times'] = array();
|
|
}
|
|
user_save($u);
|
|
|
|
if(is_array($_POST['questions'])){
|
|
questions_save_answers("judgereg",$u['id'],$_POST['questions']);
|
|
}
|
|
happy_("Preferences successfully saved");
|
|
|
|
$u = user_load($u['id']);
|
|
$newstatus=judge_status_other($u);
|
|
?>
|
|
<script type="text/javascript">
|
|
user_update_tab_status('judge','<?=$newstatus?>');
|
|
</script>
|
|
<?
|
|
exit;
|
|
}
|
|
|
|
$fields = array('languages[]', 'years_school','years_regional','years_national','willing_chair','highest_psd','time[]');
|
|
$required = array('languages[]');
|
|
|
|
if(count($times) > 1) $required[] = 'time[]';
|
|
|
|
?>
|
|
<h4><?=i18n("Judge Information")?> - <span class="status_judge"></span></h4>
|
|
<br/>
|
|
<form class="editor" id="judgeother_form">
|
|
<table width="90%">
|
|
<tr><td style="text-align: left" colspan="2"><b><?=i18n('Judging Language(s)')?></b><hr /></td></tr>
|
|
<tr><?=user_edit_item($u, 'Languages', 'languages[]', 'languages')?></tr>
|
|
|
|
<tr><td style="text-align: left" colspan="2"><br /><b><?=i18n('Judging Experience')?></b><hr />
|
|
<i><?=i18n('Please specify the number of years you\'ve judged at the following levels. This helps balance the experience on judging teams.')?></i>
|
|
</td></tr>
|
|
|
|
<tr><?=user_edit_item($u, 'Judge at a School/District Fair', 'years_school', 'textbox', 5)?></tr>
|
|
<tr><?=user_edit_item($u, 'Judge at a Regional Fair', 'years_regional', 'textbox', 5)?></tr>
|
|
<tr><?=user_edit_item($u, 'Judge at a National Fair', 'years_national', 'textbox', 5)?></tr>
|
|
|
|
<? if(count($times) > 1) { ?>
|
|
<tr><td style="text-align: left" colspan="2"><br /><b><?=i18n('Time Availability')?></b><hr />
|
|
<i><?=i18n('Please specify the time(s) you are available to judge. You will be scheduled to judge in ALL (not just one) judging timeslot.')?></i>
|
|
</td></tr>
|
|
|
|
<?
|
|
/* Get all their available times */
|
|
foreach($times as $slot){
|
|
$options[$slot['id']] = trim($slot['name'] . ' (' . $slot['date'] . ' ' . $slot['starttime'] . ' - ' . $slot['endtime'] . ')');
|
|
}
|
|
echo '<tr>';
|
|
user_edit_item($u, 'Time Availability', 'time[]', 'checklist', $options, $u['available_times']);
|
|
echo '</tr>';
|
|
}
|
|
?>
|
|
|
|
<tr><td style="text-align: left" colspan="2"><br /><b><?=i18n('Judging Questions')?></b><hr /></td></tr>
|
|
<tr><?=user_edit_item($u, 'I am willing to be the lead for my judging team', 'willing_chair', 'yesno')?></tr>
|
|
<tr><?=user_edit_item($u, 'Highest post-secondary degree', 'highest_psd', 'textbox')?></tr>
|
|
|
|
<?
|
|
questions_print_answer_editor('judgereg', $u, 'questions');
|
|
?>
|
|
</table>
|
|
<br />
|
|
<button><?=i18n("Save Information")?></button>
|
|
</form>
|
|
|
|
<script type="text/javascript">
|
|
|
|
function judgeother_save()
|
|
{
|
|
$("#debug").load("<?=$config['SFIABDIRECTORY']?>/judge_other.php?action=save&users_id=<?=$u['id']?>", $("#judgeother_form").serializeArray());
|
|
return false;
|
|
}
|
|
|
|
$(document).ready(function() {
|
|
$("#judgeother_form").validate({
|
|
errorPlacement: function(error, element) {
|
|
if( element.attr('type') == 'checkbox' ) {
|
|
error.insertAfter( element.parent("span") );
|
|
} else {
|
|
error.insertAfter(element);
|
|
}
|
|
|
|
},
|
|
|
|
rules: {
|
|
"languages[]": { required: true },
|
|
"time[]": { required: <?=in_array('time[]', $required)?'true':'false'?> },
|
|
years_school: { min: 0, max:100 },
|
|
years_regional: { min: 0, max:100 },
|
|
years_national: { min: 0, max:100 }
|
|
},
|
|
|
|
messages: {
|
|
"languages[]": { required: "<?=i18n('Please select the language(s) you can judge in')?>" },
|
|
"time[]": { required: "<?=i18n('Please select the time(s) you are available for judging')?>" },
|
|
years_school: {
|
|
min: "<?=i18n('Please enter a valid number')?>",
|
|
max: "<?=i18n('Please enter a valid number')?>",
|
|
},
|
|
years_regional: {
|
|
min: "<?=i18n('Please enter a valid number')?>",
|
|
max: "<?=i18n('Please enter a valid number')?>",
|
|
},
|
|
years_national: {
|
|
min: "<?=i18n('Please enter a valid number')?>",
|
|
max: "<?=i18n('Please enter a valid number')?>",
|
|
}
|
|
},
|
|
submitHandler: function() {
|
|
judgeother_save();
|
|
return false;
|
|
},
|
|
cancelHandler: function() {
|
|
judgeother_save();
|
|
return false;
|
|
}
|
|
});
|
|
|
|
user_update_tab_status('judge');
|
|
|
|
// $("#judgeother_form").valid();
|
|
});
|
|
|
|
</script>
|
|
|
|
<?
|
|
/*
|
|
if($config['judges_specialaward_only_enable'] == 'yes') {
|
|
|
|
<tr><td colspan="2"><hr /></td></tr>
|
|
<tr><td><?=i18n("I am a judge for a specific special award")?>:</td>
|
|
<td><table><tr><td>
|
|
|
|
$ch = ($u['special_award_only'] == 'yes') ? 'checked="checked"' : '';
|
|
echo "<input $ch type=\"checkbox\" name=\"special_award_only\" value=\"yes\" />";
|
|
echo "</td><td>";
|
|
echo i18n("Check this box if you are supposed to judge a specific special award, and please select that award on the Special Award Preferences page.");
|
|
|
|
</td></tr></table>
|
|
</td></tr>
|
|
|
|
}
|
|
*/
|
|
?>
|