forked from science-ation/science-ation
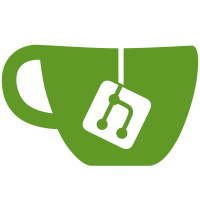
Make queries for communications easier, all you need is a users.id queried, the system will find everything else for you Add ability to use [PASSWORD], [USERNAME], [EMAIL] (tries accounts.email first, if its not there, it uses accounts.pendingemail), in _ANY_ email. [REGNUM] also added but will obviously only work for participants Add "all" section to the tabs list for user editor, so a user without any roles can still get the basic pages like "account", "roles", and "personal info" Put count on participant invitations for teachers (and superusers) Fix a bug where changing a password for a different user didnt work (it changed YOURS!)
145 lines
4.0 KiB
PHP
145 lines
4.0 KiB
PHP
<?
|
|
/*
|
|
This file is part of the 'Science Fair In A Box' project
|
|
SFIAB Website: http://www.sfiab.ca
|
|
|
|
Copyright (C) 2005 Sci-Tech Ontario Inc <info@scitechontario.org>
|
|
Copyright (C) 2005 James Grant <james@lightbox.org>
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public
|
|
License as published by the Free Software Foundation, version 2.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; see the file COPYING. If not, write to
|
|
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
Boston, MA 02111-1307, USA.
|
|
*/
|
|
?>
|
|
<?
|
|
if($_GET['show_types'])
|
|
$NAV_IDENT=$_GET['show_types'][0];
|
|
if($_POST['show_types'])
|
|
$NAV_IDENT=$_POST['show_types'][0];
|
|
|
|
require_once('../common.inc.php');
|
|
require_once('../user.inc.php');
|
|
require_once('../judge.inc.php');
|
|
user_auth_required('admin');
|
|
|
|
require_once('judges.inc.php');
|
|
|
|
if($_GET['action']=="join" && $_GET['accounts_id']) {
|
|
//we're making this user join this conference
|
|
echo "joining {$_GET['accounts_id']} with {$conference['id']}";
|
|
$u = user_create(intval($_GET['accounts_id']), $conference['id']);
|
|
echo happy(i18n("User joined conference"));
|
|
}
|
|
|
|
send_header("Account List",
|
|
array('Committee Main' => 'committee_main.php',
|
|
'Administration' => 'admin/index.php')
|
|
);
|
|
|
|
$querystr = "SELECT * FROM accounts ORDER BY username";
|
|
/*
|
|
echo $querystr;
|
|
echo "<br />\n";
|
|
echo "<br />\n";
|
|
*/
|
|
$q = mysql_query($querystr);
|
|
echo mysql_error();
|
|
echo "<br />\n";
|
|
$num = mysql_num_rows($q);
|
|
echo i18n("Listing %1 account total.",array($num));
|
|
|
|
echo mysql_error();
|
|
echo "<table class=\"tableview\">";
|
|
echo "<thead>";
|
|
echo "<tr>";
|
|
echo " <th>".i18n("Account ID")."</th>";
|
|
echo " <th>".i18n("Username")."</th>";
|
|
echo " <th>".i18n("Email Address")."</th>";
|
|
echo " <th>".i18n("Pending Email")."</th>";
|
|
echo " <th>".i18n("User Info")."</th>";
|
|
// echo " <th>".i18n("Actions")."</th>";
|
|
echo "</tr>";
|
|
echo "</thead>";
|
|
echo "<tbody>";
|
|
|
|
$tally = array();
|
|
$tally['active'] = array();
|
|
$tally['inactive'] = array();
|
|
$tally['active']['complete'] = 0;
|
|
$tally['active']['incomplete'] = 0;
|
|
$tally['active']['na'] = 0;
|
|
$tally['inactive']['complete'] = 0;
|
|
$tally['inactive']['incomplete'] = 0;
|
|
$tally['inactive']['na'] = 0;
|
|
while($r=mysql_fetch_assoc($q)) {
|
|
// get the role data for this user
|
|
echo "<tr>";
|
|
echo "<td>";
|
|
echo $r['id'];
|
|
echo "</td>";
|
|
|
|
echo "<td>";
|
|
echo $r['username'];
|
|
echo "</td>";
|
|
|
|
echo "<td>";
|
|
echo $r['email'];
|
|
echo "</td><td>";
|
|
echo $r['pendingemail'];
|
|
echo "</td>";
|
|
echo "<td>";
|
|
$u=user_load_by_accounts_id($r['id']);
|
|
if($u) {
|
|
//we can edit them even if they dont have any roles, duh
|
|
echo "<b>";
|
|
echo "<a href=\"#\" onclick=\"return openeditor({$u['id']})\">";
|
|
if($u['firstname'] || $u['lastname']) {
|
|
echo $u['firstname']." ".$u['lastname'];
|
|
}
|
|
else {
|
|
echo i18n("No name specified");
|
|
}
|
|
echo "</a>";
|
|
echo "</b>";
|
|
echo "<br />";
|
|
|
|
if(count($u['roles'])) {
|
|
echo "<table>";
|
|
foreach($u['roles'] AS $r=>$rd) {
|
|
echo "<tr><td>";
|
|
echo $rd['name'];
|
|
echo "</td>";
|
|
if($rd['active']=="yes"){ $cl="happy"; $cls=""; } else { $cl="error"; $cls="not "; }
|
|
echo "<td class=\"$cl\">{$cls}active</td>";
|
|
if($rd['complete']=="yes"){ $cl="happy"; $cls=""; } else { $cl="error"; $cls="not "; }
|
|
echo "<td class=\"$cl\">{$cls}complete</td>";
|
|
echo "</tr>";
|
|
}
|
|
echo "</table>";
|
|
} else {
|
|
echo "no roles";
|
|
}
|
|
}
|
|
else {
|
|
echo "no user record for this conference. ";
|
|
echo " <a href=\"account_list.php?action=join&accounts_id={$r['id']}\">click to join conference</a>";
|
|
}
|
|
echo "</td>";
|
|
echo "</tr>";
|
|
}
|
|
echo "</tbody>";
|
|
echo "</table>";
|
|
|
|
send_footer();
|
|
?>
|