forked from science-ation/science-ation
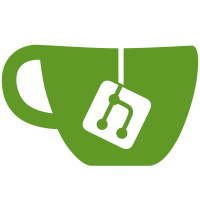
Add min/max judges/volunteers/teams Add defaults Update science olympic tab display Add Teams/Volunteers/Judges status to admin schedule view
430 lines
13 KiB
PHP
430 lines
13 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2010 Youth Science Ontario <info@scitechontario.org>
|
|
Copyright (C) 2010 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
require("../common.inc.php");
|
|
require_once("../user.inc.php");
|
|
require_once("../schedule.inc.php");
|
|
|
|
user_auth_required('admin');
|
|
|
|
if($_GET['action']=="loadschedule") {
|
|
$date=$_POST['date'];
|
|
$starthour=$_POST['starthour'];
|
|
$endhour=$_POST['endhour'];
|
|
|
|
//do some sanity checks
|
|
if($starthour<0 || $starthour>24) $starthour=8;
|
|
if($endhour<$starthour)
|
|
$endhour=$starthour+10;
|
|
if($endhour<0 || $endhour>24) $endhour=15;
|
|
|
|
//minute increment
|
|
$increment=15;
|
|
|
|
if(!eregi("[0-9]{4}-[0-9]{2}-[0-9]{2}",$date)) {
|
|
echo "Invalid date";
|
|
exit;
|
|
}
|
|
echo "<h3>".i18n("Schedule for %1",array(format_date($date)))."</h3>";
|
|
$q=mysql_query("SELECT * FROM locations WHERE conferences_id='{$conference['id']}' ORDER BY name");
|
|
while($r=mysql_fetch_object($q)) {
|
|
$locations[$r->id]=$r->name;
|
|
}
|
|
if(!count($locations)) {
|
|
echo error(i18n("There are no locations defined. Please set up your locations first"));
|
|
echo "<a href=\"locations.php\">".i18n("Location Editor")."</a>\n";
|
|
exit;
|
|
}
|
|
|
|
echo "<table class=\"schedule\" id=\"schedule\">\n";
|
|
echo "<tr>";
|
|
echo "<th style=\"width: 50px;\"> </th>";
|
|
foreach($locations AS $id=>$name) {
|
|
echo " <th>$name</th>\n";
|
|
}
|
|
for($h=$starthour;$h<$endhour;$h++) {
|
|
for($m=0;$m<60;$m+=$increment) {
|
|
echo "<tr>";
|
|
echo " <td class=\"scheduletime\">";
|
|
if($m==0) {
|
|
echo format_time("$h:$m");
|
|
}
|
|
echo "</td>";
|
|
foreach($locations AS $id=>$name) {
|
|
echo "<td id=\"{$h}_{$m}_{$id}\" onclick=\"clickTableCell(this)\"><div>";
|
|
echo "</div></td>";
|
|
}
|
|
echo "</tr>";
|
|
}
|
|
}
|
|
echo "</table>\n";
|
|
|
|
$js="var eventdivs=new Array();\n";
|
|
|
|
//now make all our DIV's for the events that are scheduled in the database
|
|
$x=0;
|
|
//they will be moved by javascript after the fact
|
|
$q=mysql_query("SELECT schedule.*, events.name, events.eventtype FROM schedule JOIN events ON schedule.events_id=events.id WHERE schedule.conferences_id='{$conference['id']}' AND date='{$date}'");
|
|
echo mysql_error();
|
|
while($r=mysql_fetch_object($q)) {
|
|
echo "<div class=\"scheduleevent scheduleevent_{$r->eventtype}\" id=\"event_{$r->id}\" onclick=\"editEvent($r->id)\">";
|
|
echo "<div style=\"width: 99%; text-align: right;\"><a href=\"#\" onclick=\"return deleteEvent(event,$r->id)\"><img style=\"border: 0px;\" src=\"".$config['SFIABDIRECTORY']."/images/16/button_cancel.{$config['icon_extension']}\"></a></div>\n";
|
|
echo "<span class=\"scheduleevent_title\">";
|
|
echo $r->title;
|
|
echo "</span>";
|
|
echo "<br />";
|
|
$starttime=strtotime($r->hour.":".$r->minute);
|
|
$endtime=$starttime+$r->duration*60;
|
|
echo format_time($starttime);
|
|
echo " to ";
|
|
echo format_time($endtime);
|
|
echo "<br />";
|
|
|
|
if($r->eventtype=="scienceolympic") {
|
|
|
|
$regteams=getNumRegistrations($r->id);
|
|
$minteams=$r->sominteams;
|
|
$maxteams=$r->somaxteams;
|
|
if($regteams<$minteams || $regteams >$maxteams)
|
|
$cl="class=\"error\"";
|
|
else $cl="";
|
|
echo "<span $cl>";
|
|
echo i18n("Teams")." : ";
|
|
echo i18n("%1 of %2-%3",array($regteams,$minteams,$maxteams));
|
|
echo "</span>";
|
|
echo "<br />";
|
|
|
|
$regjudges=getNumJudges($r->id);
|
|
$minjudges=$r->sominjudges;
|
|
$maxjudges=$r->somaxjudges;
|
|
if($regjudges<$minjudges || $regjudges >$maxjudges)
|
|
$cl="class=\"error\"";
|
|
else $cl="";
|
|
echo "<span $cl>";
|
|
echo i18n("Judges")." : ";
|
|
echo i18n("%1 of %2-%3",array($regjudges,$minjudges,$maxjudges));
|
|
echo "</span>";
|
|
echo "<br />";
|
|
|
|
$regvolunteers=getNumVolunteers($r->id);
|
|
$minvolunteers=$r->sominvolunteers;
|
|
$maxvolunteers=$r->somaxvolunteers;
|
|
if($regvolunteers<$minvolunteers || $regvolunteers>$maxvolunteers)
|
|
$cl="class=\"error\"";
|
|
else $cl="";
|
|
echo "<span $cl>";
|
|
echo i18n("Volunteers")." : ";
|
|
echo i18n("%1 of %2-%3",array($regvolunteers,$minvolunteers,$maxvolunteers));
|
|
echo "</span>";
|
|
}
|
|
|
|
echo "</div>";
|
|
$js.="eventdivs[$r->id]={hour:$r->hour,minute:$r->minute,location:$r->locations_id,duration:$r->duration};\n";
|
|
$x++;
|
|
}
|
|
$js.="\n\nvar eventdefaults=new Array();\n";
|
|
$q=mysql_query("SELECT events.* FROM events WHERE conferences_id='{$conference['id']}'");
|
|
while($r=mysql_fetch_array($q)) {
|
|
$js.="eventdefaults[{$r['id']}]=".json_encode($r)."\n";
|
|
}
|
|
|
|
echo "<script type=\"text/javascript\">\n";
|
|
echo $js;
|
|
echo "</script>";
|
|
}
|
|
else if($_GET['action']=="loadevent") {
|
|
$id=intval($_GET['id']);
|
|
$q=mysql_query("SELECT schedule.*, events.name, events.eventtype FROM schedule, events WHERE schedule.id='$id' AND schedule.conferences_id='{$conference['id']}' AND schedule.events_id=events.id");
|
|
echo mysql_error();
|
|
if($r=mysql_fetch_assoc($q)) {
|
|
$r['idtype']=$r['events_id'].":".$r['eventtype'];
|
|
$teams=array();
|
|
$teamq=mysql_query("SELECT so_teams.id,
|
|
so_teams.name AS teamname,
|
|
schools.school AS schoolname
|
|
FROM
|
|
so_teams
|
|
JOIN schedule_registrations ON so_teams.id=schedule_registrations.so_teams_id
|
|
JOIN schools ON so_teams.schools_id=schools.id
|
|
WHERE
|
|
schedule_registrations.conferences_id='{$conference['id']}'
|
|
AND so_teams.conferences_id='{$conference['id']}'
|
|
AND schedule_registrations.schedule_id='$id'");
|
|
echo mysql_error();
|
|
while($teamr=mysql_fetch_assoc($teamq)) {
|
|
$teams[]=$teamr;
|
|
}
|
|
$r['teams']=$teams;
|
|
echo json_encode($r);
|
|
}
|
|
else
|
|
echo json_encode(array("id"=>0));
|
|
exit;
|
|
}
|
|
else if($_GET['action']=="saveevent") {
|
|
$id=intval($_POST['id']);
|
|
list($event_id,$event_type)=explode(":",$_POST['event_id']);
|
|
if($event_id>0) {
|
|
if(!$id) {
|
|
mysql_query("INSERT INTO schedule (conferences_id) VALUES ('{$conference['id']}')");
|
|
$id=mysql_insert_id();
|
|
}
|
|
mysql_query("UPDATE schedule SET
|
|
title='".mysql_real_escape_string($_POST['title'])."',
|
|
date='".mysql_real_escape_string($_POST['date'])."',
|
|
hour='".mysql_real_escape_string($_POST['hour'])."',
|
|
minute='".mysql_real_escape_string($_POST['minute'])."',
|
|
duration='".mysql_real_escape_string($_POST['duration'])."',
|
|
events_id='$event_id',
|
|
locations_id='".mysql_real_escape_string($_POST['location_id'])."',
|
|
sominteams='".mysql_real_escape_string($_POST['sominteams'])."',
|
|
somaxteams='".mysql_real_escape_string($_POST['somaxteams'])."',
|
|
sominjudges='".mysql_real_escape_string($_POST['sominjudges'])."',
|
|
somaxjudges='".mysql_real_escape_string($_POST['somaxjudges'])."',
|
|
sominvolunteers='".mysql_real_escape_string($_POST['sominvolunteers'])."',
|
|
somaxvolunteers='".mysql_real_escape_string($_POST['somaxvolunteers'])."',
|
|
sominteamsize='".mysql_real_escape_string($_POST['sominteamsize'])."',
|
|
somaxteamsize='".mysql_real_escape_string($_POST['somaxteamsize'])."'
|
|
WHERE id='$id' AND conferences_id='{$conference['id']}'");
|
|
echo mysql_error();
|
|
happy_("Event successfully saved");
|
|
} else {
|
|
error_("Error saving event - You must select an event");
|
|
}
|
|
exit;
|
|
}
|
|
else if($_GET['action']=="deleteevent") {
|
|
$id=intval($_POST['id']);
|
|
$q=mysql_query("SELECT * FROM schedule_registrations WHERE schedule_id='{$id}' AND conferences_id='{$conference['id']}'");
|
|
if(mysql_num_rows($q)) {
|
|
error_("Cannot remove an event that has teams registered for it. Remove the teams first");
|
|
}
|
|
else {
|
|
mysql_query("DELETE FROM schedule WHERE conferences_id='{$conference['id']}' AND id='{$id}'");
|
|
happy_("Event successfully removed from the schedule");
|
|
}
|
|
exit;
|
|
}
|
|
else {
|
|
|
|
send_header("Schedule Management",
|
|
array('Committee Main' => 'committee_main.php',
|
|
'Administration' => 'admin/index.php',
|
|
'Events & Scheduling' => 'admin/eventsscheduling.php'),
|
|
"events_scheduling" );
|
|
echo "<br />";
|
|
?>
|
|
<script type="text/javascript">
|
|
$(document).ready(function() {
|
|
$(".date").datepicker({ dateFormat: 'yy-mm-dd' });
|
|
changeDate();
|
|
|
|
/* Setup the editor dialog */
|
|
$("#event_editor_dialog").dialog({
|
|
bgiframe: true, autoOpen: false,
|
|
modal: true, resizable: false,
|
|
draggable: false,
|
|
buttons: {
|
|
"<?=i18n('Cancel')?>": function() {
|
|
$(this).dialog("close");
|
|
},
|
|
"<?=i18n('Save')?>": function() {
|
|
saveEvent();
|
|
$(this).dialog("close");
|
|
}
|
|
}
|
|
});
|
|
$(window).resize(function() {
|
|
placeEvents();
|
|
}
|
|
);
|
|
});
|
|
|
|
function changeDate() {
|
|
$("#schedulediv").load("schedule.php?action=loadschedule",{date:$("#date").val(),starthour:$("#starthour").val(),endhour:$("#endhour").val()},function() {
|
|
placeEvents();
|
|
});
|
|
}
|
|
|
|
function clickTableCell(t) {
|
|
var p=$("#"+t.id).offset();
|
|
editEvent(null,t.id);
|
|
|
|
|
|
}
|
|
|
|
function placeEvents() {
|
|
$('.scheduleevent').each(function(idx,item) {
|
|
var eventid=item.id.substr(6);
|
|
var eventobj=eventdivs[eventid];
|
|
|
|
var tablecellid=eventobj.hour+'_'+eventobj.minute+'_'+eventobj.location;
|
|
|
|
if($("#"+tablecellid).length) {
|
|
var eheight=((eventobj.duration/15)*<?=$ROWHEIGHT?>)-<? echo $BORDERSIZE*2; ?>;
|
|
var ewidth=$("#"+tablecellid).width()-<? echo $BORDERSIZE*2; ?>;
|
|
var p=$("#"+tablecellid).offset();
|
|
$("#"+item.id).css(p);
|
|
$("#"+item.id).show();
|
|
$("#"+item.id).height(eheight);
|
|
$("#"+item.id).width(ewidth);
|
|
}
|
|
else {
|
|
$("#"+item.id).hide();
|
|
}
|
|
}
|
|
);
|
|
}
|
|
|
|
function editEvent(id,cell) {
|
|
if(id) {
|
|
var eventobj=eventdivs[id];
|
|
$("#event_editor_dialog").dialog('option','title','Edit Event');
|
|
|
|
$.getJSON("schedule.php?action=loadevent&id="+id,function(json) {
|
|
$("#edit_event").val(json.idtype);
|
|
event_change();
|
|
|
|
//general tab
|
|
$("#edit_schedule_id").val(json.id);
|
|
$("#edit_title").val(json.title);
|
|
$("#edit_date").val(json.date);
|
|
$("#edit_hour").val(json.hour);
|
|
$("#edit_minute").val(json.minute);
|
|
$("#edit_duration").val(json.duration);
|
|
$("#edit_location").val(json.locations_id);
|
|
|
|
//we do this here to set the tabs properly, becuase it also sets the defaults, then we'll override the defaults wth
|
|
//teh real values below
|
|
|
|
if(json.eventtype=="scienceolympic") {
|
|
//science olympics tab
|
|
$("#edit_sominteams").val(json.sominteams);
|
|
$("#edit_somaxteams").val(json.somaxteams);
|
|
|
|
$("#edit_sominjudges").val(json.sominjudges);
|
|
$("#edit_somaxjudges").val(json.somaxjudges);
|
|
|
|
$("#edit_sominvolunteers").val(json.sominvolunteers);
|
|
$("#edit_somaxvolunteers").val(json.somaxvolunteers);
|
|
|
|
$("#edit_sominteamsize").val(json.sominteamsize);
|
|
$("#edit_somaxteamsize").val(json.somaxteamsize);
|
|
|
|
var s='<table class="summarytable"><tr><th><?=i18n("Team Name")?></th><th><?=i18n("School Name")?></th></tr>';
|
|
|
|
for(var i=0;i<json.teams.length;i++) {
|
|
var t=json.teams[i];
|
|
s+='<tr><td>'+t.teamname+'</td><td>'+t.schoolname+'</td></tr>';
|
|
}
|
|
s+='</table>';
|
|
$("#event_editor_tab_scienceolympics_registeredteams").html(s);
|
|
}
|
|
|
|
$("#schedule_tabs").tabs('select',0);
|
|
});
|
|
}
|
|
else {
|
|
$("#event_editor_dialog").dialog('option','title','Create Event');
|
|
var a=cell.split("_");
|
|
$("#edit_event").val("");
|
|
event_change();
|
|
$("#edit_schedule_id").val(0);
|
|
$("#edit_title").val("");
|
|
$("#edit_date").val($("#date").val());
|
|
$("#edit_hour").val(a[0]);
|
|
$("#edit_minute").val(a[1]);
|
|
$("#edit_duration").val(60);
|
|
$("#edit_location").val(a[2]);
|
|
|
|
//science olympics tab
|
|
$("#edit_somaxteams").val("");
|
|
$("#edit_sominteams").val("");
|
|
|
|
$("#edit_somaxjudges").val("");
|
|
$("#edit_sominjudges").val("");
|
|
|
|
$("#edit_somaxvolunteers").val("");
|
|
$("#edit_sominvolunteers").val("");
|
|
|
|
$("#edit_sominteamsize").val("");
|
|
$("#edit_somaxteamsize").val("");
|
|
|
|
$("#schedule_tabs").tabs('select',0);
|
|
|
|
$("#event_editor_tab_scienceolympics_registeredteams").html("");
|
|
|
|
}
|
|
$("#event_editor_dialog").dialog('option','width',600);
|
|
$("#event_editor_dialog").dialog('option','height',400);
|
|
$("#event_editor_dialog").dialog('open');
|
|
|
|
}
|
|
|
|
function saveEvent() {
|
|
var o=$("#edit_event_form").serializeArray();
|
|
//alert(o);
|
|
$("#debug").load("schedule.php?action=saveevent",o,function() {
|
|
changeDate();
|
|
});
|
|
}
|
|
function deleteEvent(event,id) {
|
|
event.stopPropagation();
|
|
|
|
if(confirmClick('Are you sure you want to remove this event from the schedule?')) {
|
|
$("#debug").load('schedule.php?action=deleteevent',{id:id},function() {
|
|
changeDate();
|
|
});
|
|
}
|
|
return false;
|
|
}
|
|
</script>
|
|
|
|
<?
|
|
if(!$editdate) {
|
|
list($editdate,$bla)=explode(" ",$config['dates']['fairdate']);
|
|
}
|
|
?>
|
|
<form method="post">
|
|
<? echo i18n("Schedule date"); ?>
|
|
<input id="date" class="date" type="text" name="date" value="<?=$editdate?>" size="15" onchange="changeDate()">
|
|
<? echo i18n("Start hour")." ";
|
|
emit_hour_selector("starthour",8,"id=\"starthour\" onchange=\"changeDate()\"");
|
|
echo i18n("End hour")." ";
|
|
emit_hour_selector("endhour",15,"id=\"endhour\" onchange=\"changeDate()\"");
|
|
?>
|
|
</form>
|
|
<hr />
|
|
<div id="schedulediv">
|
|
</div>
|
|
<div id="event_editor_dialog">
|
|
<? include "schedule_edit_dialog.php"; ?>
|
|
</div>
|
|
<?
|
|
|
|
send_footer();
|
|
|
|
}
|
|
?>
|